How to Upload Media in Django
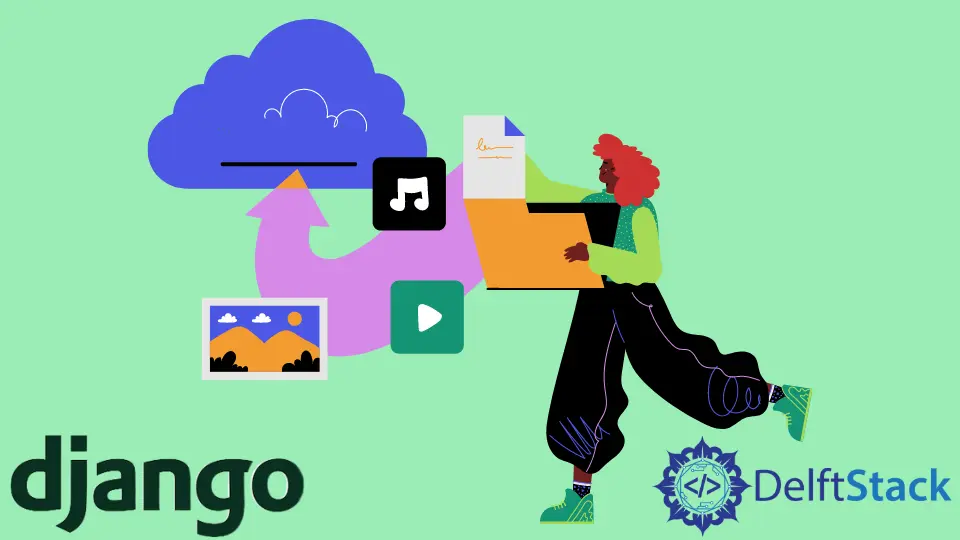
In this demo article, we will have a look at a short introduction about the media files and also have a look at how we can operate media files in the Django project.
Upload Media Using MEDIA_ROOT
and MEDIA_URL
in Django
Django, by default, has a standard way of dealing with files in the local file system.
If you ever looked through your settings in the Django project, you have probably seen MEDIA_ROOT
and MEDIA_URL
, and in another project, you have probably seen where you have access to files on the MEDIA_URL
.
So now we will cover how to set it up in your Django project.
For example, we have the Demo project, and inside it, we have a document app. If we look at the model inside, we will see the document model with a title
and a file
field.
In the file
field, we have the upload_to
keyword argument equal to documents which means wherever files are uploaded. We want to put all files for this object inside a subfolder of documents.
Code:
from django.db import models
class Document(models.Model):
title = models.CharField(max_length=128, blank=True)
file = models.FileField(upload_to="documents", max_length=200)
So, the first thing we need to start our configuration is to open up our settings.pys
.
If you are top of your settings, you will see a based or constant. This gets to the root of our project and allows us to set up a based path.
Code:
BASE_DIR = Path(__file__).resolve().parent.parent
If we jump to the bottom of our setting.py
file, we will start with a MEDIA_ROOT
and set os.path.join
. Then we will join BASE_DIR
as a media, which means we will define the media folder in our project, where we will upload all of our files.
Next, we will set our MEDIA_URL
, so Django can properly build our URLs. This also means that media will be the first thing we see at the end of our domain name for accessing user-uploaded content.
Code:
# Manage media
import os
MEDIA_ROOT = os.path.join(BASE_DIR, "media")
MEDIA_URL = "/media/"
The next thing that we need to set up is our URL routing to properly view these on our local machine with the help of the urls.py
file. So, we need to open up our urls.py
, call a static()
method, and use our settings for MEDIA_URL
and MEDIA_ROOT
.
We will need a static file; then, the urls.py
file will know where to build a proper URL to get locally.
The static
method generates the URLs correctly. We need to import our setting because the interesting thing about this static
method is that it will only work locally, not in production.
Meaning that when you have a debug set to False
in production, this will not work. You need to properly configure the webserver you are using to serve your media folder properly.
Code:
from django.contrib import admin
from django.urls import path, include
from django.conf import settings
from django.conf.urls.static import static
urlpatterns = [
path("admin/", admin.site.urls),
] + static(settings.MEDIA_URL, document_root=settings.MEDIA_ROOT)
The last thing we need to do is create a media folder at the root of our project and take care of that by creating a documents folder inside our media folder.
If we go to our Django admin panel and try to upload a new image, then we can upload a file. After uploading, when you look at this image in your documents folder, you will see it properly uploaded.
We now have a good introduction to configuring the media URL on the media root. When users upload files to your system, the backend knows where to put them and where to pull them.
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn