How to Generate Random Numbers in Unity3D
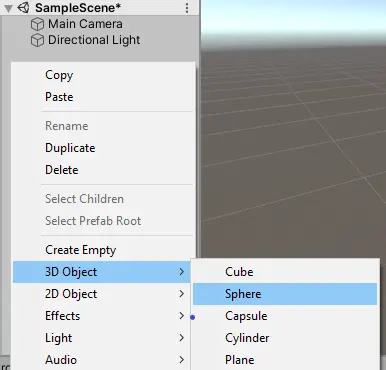
This tutorial will explain the methods to generate random numbers in unity3d. We will implement random number generators with float
and int
return types and look into their execution behaviors with examples.
Random Number Generator in Unity3D
The UnityEngine.Random
provides a built-in method, Range()
, to generate random numbers. Unfortunately, the Range()
method has been overloaded for both int
and float
data types in unity3d.
You can invoke this method by passing float
or int
data types as parameters. The parameters of this function define the range of random numbers by specifying lower and upper bound.
Here is the definition of UnityEngine.Random.Range
function for float
return type:
public static float Range(float minValue, float maxValue);
The Range()
method returns a random float
number within [minValue to maxValue]
. This method swaps the values if maxValue
is less than minValue
.
The float
Range()
method includes lower and upper bounds. For example, Random.Range(1.2f,5.2f)
returns a number between 1.2
and 5.2
, including the extremes.
Here is the definition of UnityEngine.Random.Range
function for int
return type:
public static int Range(int minValue, int maxValue);
This method returns a random integer number within the range [minValue to maxValue]
. The following behaviors are how this method will execute:
- The upper bound is not included in this method. For example,
Random.Range(-5, 5)
returns a value between-5
and4
. - If both
minValue
andmaxValue
are equal, then the upper bound is excluded, and the method returns the lower bound, i.e.,minValue
. - If
maxValue
is less thanminValue
, then the numbers are automatically swapped.
We will implement both of these methods in the Unity3D project. Following is the complete demonstration of implementing these functions. But, first, let’s follow the process step-by-step.
-
Create New Project
Create a new unity project. A sample scene will be created by default.
-
Create 3D Game Object
Create any 3D game object in the scene by right-clicking in the hierarchy window.
-
Create C# Script to Generate
float
Random Number GeneratorCreate a new C# script by right-clicking in the Project window. Then, write the following code snippet to the newly created script.
The following code snippet depicts a
float
random number generator. This code snippet moves thegameObject
in thex-direction
by adding a random number generated within the range of-5.0
and5.0
.using System.Collections; using System.Collections.Generic; using UnityEngine; public class RandomNumberGenerator : MonoBehaviour { public GameObject gameObject; float xOffset = 0.0f; void OnGUI() { xOffset = Random.Range(-5.0f, 5.0f); if (GUI.Button(new Rect(10, 300, 100, 50), "MOVE")) { gameObject.transform.position = new Vector3(gameObject.transform.position.x + xOffset, gameObject.transform.position.y, gameObject.transform.position.z); } } }
-
Attach Script to Game Object
Attach the script to the game object created in the scene. Then, pass the game object to the script by dragging it from the hierarchy.
-
Run Game
Run the game and press the
Move
button in the Game View to change the game object’s position. -
Create Empty Game Object
Create the empty game object in the scene to implement the integer type random number generator.
-
Create New C# Script to Generate
int
Random Number GeneratorCreate a new C# script again and write the following code in the script. This code fence generates a random number between
1
and4
(integer numbers) and prints the descending ordered values, including0
.using System.Collections; using System.Collections.Generic; using UnityEngine; public class IntRNG : MonoBehaviour { void Start() { int x = Random.Range(1, 5); for (int i = x; i >= 0; i--) { print(i); } } }
-
Attach Script to Empty Object
Attach the above script to an empty game object created in the scene.
-
Run Game
Run the game and check the console window to see the output. You can see Unity3D Random Number Generator for further details.