How to Change Password in Django
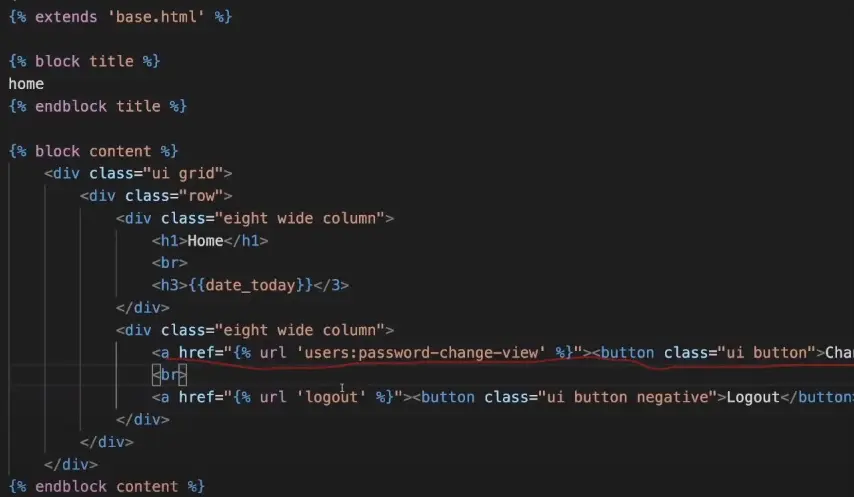
We will learn how to create a webpage for the user to change the password after logging on to the Django webpage.
Create a Webpage for the User to Change the Password in Django
For example, we have a project in Django, and we will be working on this project where we have the users’ application. In this app, we find the views.py
file, and we need to import two necessary views.
One of them is called PasswordChangeView
, which will allow us to change the password, and the PasswordResetDoneView
will confirm that the password has been reset.
Now we need to define a class called MyPasswordChangeView
which will inherit from PasswordChangeView
; inside this class, we declare the template_name
variable and store the template URL where the user will change the password.
In the success_url
variable, we need to put the reverse_lazy()
variable. Below the code, we need to create another class called MyPasswordResetDoneView
and inherit it from PasswordResetDoneView
.
We only declare the template_name
variable inside this class and store the path of the template to this template_name
variable; then, we need to save it and register these views in the urls.py
file.
views.py
file:
from django.shortcuts import render
from django.urls import reverse_lazy
from django.contrib.auth.views import PasswordChangeView, PasswordResetDoneView
class MyPasswordChangeView(PasswordChangeView):
template_name = "users/password-change.html"
success_url = reverse_lazy("users:password-change-done-view")
class MyPasswordResetDoneView(PasswordResetDoneView):
template_name = "users/password-reset-done.html"
We will create a urls.py
file inside the users’ application and then import our views into the file we created.
Then we need to create a variable called app_name
and equal to users
, which is our app name. Below the line, we will create a urlpatterns
list where we will integrate views with URLs.
We will be creating templates, but before moving anywhere, we need to include the users’ path to urlpatterns
of the main project in the urls.py
file. Let’s create a new path below the movies
for the users, and the namespace
should match the app; after writing the code, save it.
Now we can go back to the users’ app, and over here, let’s create a templates
folder; inside the templates
, we will create another folder called users
. In the users
folder, we need to create two HTML files we have defined inside the views.py
file.
We will start working on the password-change.html
file, so first of all, inherit from the base.html
, and after that, we set the block title
and the block content
that will consist of a form. Let’s put in form action
blank, and the method is equal to POST
, which means we are dealing with a POST
request.
We need to put csrf_token
inside the form, which will allow sending requests. We will create a button and keep this type as submit
, making it a green button by adding a class.
We will work on password-reset-done.html
, and over here, we again need to inherit from the base.html
file; after this, the approach will be the same, but the syntax will be a little bit different.
Then we will write a href
attribute and provide the path’s name for the home view, so the user will reach out by pressing this button.
The final thing is to go to the home.html
to create a button to access a page where the user will change the password.
The server is running, and we will go to change the password through the home page, and we can see that after login, the user can change the password.
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn