How to Use switch Statement in C++
-
Use
switch
Statement to Construct Multi-Case Code Path Scenarios -
Use
default
Keyword to Specify a Default Code Path in theswitch
-
Use the
{}
Block Notation to Declare Local Variables in Different Code Paths
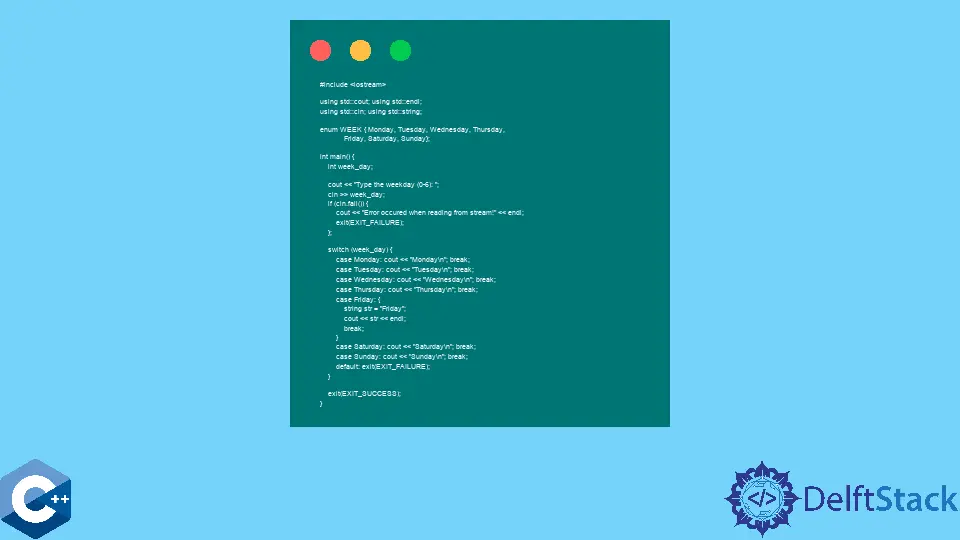
This article will explain several methods of how to use the switch
statement in C++.
Use switch
Statement to Construct Multi-Case Code Path Scenarios
The switch
keyword is used similarly to other block-based statements that evaluate a given expression as a condition. The only difference is that the expression must have an integral value compared to each code path specified by the case
keywords. Paths have the following structure - case label : statements
where the label
is the value compared to the expression in the switch
condition. If the expression matches one of the labels, program execution moves to the statements followed after that label. Note that if the case
block does not end with the break;
statement, the program will continue to execute statements in all following case
blocks until the break;
is not reached or the switch
scope itself is ended.
#include <iostream>
using std::cin;
using std::cout;
using std::endl;
using std::string;
enum WEEK { Monday, Tuesday, Wednesday, Thursday, Friday, Saturday, Sunday };
int main() {
int week_day;
cout << "Type the weekday (0-6): ";
cin >> week_day;
if (cin.fail()) {
cout << "Error occured when reading from stream!" << endl;
exit(EXIT_FAILURE);
};
switch (week_day) {
case Monday:
cout << "Monday\n";
break;
case Tuesday:
cout << "Tuesday\n";
break;
case Wednesday:
cout << "Wednesday\n";
break;
case Thursday:
cout << "Thursday\n";
break;
case Friday:
cout << "Friday\n";
break;
case Saturday:
cout << "Saturday\n";
break;
case Sunday:
cout << "Sunday\n";
break;
default:
exit(EXIT_FAILURE);
}
exit(EXIT_SUCCESS);
}
Use default
Keyword to Specify a Default Code Path in the switch
The default
keyword defines the special case label that gets executed if none of the declared cases match the switch
expression. The important point to be aware of is that every label block needs the break;
statement at the end to satisfy the basic logic of the switch
construct. Notice that the next code sample demonstrates the switch
statement without any breaks, which will behave rather abruptly. If the week_day
value matches the first label Monday
, then every cout
statement will be executed, including the one alongside the default label. On the other hand, if the week_day
value does not match any case label, then cout
statements starting with the default
label get executed.
#include <iostream>
using std::cin;
using std::cout;
using std::endl;
using std::string;
enum WEEK { Monday, Tuesday, Wednesday, Thursday, Friday, Saturday, Sunday };
int main() {
int week_day;
cout << "Type the weekday (0-6): ";
cin >> week_day;
if (cin.fail()) {
cout << "Error occured when reading from stream!" << endl;
exit(EXIT_FAILURE);
};
switch (week_day) {
case Monday:
cout << "Monday\n";
case Tuesday:
cout << "Tuesday\n";
case Wednesday:
cout << "Wednesday\n";
case Thursday:
cout << "Thursday\n";
case Friday:
cout << "Friday\n";
default:
cout << "Wrong number specified!\n";
case Saturday:
cout << "Saturday\n";
case Sunday:
cout << "Sunday\n";
}
exit(EXIT_SUCCESS);
}
Use the {}
Block Notation to Declare Local Variables in Different Code Paths
Like other block-based statements, switch
body has the scope in which variables can be declared, but cases require the user to have local variables in each case
construct. Note that statements from every case
path have shared scope unless declared inside a separate block inside {}
. Thus, if a variable is initialized in one case
, the statements in other cases can’t refer to the same variable. The following example implements the switch
statement where there is a local block for the case Friday
and the variable inside is not seen from other cases
.
#include <iostream>
using std::cin;
using std::cout;
using std::endl;
using std::string;
enum WEEK { Monday, Tuesday, Wednesday, Thursday, Friday, Saturday, Sunday };
int main() {
int week_day;
cout << "Type the weekday (0-6): ";
cin >> week_day;
if (cin.fail()) {
cout << "Error occured when reading from stream!" << endl;
exit(EXIT_FAILURE);
};
switch (week_day) {
case Monday:
cout << "Monday\n";
break;
case Tuesday:
cout << "Tuesday\n";
break;
case Wednesday:
cout << "Wednesday\n";
break;
case Thursday:
cout << "Thursday\n";
break;
case Friday: {
string str = "Friday";
cout << str << endl;
break;
}
case Saturday:
cout << "Saturday\n";
break;
case Sunday:
cout << "Sunday\n";
break;
default:
exit(EXIT_FAILURE);
}
exit(EXIT_SUCCESS);
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook