The value_type in STL Containers in C++
- Understanding STL Containers
- Accessing Value_Type in STL Containers
- Using Value_Type in Template Functions
- Importance of Value_Type in Memory Management
- Conclusion
- FAQ
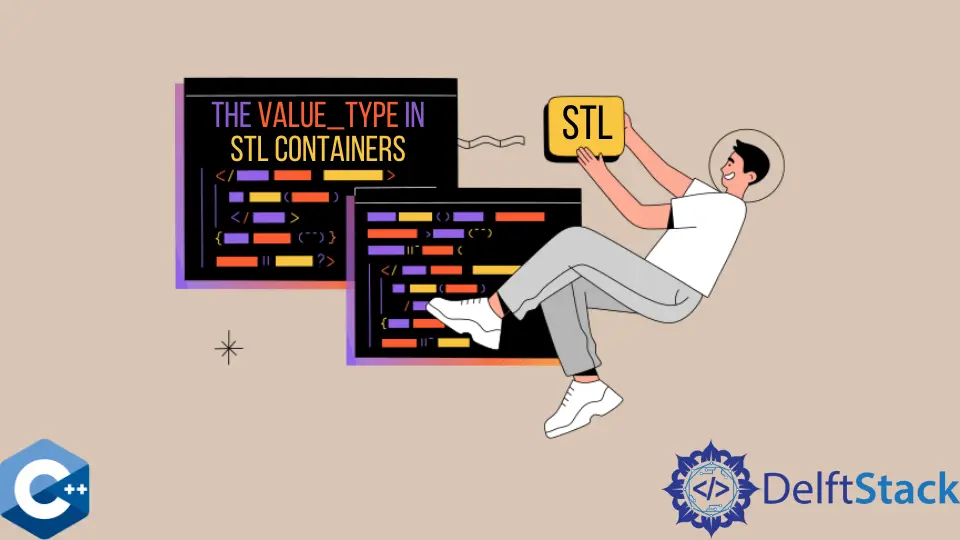
The Standard Template Library (STL) in C++ is a powerful tool that provides a rich set of data structures and algorithms, allowing developers to create efficient and reusable components. One of the key features of STL containers is the value_type
, which defines the type of elements contained within a specific container. Understanding value_type
is essential for effective memory management and type safety in your C++ applications.
This article will delve into the significance of value_type
in STL containers, explore various STL containers, and demonstrate how to utilize value_type
effectively in your code. Whether you’re a seasoned developer or just starting with C++, mastering value_type
will enhance your programming skills and make your code more robust.
Understanding STL Containers
STL containers are designed to store collections of objects. Common types of STL containers include vectors, lists, sets, maps, and queues. Each of these containers has its unique characteristics and use cases, but they all share a common trait: they define a value_type
. The value_type
is a type alias that indicates what kind of data the container holds. This feature is crucial for template programming, enabling developers to write generic code that can work with any data type.
For instance, when you declare a std::vector<int>
, the value_type
is int
. This means that the vector is intended to hold integers. Knowing the value_type
helps in iterating over the container, accessing its elements, and ensuring type safety during operations.
Accessing Value_Type in STL Containers
To access the value_type
of an STL container, you can utilize the ::
operator. This allows you to refer to the value_type
directly from the container’s type. Here’s how you can do it with a simple example using a vector.
#include <iostream>
#include <vector>
int main() {
std::vector<int> myVector;
std::cout << "The value type of myVector is: "
<< typeid(myVector::value_type).name() << std::endl;
return 0;
}
Output:
The value type of myVector is: int
In this example, we create a vector of integers called myVector
. By using typeid(myVector::value_type).name()
, we retrieve the name of the value_type
, which in this case is int
. This approach is particularly useful when working with templates, as it allows you to write functions that can handle various container types without knowing their specific types in advance.
Using Value_Type in Template Functions
One of the most powerful aspects of value_type
is its ability to enhance template programming. By defining functions that accept containers as parameters, you can create reusable code that works with any container type. Here’s an example of a template function that prints the elements of any STL container.
#include <iostream>
#include <vector>
#include <list>
template <typename Container>
void printContainer(const Container& container) {
for (typename Container::value_type element : container) {
std::cout << element << " ";
}
std::cout << std::endl;
}
int main() {
std::vector<int> myVector = {1, 2, 3, 4, 5};
std::list<std::string> myList = {"Hello", "World"};
printContainer(myVector);
printContainer(myList);
return 0;
}
Output:
1 2 3 4 5
Hello World
In this example, we define a template function printContainer
that takes a container as an argument. Inside the function, we use typename Container::value_type
to access the type of elements in the container. This allows us to iterate through the container and print its elements, regardless of whether it’s a vector of integers or a list of strings. This flexibility is one of the key advantages of using value_type
in STL containers.
Importance of Value_Type in Memory Management
Understanding value_type
is not just about type safety; it also plays a significant role in memory management. When you declare a container, the value_type
determines how memory is allocated and deallocated for the elements. For instance, if you have a container of complex objects, knowing the value_type
allows you to manage resources efficiently.
Here’s an example demonstrating the importance of value_type
when working with custom objects.
#include <iostream>
#include <vector>
class MyClass {
public:
MyClass(int id) : id(id) {}
void display() const { std::cout << "MyClass ID: " << id << std::endl; }
private:
int id;
};
int main() {
std::vector<MyClass> myObjects;
myObjects.emplace_back(1);
myObjects.emplace_back(2);
for (const MyClass& obj : myObjects) {
obj.display();
}
return 0;
}
Output:
MyClass ID: 1
MyClass ID: 2
In this example, we create a vector of MyClass
objects. By using emplace_back
, we efficiently construct MyClass
objects directly in the vector, leveraging the value_type
to manage memory allocation. Understanding value_type
becomes crucial when dealing with dynamic memory and complex objects, ensuring that resources are utilized effectively.
Conclusion
The value_type
in STL containers is a fundamental concept that every C++ developer should grasp. It facilitates type safety, enhances template programming, and plays a critical role in memory management. By understanding how to access and utilize value_type
, you can write more efficient and reusable code. Whether you’re working with basic data types or complex objects, leveraging value_type
will help you create robust C++ applications. As you continue your journey in C++, remember that mastering these foundational concepts will set you apart as a proficient programmer.
FAQ
-
What is the purpose of value_type in STL containers?
value_type defines the type of elements that a specific STL container holds, ensuring type safety and enabling efficient memory management. -
How can I access the value_type of an STL container?
You can access the value_type using the syntax Container::value_type, which allows you to reference the type of elements stored in the container. -
Can I use value_type with custom objects?
Yes, value_type can be used with custom objects, allowing you to manage collections of user-defined types effectively. -
Why is value_type important for template programming?
value_type enhances template programming by allowing you to write generic functions that can operate on different container types without needing to know their specific types in advance. -
How does value_type impact memory management in C++?
value_type determines how memory is allocated and deallocated for the elements in a container, which is especially important when dealing with complex objects and dynamic memory.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook