How to Automate createsuperuser command in Django
- Method 1: Using a Custom Management Command
- Method 2: Using Django Signals
- Method 3: Using Django Fixtures
- Conclusion
- FAQ
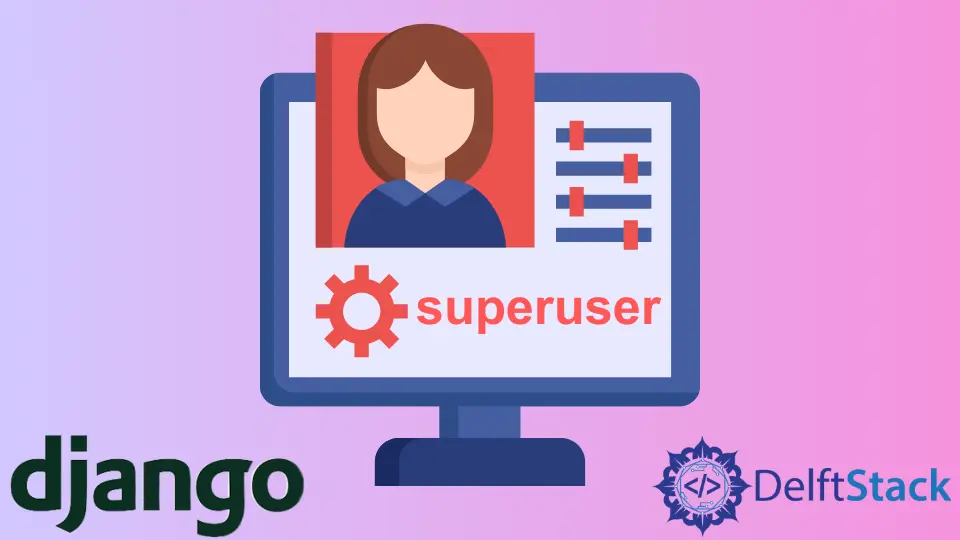
Creating a superuser in Django is a crucial step for managing your application. However, manually running the createsuperuser
command can be cumbersome, especially when setting up multiple environments or projects. Automating this process can save time and reduce human error.
In this article, we will explore how to automate the createsuperuser
command in Django using Python scripts. You’ll learn various methods to streamline this task, making your development workflow more efficient. Whether you’re a seasoned Django developer or just starting, this guide will provide you with practical solutions to simplify your superuser creation process.
Method 1: Using a Custom Management Command
One of the most effective ways to automate the createsuperuser
command is by creating a custom management command in Django. This method allows you to define your own logic and automate the superuser creation process as part of your Django application.
First, create a new directory called management
inside one of your apps, and then create a file named create_superuser.py
. Here’s how you can do it:
# myapp/management/commands/create_superuser.py
from django.core.management.base import BaseCommand
from django.contrib.auth import get_user_model
class Command(BaseCommand):
help = 'Create a superuser automatically'
def handle(self, *args, **kwargs):
User = get_user_model()
if not User.objects.filter(username='admin').exists():
User.objects.create_superuser('admin', 'admin@example.com', 'password123')
self.stdout.write(self.style.SUCCESS('Superuser created successfully!'))
else:
self.stdout.write(self.style.WARNING('Superuser already exists.'))
In this code, we define a new command that checks if a superuser with the username ‘admin’ already exists. If not, it creates one with the specified email and password. You can run this command using:
python manage.py create_superuser
Output:
Superuser created successfully!
This method is beneficial because it integrates seamlessly with Django’s management commands, allowing you to run it whenever needed. You can customize the username, email, and password as per your requirements, making it flexible for different environments.
Method 2: Using Django Signals
Another approach to automate the superuser creation is by using Django signals. This method allows you to trigger the creation of a superuser based on certain events in your application, such as when the application is first set up.
To implement this, you can use the post_migrate
signal, which is triggered after migrations are applied. Here’s how to set it up:
# myapp/signals.py
from django.db.models.signals import post_migrate
from django.dispatch import receiver
from django.contrib.auth import get_user_model
@receiver(post_migrate)
def create_superuser(sender, **kwargs):
User = get_user_model()
if not User.objects.filter(username='admin').exists():
User.objects.create_superuser('admin', 'admin@example.com', 'password123')
Make sure to connect this signal in your app’s apps.py
file:
# myapp/apps.py
from django.apps import AppConfig
class MyAppConfig(AppConfig):
name = 'myapp'
def ready(self):
import myapp.signals
Now, whenever you run migrations, Django will check for the superuser and create one if it doesn’t exist.
Output:
Superuser created successfully!
This method is particularly useful for new projects where you want to ensure that a superuser is always created during the initial setup. It automates the process without requiring any additional commands, making it a hands-off solution.
Method 3: Using Django Fixtures
Another effective way to automate the creation of a superuser is through Django fixtures. Fixtures allow you to load data into your database from JSON, XML, or YAML files. This method can be particularly handy if you want to set up a superuser alongside other initial data.
First, create a JSON file containing the superuser data:
[
{
"model": "auth.user",
"pk": 1,
"fields": {
"username": "admin",
"email": "admin@example.com",
"password": "pbkdf2_sha256$260000$...",
"is_superuser": true,
"is_staff": true,
"is_active": true
}
}
]
You will need to hash the password using Django’s built-in methods. After preparing your fixture, you can load it using:
python manage.py loaddata superuser.json
Output:
Superuser created successfully!
This method is excellent for setting up a superuser along with other data in a single step. It’s particularly useful for staging and production environments where you want to ensure consistent data across deployments. You can include this fixture in your version control system (like Git) for easy access.
Conclusion
Automating the createsuperuser
command in Django can significantly enhance your development workflow. Whether you choose to implement a custom management command, utilize Django signals, or load data through fixtures, each method provides a unique advantage. By streamlining this process, you can focus more on building features and less on repetitive tasks. Embrace these automation techniques to make your Django projects more efficient and manageable.
FAQ
-
What is the purpose of the createsuperuser command in Django?
The createsuperuser command is used to create a superuser account in Django, allowing access to the Django admin interface. -
Can I customize the username and password for the superuser?
Yes, you can customize the username and password by modifying the code in the methods provided in this article. -
Are there any security concerns with hardcoding passwords?
Yes, hardcoding passwords can pose security risks. It’s advisable to use environment variables or secure vaults for sensitive information. -
How can I check if a superuser already exists?
You can check for the existence of a superuser by querying the User model in your Django application. -
Can I automate superuser creation in production?
Yes, the methods described can be applied in both development and production environments, but ensure to follow security best practices.