How to Integrate Ajax With Django in Python
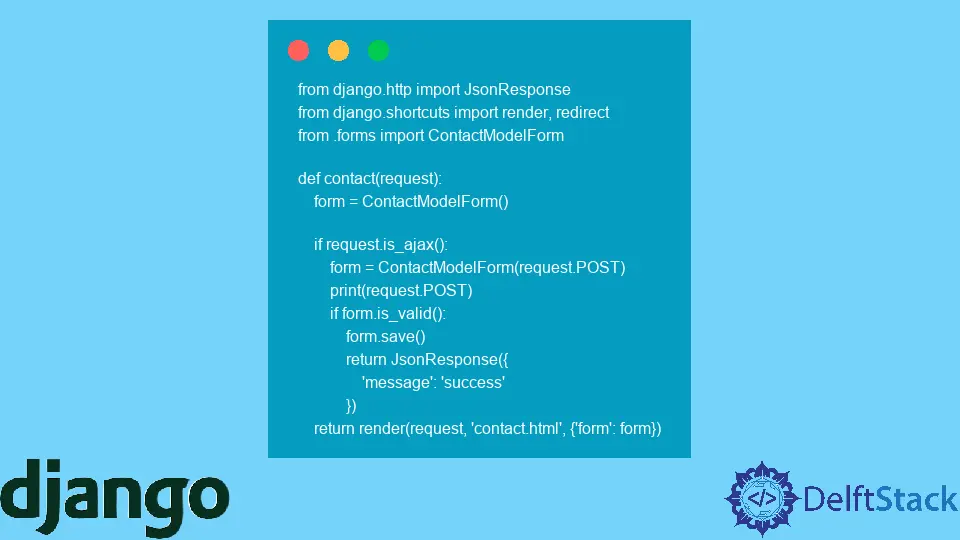
This article aims to demonstrate what Ajax is, integrate Ajax with Django, send Ajax’s get
and post
requests from a browser, and handle the data on the Django site in Python.
Integrate Ajax With Django in Python
Ajax stands for asynchronous JavaScript and XML, but what does that mean? Ajax is merely a web development technique used to send and retrieve data in the background without refreshing a web page.
A real-world Ajax example is if we head over to Twitter and open our feed.
After some time, you will see a menu bar appear at the top of your screen indicating that new tweets are available, and if you wait even longer, you will see that the number increases as more and more people you follow post something on Twitter.
We need to note that our page never refreshes entirely. Now we have some idea of Ajax, so let’s see how to use it with the Django framework.
We created a simple project to demonstrate how to work with Ajax, but it is impossible to demonstrate how to work our entire project. If you are familiar with Django, you already know how to create a Django project, so we have a model named contact
with a few fields and a simple model for the contact model form.
When we submit the form, the data will be saved into the database, redirected to the same page itself, and set those corresponding URLs.
We are using jQuery to trigger Ajax, and CDN is also required. So the logic we will do in the form whenever we submit the form is that if the form is valid, the server will respond.
We are using regular JavaScript to fetch the form’s objects, so we need a submit
event, and if that event is triggered, it will call a submithandler
function.
We create a submithandler
function that will receive the event by default, and we are going to prevent the form submission’s default action, which means the form will not get submitted, so to make an Ajax request, we will write some jQuery in our HTML file.
We will specify some attributes in the Ajax object; the first is type
, which is a type of request.
We can pass two types of requests; get
or post
, so we must pass post
. The next attribute is the url
which means which URL we want to hit the post
request.
So we will use the Django templates index here; we will pass the URL of our contact
page. The contact
is the name of the URL that we will write later in the url.py
.
Now the next one is data
, which means we want the form data to be on the server-side to process form data on the server. So we need to send that data in the Ajax request using form ID.
The next one is the dataType
which is the type of data we expect back from the server, so if we are sending some HTTP response in the views and the form is valid or sending some JSON data, we need to pass data in JSON format, so we pass it in JSON type.
We passed the success
attribute for the last one, which takes a function called successFunction
. This function will raise a pop-up alert window if the request is successful.
<script src="https://cdn.jsdelivr.net/npm/popper.js@1.16.0/dist/umd/popper.min.js"
integrity="sha384-Q6E9RHvbIyZFJoft+2mJbHaEWldlvI9IOYy5n3zV9zzTtmI3UksdQRVvoxMfooAo"
crossorigin="anonymous"></script>
<script>
const form = document.getElementById('form');
form.addEventListener("submit", submitHandler);
function submitHandler(e) {
e.preventDefault();
$.ajax({
type : 'POST', // define the type of HTTP verb we want to use (POST for our form)
url : '{% url 'contact' %}', // the url where we want to POST
data : $('#form').serialize(), // our form data
dataType : 'json', // what type of data do we expect back from the server
success : successFunction
});
}
function successFunction(msg) {
if (msg.message === 'success') {
alert('Success!');
form.reset()
}
}
</script>
Now we need to set the URL with the name contact
and call a contact
method from the views.py
file, which means when the user hits this URL, the contact
method will call.
urlpatterns = [
path("", views.contact, name="contact"),
]
Now we could do something in the views.py
file, so we know that whenever we submit the form, the contact
function will call the corresponding function and catch that, and it will receive an Ajax request and check that the Ajax request is valid or not using .is_ajax()
method.
from django.http import JsonResponse
from django.shortcuts import render, redirect
from .forms import ContactModelForm
def contact(request):
form = ContactModelForm()
if request.is_ajax():
form = ContactModelForm(request.POST)
print(request.POST)
if form.is_valid():
form.save()
return JsonResponse({"message": "success"})
return render(request, "contact.html", {"form": form})
Click here to read the official documentation to integrate Ajax with Django.
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn