Typedef for Function Pointer in C++
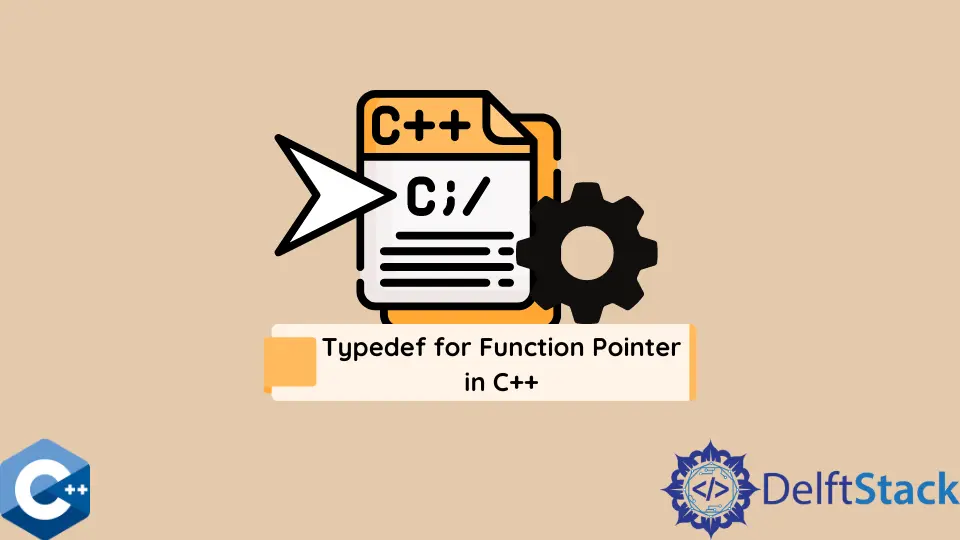
This article will explain the purpose of typedef
in C/C++. We will further discuss how we can use typedef
with a function pointer and what are the benefits of using it.
Let’s discuss typedef
and its common use first.
the typedef
Keyword
typedef
stands for type definition. As the name suggests, typedef
is a way of assigning a new name to an existing data type, the type of a variable.
For example, if you want to store an integer variable, the data type will be int. Similarly, the char or string data type is used for a word or phrase.
#include <iostream>
#include <string>
using namespace std;
int main() {
int a = 10;
string greeting = "Hello!";
return 0;
}
With the help of typedef
, you may detach yourself from the actual types being used and concentrate more on the idea of what a variable should signify. This makes writing clean code simpler, but it also makes editing your code much simpler.
For example, if you are recording the statements of three different players after a cricket match, then typedef
can be used.
#include <iostream>
using namespace std;
int main() {
typedef char* statement;
statement PlayerA = "I played bad";
statement PlayerB = "I played very well";
statement PlayerC = " I could not get the chance to Bat";
cout << "Player A said:" << PlayerA;
return 0;
}
In the above code, char*
is a character pointer on which we have applied the typedef
keyword for changing this data type with the new name statement
. This new alias is more meaningful in this case since we are recording the players’ statements.
Hence, the typedef
enhances the code readability.
The typedef
can also be used with function pointers. Before jumping into that, let us briefly introduce function pointers.
Function Pointers
In C++, pointers are variables that hold the memory address of a variable. Similarly, a function pointer is a pointer that holds the address of a function.
A function pointer can be declared with the following code:
int (*point_func)(int, int);
In the above code, point_func
is a pointer that points to a function having two integer variables as arguments and int as the return type.
typedef
With Function Pointer
The syntax looks somehow odd for typedef
with a function pointer. You only need to put the typedef
keyword at the start of the function pointer declaration.
typedef int (*point_func)(int, int);
The above command means you defined a new type with the name point_func
(a function pointer that takes two int arguments and returns an integer, i.e., int (*) (int, int)
). Now you can use this new name for pointers declaration.
Let’s look at the following programming example:
#include <iostream>
using namespace std;
int abc(int x1, int x2) { return (x1 * x2); }
int main() {
typedef int (*pair_func)(int, int);
pair_func PairProduct; // PairProduct is pointer of type pair_func
PairProduct = &abc; // PairProduct pointer holds the address of function abc
int product = (*PairProduct)(20, 5);
cout << "The product of the pair is: " << product;
return 0;
}
Output:
The product of the pair is: 100
It is clear up to this point what typedef
does if it is used before the function pointer. The abc
function takes two arguments and returns their product in the above code.
In the main
function, we used typedef
to define a new name for the function pointer (i.e., pair_func
). Then, we defined the PairProduct
of type pair_func
and assigned the function abc
address.
After that, we called the function abc
by dereferencing the pointer PairProduct
(simpler syntax) and passing two arguments.