How to Download File in Django
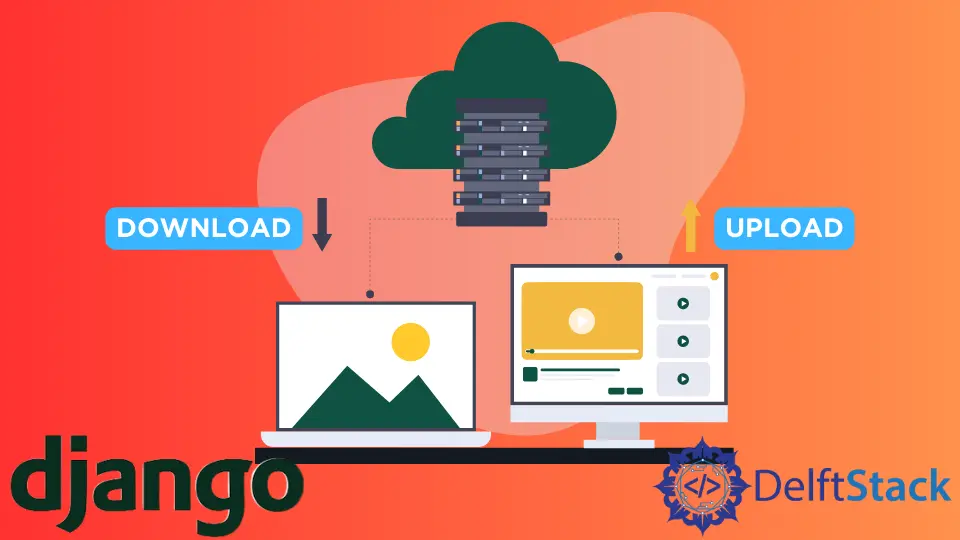
This article shows how to create a website where the admin can upload a file and make the user download a file from a web page in Django.
Upload and Download a File in Django
Let’s go to the terminal and create a demo project to demonstrate to you. Follow the commands to create a Django project and an app in that project.
django-admin startproject django_project
python manage.py startapp blog
Let’s open this newly created Django project in the available text editor.
We will first create a folder for our uploaded files, so let’s open settings.py
. Find the INSTALLED_APPS
list and pass the app name as an item.
INSTALLED_APPS = [
"blog.apps.BlogConfig",
]
Now we need to create a folder where the files will get uploaded. Find the STATIC_URL
variable and write the following code below the variable.
STATICFILES_DIRS = [os.path.join(BASE_DIR, "static")]
Now we need to set our MEDIA_URL
equals to '/media/'
and then set MEDIA_ROOT
, which would be the folder’s name where the uploaded files will be saved.
MEDIA_ROOT = os.path.join(BASE_DIR, "media_cdn")
Now let’s add our media and static rules to the urls.py
. Let’s define the path for home
and download
functions that will pull from the views.py
file.
We are also going to set the URL for the download file.
We cannot use the url
object because this is deprecated. So instead of this, we can easily use the re_path
object.
re_path(r"^download/(?P<path>.*)$", serve, {"document_root": settings.MEDIA_ROOT})
In our case, we are using this code.
Let’s create our model inside the models.py
file, and in this model, we will have two fields. The first field would be a FileField
, and the second would be CharField
.
We also need to create a string dunder method to return the page’s title.
We need to register our FilesAdmin
model in the admin.py
file.
from .models import FilesAdmin
admin.site.register(FilesAdmin)
We will create a home.html
file within the blog templates inside the blog folder. Let’s add the following code in the home.html
file among the body tag.
{% for post in file %}
<h2>{{post.title}}</h2>
<a href="{{post.adminupload.url}}" download="{{post.adminupload.url}}">Download</a>
{% endfor %}
We will get all objects from the FilesAdmin
model in the home
function. Then pass the context in the home.html
file and render this page.
We need to define our home
and download
functions in the views.py
file. We will get the path in the download
function, where the file will download by default.
In the next line, we will check whether the directory exists or not. Then we will open a file in reading binary mode and return it in the HTTP response.
from django.http import HttpResponse, Http404
from django.conf import settings
import os
from django.shortcuts import render
from requests import Response
from .models import FilesAdmin
def home(request):
# get all objects
OUR_CONTEXT = {"file": FilesAdmin.objects.all()}
return render(request, "blog/home.html", OUR_CONTEXT)
def download(request, path):
# get the download path
download_path = os.path.join(settings.MEDIA_ROOT, path)
if os.path.exists(download_path):
with open(download_path, "rb") as fh:
response = HttpResponse(fh.read(), content_type="application/adminupload")
response["Content-Disposition"] = "inline; filename=" + os.path.basename(
download_path
)
return response
raise Http404
Now we need to make migration by running these commands.
python manage.py makemigrations
python manage.py migrate
Create a superuser.
python manage.py createsuperuser
Let’s run the Django server and try to upload an mp3 file from the admin panel.
Let’s open the home page and see if we can download it or not.
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn