How to Make a Text Blink Using CSS and JavaScript
- Blink Text in HTML
- Make a Text Blink Using Internal or Embedded CSS
- Make a Text Blink Using In-Line CSS
- Make a Text Blink Using External CSS
- Make a Text Blink Using JavaScript
- Make a Text Blink Using JQuery
- Make a Blinking Text at Multiple Sections of a Page Using JavaScript
- Make an Alternate Blinking Text Using CSS
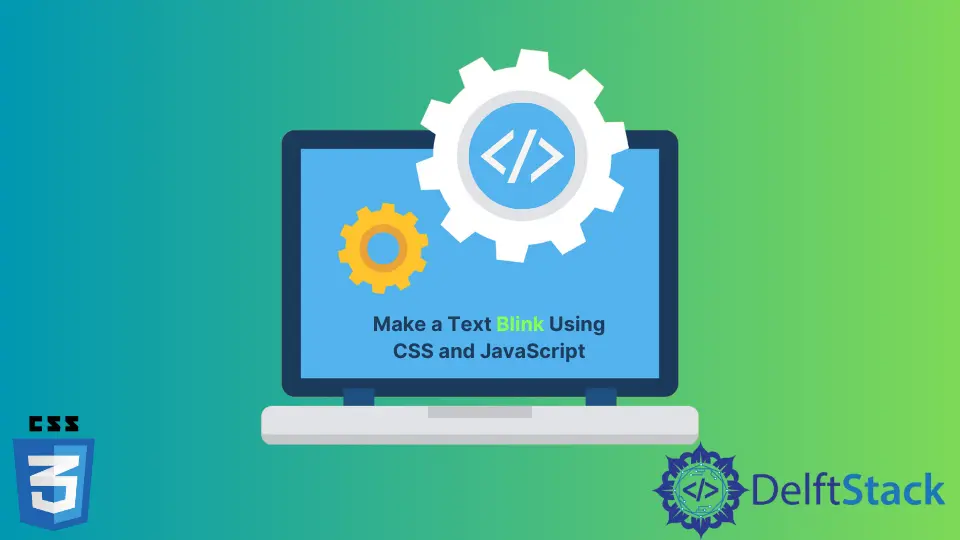
This article will go through the blink/flash text in great depth. We will see its purpose and advantages.
We will learn numerous strategies and techniques to implement blinking text on our website. To implement blink text, we will explore internal CSS, in-line CSS, external CSS, JavaScript, and jQuery.
Blink Text in HTML
Blink Text is the type of text that flashes. On shopping websites, you may see a flashing sale icon or a new collection banner in a flashing style.
It is an effective strategy to get the attention of users. HTML offers the tag <blink>
for this function; however, browsers no longer support it, making it a deprecated tag.
Let’s examine various methods to accomplish this functionality without using the tag.
Make a Text Blink Using Internal or Embedded CSS
Internal CSS is contained within the HTML style
tag. In the tag, we can include any CSS property.
Look at the code below to learn how we used internal CSS to create the blinking text.
<html>
<head>
<style>
.blink {
animation: blinker 1s linear infinite;
}
@keyframes blinker
{
50% {
opacity: 0;
}
}
</style>
</head>
<body>
<div class="blink">Internal CSS Blink !</div>
</body>
</html>
This is one of the most straightforward approaches to making a text blink. We just did a little styling, and the work is done.
Some of the CSS terms are maybe new to you but don’t worry, let us see in detail what each of the terms means.
the animation
Property
We used CSS’s animation
property on the class blink
to apply animations. The animation
property is a shorthand for:
animation-name |
Specify a name for animation. |
animation-duration |
It defines how long an animation should take to complete one cycle. |
animation-timing-function |
Specify the speed curve from the start to the end of an animation. |
animation-delay |
Specify a wait for the start of an animation. |
animation-iteration-count |
Specify the number of times an animation should be played. |
animation-direction |
Specify the direction of animation, i.e., forward, backward, or alternate back and forth. |
animation-fill-mode |
Indicate how CSS animations apply styles to their targets before and after execution. |
animation-play-state |
Specify whether the animation is running or paused. |
Now, let us understand what we wrote in our code. The animation
in the above code holds the following properties:
- The
blinker
is an animation name. - The
1s
is theanimation-duration
, meaning that each cycle will be completed after 1 second. - The
linear
is theanimation-timing-function
, meaning that the animation will play at the same speed from beginning to end. - The
infinite
is theanimation-iteration-count,
which means the animation will be played unlimited times.
