Namespace Alias in C#
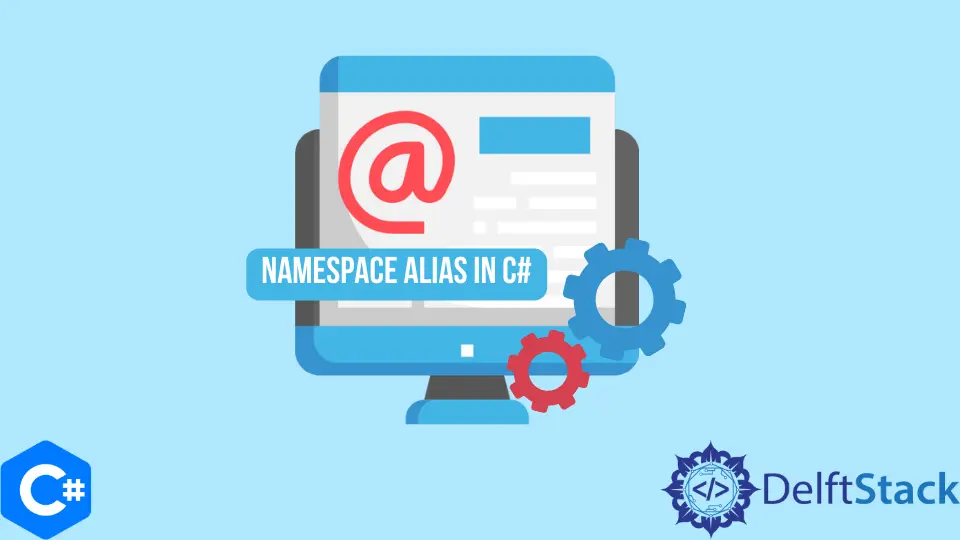
This tutorial will help to understand the concept of namespace alias in C#. We will implement a demo code to help you completely grasp this concept.
Namespace in C#
In C#, a namespace is used to organize the methods, classes, structs, and enums logically to make them efficient and to control the scope of functions and classes.
Type or namespace aliasing is helpful in making code easy to understand and saves time for the developers. Type aliasing is useful when you want to alias a specific class or function from a single namespace.
The using
keyword allows us to access these functionalities in C#.
the using
Directive in C#
The using
compiler directive in C# can be used to define a scope. It can also be used to create an alias for a namespace, import static members of a class, or import specific types from other namespaces.
Console input and output is the basic thing in programming. In C#, the Console
class from the System
namespace is used to perform console operations, i.e., input or output.
You must have used it in C# projects as using System
to access WriteLine()
, ReadLine()
, etc.
Alias in C#
Alias helps to avoid ambiguous statements or classes by replacing long names of namespaces/classes with short ones. It retrains the original logical functionality.
The developer can use an alias to utilize namespace functionality more quickly and effectively. It has no adverse effects on the program run-time; rather, it accelerates the development time.
First, to understand the concept, let’s create a simple C# console project and write the following code that does not use aliases.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace NamespaceAliasing {
class Program {
static void Main(string[] args) {
Console.WriteLine("Hi! I am a Console.WriteLine");
Console.Write("Let me know your good name: ");
var name = Console.ReadLine();
Console.WriteLine("Welcome: " + name);
Console.ReadKey();
}
}
}
If you type user
as input, the output will be as follows.
Hi! I am a Console.WriteLine
Let me know your good name: user
Welcome: user
When you create a console C# project in Visual Studio, it imports some basic namespaces by itself. As you can see, the using System
and other namespaces are imported on top of the source file.
When you import a namespace, the compiler knows that you need to access every member class, method, and variable from that namespace. So, by importing the System
namespace, you can access ReadLine()
, WriteLine()
, Write()
methods.
Now, let’s create an alias for the Console
class so we don’t always need to type it while invoking its associated methods. We created an alias S
to System.Console
in the following code.
Now, we can access the console methods using this alias S
instead of typing Console
.
It is important to note that an alias is just another name for an identifier, so the previous identifier will be functional like it was available earlier.
For example, in the following code, the System.Console
will still be accessible and functional as it was before declaring any alias.
using S = System.Console;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace NamespaceAliasing {
class Program {
static void Main(string[] args) {
S.WriteLine("Hi! I am a S.WriteLine");
S.Write("Let me know your good name: ");
var name = S.ReadLine();
S.WriteLine("Welcome: " + name);
System.Console.WriteLine("I am Console.WriteLine");
S.ReadKey();
}
}
}
Output:
Hi! I am a S.WriteLine
Let me know your good name: user
Welcome: user
I am Console.WriteLine
Another use of an alias is when you have to use multiple classes from different namespaces but have types with the same names.
You can implement this either by using the complete name referencing specific classes or can create aliases for namespaces and access types using aliases.
The following example shows a scenario where two namespaces have methods with the same name.
using S = System.Console;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace NamespaceAliasing {
using Alias1 = NameSpace1.ClassOne;
using Alias2 = NameSpace2.ClassTwo;
class Program {
static void Main(string[] args) {
var object1 = new Alias1();
object1.Sample();
var object2 = new Alias2();
object2.Sample();
S.ReadKey();
}
}
}
namespace NameSpace1 {
public class ClassOne {
public void Sample() {
S.WriteLine("I am a Sample from Namespace1");
}
}
}
namespace NameSpace2 {
class ClassTwo {
public void Sample() {
S.WriteLine("I am a Sample from Namespace2");
}
}
}
Output:
I am a Sample from Namespace1
I am a Sample from Namespace2
The above code snippet has two namespaces named NameSpace1
and NameSpace2
. Both have classes called ClassOne
and ClassTwo,
respectively.
The methods from both namespaces have the same name, so if we call them just by using their name, i.e., Sample()
, the compiler cannot specify from which namespace it’s being called.
We have to specify the complete reference to it by typing Namespace1.ClassOne.Sample()
for correct execution.
It’s challenging and ambiguous if we use it multiple times in our code. This issue can be resolved by creating aliases of namespaces as we created in the above example code.
We don’t need to type Namespace1.ClassOne.Sample
every time; rather, we can use aliases Alias1
and Alias2
.
Aliasing can refer to the static members of a class and use them by their names rather than typing the whole reference. Such as, the Math
class from the System
namespace contains static functions like Sqrt()
and static members like PI
and E
.
Following is an example of calling a static function from another namespace by creating an alias.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using static NameSpace1.SampleClass;
namespace StaticAliasing {
class Program {
static void Main(string[] args) {
function1();
Console.ReadKey();
}
}
}
namespace NameSpace1 {
public class SampleClass {
public static void function1() {
System.Console.WriteLine("I am a static function.");
}
}
}
Output:
I am a static function.
Imagine having to use function1()
multiple times throughout the program. We have to type NameSpace1.SampleClass.function1
every time.
But now we can call it by its name. For more depth, you can see using directive in C#.