Decimal Literal in C#
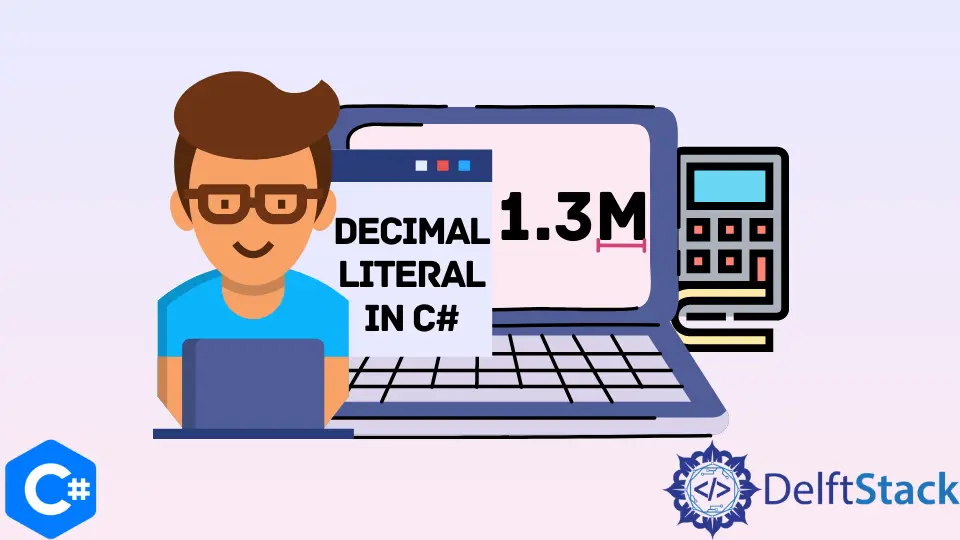
When initializing variables in C#, you may have to specify the data type explicitly you intend it to be for numeric data types. If not, they will be treated as default data types like integers or doubles.
In this section, we’ll explore the importance of the M
or m
suffix in maintaining precision and avoiding inadvertent type conversions.
the Decimal Literal
The decimal
data type serves this purpose, but when working with literal values, the default behavior of the compiler might lead to unintended precision loss. Enter the M
or m
suffix method, a simple yet powerful technique that explicitly indicates to the compiler that a literal should be treated as a decimal
.
If you don’t add the decimal literal M
, the numeric value will be treated as a double and will cause errors. You can use both uppercase and lowercase notation.
Let’s dive into a code example that demonstrates the application of the M
or m
suffix, utilizing the var
keyword for variable declaration, and including comparisons with double
and float
:
using System;
class Program {
static void Main() {
// Representing a monetary value with the M or m suffix
var priceWithSuffix = 49.99M;
// Representing the same value without the suffix (interpreted as double)
var priceWithoutSuffixDouble = 49.99;
// Representing the same value with the F suffix (interpreted as float)
var priceWithSuffixFloat = 49.99F;
// Output the results and data types
Console.WriteLine("Price with M or m suffix: " + priceWithSuffix);
Console.WriteLine("Data type of priceWithSuffix: " + priceWithSuffix.GetType());
Console.WriteLine("Price without suffix (interpreted as double): " + priceWithoutSuffixDouble);
Console.WriteLine("Data type of priceWithoutSuffixDouble: " +
priceWithoutSuffixDouble.GetType());
Console.WriteLine("Price with the F suffix (interpreted as float): " + priceWithSuffixFloat);
Console.WriteLine("Data type of priceWithSuffixFloat: " + priceWithSuffixFloat.GetType());
}
}
In this code snippet, we begin by declaring a variable named priceWithSuffix
and assign a literal value of 49.99M
to it. The M
suffix explicitly indicates that this value should be treated as a decimal
.
The var
keyword allows the compiler to infer the type, ensuring precision in the representation.
Next, we declare two more variables, priceWithoutSuffixDouble
and priceWithSuffixFloat
, and assign the same literal value, 49.99
, to them. These literals are without the M
suffix, and their types are implicitly interpreted as double
and float
, respectively.
Finally, we write Console.WriteLine
statements to output both values and their respective runtime data types using the GetType()
method.
Output:
Price with M or m suffix: 49.99
Data type of priceWithSuffix: System.Decimal
Price without suffix (interpreted as double): 49.99
Data type of priceWithoutSuffixDouble: System.Double
Price with the F suffix (interpreted as float): 49.99
Data type of priceWithSuffixFloat: System.Single
In this example, we’ve explored the application of the M
or m
suffix method in maintaining precision for decimal literals in C#. The output clearly demonstrates that the literal with the suffix is explicitly treated as a decimal
, while the ones without are implicitly interpreted as double
and float
.
The use of var
for variable declaration adds flexibility while ensuring clarity regarding the intended type. The M
or m
suffix method aligns with the principles of clean and expressive C# coding, providing a straightforward way to communicate and preserve precision in decimal literals, especially when compared to other numeric types.
Conclusion
In conclusion, when working with decimal literals in C#, it is crucial to use the M
or m
suffix to explicitly indicate to the compiler that a literal should be treated as a decimal
type. Failure to do so may result in unintended precision loss, as the default behavior of the compiler might interpret the literal as a double
.
The code example provided demonstrates the significance of the M
or m
suffix in maintaining precision, with clear distinctions in data types between decimal
, double
, and float
. Additionally, the use of the var
keyword for variable declaration enhances flexibility while ensuring clarity regarding the intended type.
Overall, adopting the M
or m
suffix aligns with best practices in C# coding, promoting clean and expressive code that accurately represents the developer’s intentions, especially when dealing with monetary values.