To summarize the entire information, we concluded that the animation blinker
would run through each cycle in one second at the same speed, an endless number of times.
Keyframes
In CSS, the @keyframes
define the animation rule. Changeable CSS styles are used to create animations.
Multiple CSS property changes may occur during an animation. We must determine when a change in style occurs in percentages or contains the phrases from
and to
, which are equivalent to 0% and 100%; 0% denotes the start, and 100% denotes its completion.
For the best browser support, always specify the 0% and the 100% selectors. We will see it later in the article.
The CSS syntax of the keyframes is:
@keyframes animation-name {*keyframes-selector* {*css-styles;}*}
Let’s have a look at what we did in our code.
We put keyframes on our animation blinker
and specify that at 50%, its opacity should be zero. An element’s transparency is set through the opacity
property.
The value for the opacity
property must be strictly between 0 to 1.
CSS Vendor Prefixes
A unique prefix added to a CSS property is called a vendor prefix. There are several CSS properties that older browser versions do not support, so you must use a browser prefix to help those tags.
There is a prefix for each rendering engine that will only apply the attribute to that specific browser.
- Internet Explorer, Microsoft Edge:
-ms-
- Chrome, Safari, iOS, Android:
-webkit-
- Firefox:
-moz-
- Opera:
-o-
Let’s rewrite the code described above to work with as many browsers as possible.
<html>
<head>
<style>
.blink {
-webkit-animation: blinker 1s linear infinite;
-moz-animation: blinker 1s linear infinite;
-ms-animation: blinker 1s linear infinite;
-o-animation: blinker 1s linear infinite;
animation: blinker 1s linear infinite;
}
@-webkit-keyframes 'blinker' {
0% {
opacity: 1;
}
50% {
opacity: 0;
}
100% {
opacity: 1;
}
}
@-moz-keyframes 'blinker' {
0% {
opacity: 1;
}
50% {
opacity: 0;
}
100% {
opacity: 1;
}
}
@-o-keyframes 'blinker' {
0% {
opacity: 1;
}
50% {
opacity: 0;
}
100% {
opacity: 1;
}
}
@-ms-keyframes 'blinker' {
0% {
opacity: 1;
}
50% {
opacity: 0;
}
100% {
opacity: 1;
}
}
@keyframes 'blinker' {
0% {
opacity: 1;
}
50% {
opacity: 0;
}
100% {
opacity: 1;
}
}
</style>
</head>
<body>
<div class="blink">Hello Blinking World!</div>
</body>
</html>
Make a Text Blink Using In-Line CSS
In-line CSS allows you to put the styling directly into the HTML elements. Although it is good to know, in-line CSS is not a recommendable option as it is element dependent, so it is not reusable.
Also, we cannot use all the properties and functionality of CSS in the in-line styling. Speaking of our current scenario of blinking text, we cannot use keyframes in in-line CSS as keyframes are not part of the HTML elements.
Let’s see how to flash a text using in-line CSS.
<html>
<head>
<style>
@-webkit-keyframes 'blinker' {
50% {
opacity: 0;
}
}
@-moz-keyframes 'blinker' {
50% {
opacity: 0;
}
}
@-o-keyframes 'blinker' {
50% {
opacity: 0;
}
}
@-ms-keyframes 'blinker' {
50% {
opacity: 0;
}
}
</style>
</head>
<body>
<div class="blink" style="animation: blinker 1s linear infinite">
In-line CSS Blink !
</div>
</body>
</html>
Make a Text Blink Using External CSS
External CSS is the way of adding a CSS file to our HTML file. It is an excellent practice to keep styling and elements separate.
