How to Read Barcode in C#
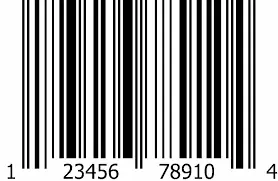
This tutorial explains how to read a barcode from any source. It presents a complete program to create a barcode reader in C# using a third-party package.
Read Barcode in C#
You can write a program to read barcode images in C# using a third-party assembly package, i.e., Aspose.BarCode
. This package can be downloaded in your visual studio project from NuGet.org.
To read the barcodes, you first need to import the Aspose.BarCode.BarCodeRecognition
namespace. Next, create an object of the BarCodeReader
class and use the ReadBarCodes()
method.
The benefit of using the Aspose.BarCode
is to write very few lines of code to accomplish your desired output. Let’s create a C# project to demonstrate the barcode reader in C#.
-
Create a C# console application in Visual Studio.
-
Navigate to the
"Project"
tab and select"Manage NuGet Packages"
. -
Browse for the
Aspose.Barcode
package from NuGet.org and install it.Now, you can import the
Aspose.BarCode.BarCodeRecognition
namespace in any portion of your code to utilize the Barcode scanning functionalities. -
Copy the following code snippet to your
program.cs
fileusing System; using Aspose.BarCode; using System.Drawing; using Aspose.BarCode.BarCodeRecognition; namespace BarcodeScanner { class Program { static void Main(string[] args) { try { // File Exits in the project directory using (BarCodeReader barCodeReader = new BarCodeReader("barcode.png")) { foreach (BarCodeResult output in barCodeReader.ReadBarCodes()) { // Read the barcode Console.WriteLine("Symbology Type: " + output.CodeType); Console.WriteLine("CodeText: " + output.CodeText); } } } catch (Exception ex) { Console.WriteLine(ex.Message); } } } }
This code will read the input image from the project directory. The
foreach
loop in the code reads the output from multiple barcodes in an image.The
BarCodeReader
class has overloaded constructors to support different types of inputs, e.g.,BitmapImage
,Image
,file name
, etc. We have used the constructor that takes the file name as input.
Assume barcode.png
has the following Barcode.
Output:
Note that the Apose Barcode Reader is a paid tool requiring a license to use unrestricted functionalities.