How to to Store Phone Numbers in Django
-
Store Phone Numbers Using a Third-Party Django App’s
PhoneNumberField
- Store Phone Numbers Using a Validator and a Django Model
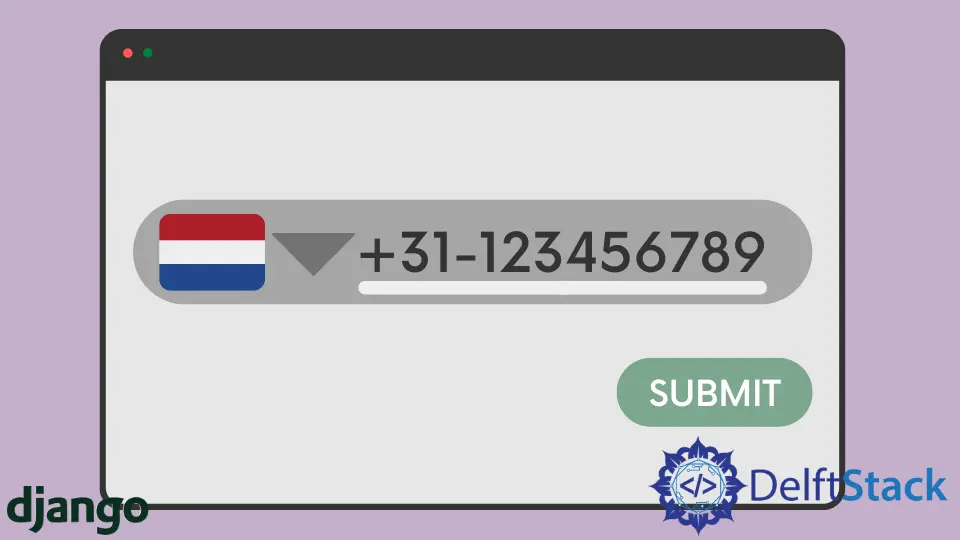
When creating a database, we often run into issues where we can’t decide the field type for specific data. Mostly, we get confused about organizing some field types. For example: if we have to store a unique number, we have to choose between a Character Field or an Integer Field. Each field type has its own advantages and disadvantages.
One such data is a phone number; when storing a phone number, we have to make sure that the field can keep phone numbers from anywhere in the world. We have to ensure that we hold the country code along with the phone number itself.
This article talks about some of the best ways to store a phone number in Django.
Store Phone Numbers Using a Third-Party Django App’s PhoneNumberField
To store phone numbers, we can use a third-party Django App or Library that implements this field: PhoneNumberField
.
You can find the GitHub repository of this library or application here.
According to the official README
, this Django library can validate and convert phone numbers. This library interfaces another Python library, python-phonenumbers, which is a port of Google’s libphonenumber library that powers the Android’s phone number handling.
Install the Django Library
This Django library can be downloaded using the following pip
command:
pip install django-phonenumber-field[phonenumbers]
Since it’s an extensive library, in terms of size, you might want to download the lighter version. To download the lighter version of this library, use the command below:
pip install django-phonenumber-field[phonenumberslite]
Set up the Django Library
To use this app or library, we have to add it inside our project’s settings.py
file. The app name has to be entered inside the INSTALLED_APPS
as follows:
INSTALLED_APPS = [
# Other apps
"phonenumber_field",
]
Use the Django Library
This library has a model field, PhoneNumberField
, which can be used to store phone numbers. Our model will look something like the one below:
from phonenumber_field.modelfields import PhoneNumberField
class Person(models.Model):
firstName = models.CharField(max_length=100)
middleName = models.CharField(max_length=100)
lastName = models.CharField(max_length=100)
phoneNumber = PhoneNumberField(unique=True, null=False, blank=False) # Here
secondPhoneNumber = PhoneNumberField(null=True, blank=True) # Here
The PhoneNumberField
field is internally based on a CharField
space and stores numbers in the form of a string, based on the international phone number standards.
To learn more about this library, refer to the official documentation.
Now, to access this field’s values, we’ll write a Python statement that goes something like this:
person = models.Person.objects.get(id=25)
phoneNumber = person.phoneNumber.as_e164
Here, the phone number is returned as a string in an E.164
standard because of as_e164
. The E.164
is an international standard to store phone numbers. You can read more about it here.
Store Phone Numbers Using a Validator and a Django Model
If you don’t want to use a third-party Django app and store phone numbers using Django models, you can use a Validator
and a CharField
. The following code depicts the same:
from django.core.validators import RegexValidator
class Person(models.Model):
phoneNumberRegex = RegexValidator(regex=r"^\+?1?\d{8,15}$")
phoneNumber = models.CharField(
validators=[phoneNumberRegex], max_length=16, unique=True
)
The phoneNumberRegex
validator validates the value entered for the CharField
. Again, the phone numbers are stored in an E.164
format.
The CharField
has a max length of 16 characters because the E.164
standard allows a maximum of 15 digits for a number. Fifteen characters include both the country code and the phone number. An additional character is reserved for the + sign, which is the prefix for the country code.