You can add the CSS file by using the link
tag. Make a CSS file with the name style.css
, copy the code in the style
tag from the above code, and add it to the HTML file. Here’s how.
<html>
<head>
<link rel="stylesheet" type="text/css" href="style.css">
</head>
<body>
<div class="blink">Hello Blinking World!</div>
</body>
</html>
Make a Text Blink Using JavaScript
JavaScript has always been a lifesaver when performing almost any functionality. It provides various methods and techniques to do amazing things very quickly.
Let us see how to make a text blink using JavaScript.
<html>
<head>
<script>
var blink_speed = 300;
setInterval(function(){
var element = document.getElementById("blink");
element.style.display = element.style.display == 'none'?'':'none';
}, blink_speed);
</script>
</head>
<body>
<div id="blink">Javascript Blink !</div>
</body>
</html>
Let’s understand the JavaScript code here. What magic is it doing?
First, we initialize a variable with the name blink_speed
and assign the value; you can set any value of your choice. If you give 1000, it means 1 second, 2000 points 2 seconds, and so on.
We assign 300 in our code, meaning it’ll be less than 1 second.
Secondly, we use the setInterval
function of JavaScript. The setInterval
method continues to call the function at specified intervals (in milliseconds).
Here is the syntax of the function:
setInterval(function, milliseconds)
The function
parameter is the instructions to be executed repeatedly at specified intervals. The millisecond
parameter receives the speed for the execution intervals.
In our code, the function
parameter of setInterval
first gets the element by Id name. After getting the required element, we check by using the ternary operator that if the display of our element is none
, then display it and vice versa.
Then we assigned the blink_speed
to the second parameter of the setInterval
function.
Make a Text Blink Using JQuery
jQuery is a library of JavaScript, and its purpose is to make JavaScript as easy as possible. Let’s see how to make blinking text using jQuery.
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script>
function blinkText(element){
$(element).fadeOut('slow', function(){
$(this).fadeIn('slow', function(){
blinkText(this);
});
});
}
$(function() {
blinkText('#blink');
});
</script>
</head>
<body>
<div id="blink">JQuery Blink !</div>
</body>
</html>
This is pretty simple. Let’s see what the code is doing.
As jQuery is a JavaScript library, we need to add its reference in our code to use it. In the code, we created a simple function, blinkText
, which receives an HTML element.
First, we call the fadeOut
function on the element, and then inside this function, we call another function, fadeIn
, on it. Inside fadeIn,
we give a recursive call to our function as we want it to show blinking text infinite times.
In conclusion, the text will fade out, fade in, fade out, fade in, and so on, as it is a never-ending recursive call.
Make a Blinking Text at Multiple Sections of a Page Using JavaScript
Let’s do an exciting task. We want to make blinking text in multiple areas of a page.
It is straightforward to do with CSS. Assign the same class to all elements you want to make blink, and it will be done.
But how to do it with JavaScript? As we saw in the above code, JavaScript selects only one element.
Let’s find out how we can achieve this.
<html>
<head>
<script>
function blinkText()
{
var elements = document.getElementsByClassName("blink");
for(var i = 0, l = elements.length; i < l; i++)
{
var blink = elements[i];
blink.style.display = blink.style.display == 'none' ? '' : 'none';
}
}
setInterval(blinkText, 300);
</script>
</head>
<body>
<div class="blink">Hello</div>
<div class="blink">World</div>
</body>
</html>
If we have many elements with the same class name, the document.getElementByClassname
will return an array of all the elements. We then traverse each element one by one and do the task.
Make an Alternate Blinking Text Using CSS
Now, let’s create another fantastic animation to make an alternate word blinking text using CSS only.
<html>
<head>
<style>
.blink::before {
content: "Welcome";
animation: blinker 1s linear infinite alternate;
}
@keyframes blinker {
0% {
content: "Welcome";
}
50% {
opacity: 0%;
}
100% {
content: "Developer";
}
}
</style>
</head>
<body>
<div class="blink"></div>
</body>
</html>
It will initially display Welcome
, then blink, and then say Developer
. This loop repeats continuously.