Concept of Read/Write Locks in C++
- Understanding Read/Write Locks
- Implementing Read/Write Locks in C++
- Advantages of Using Read/Write Locks
- Potential Pitfalls
- Conclusion
- FAQ
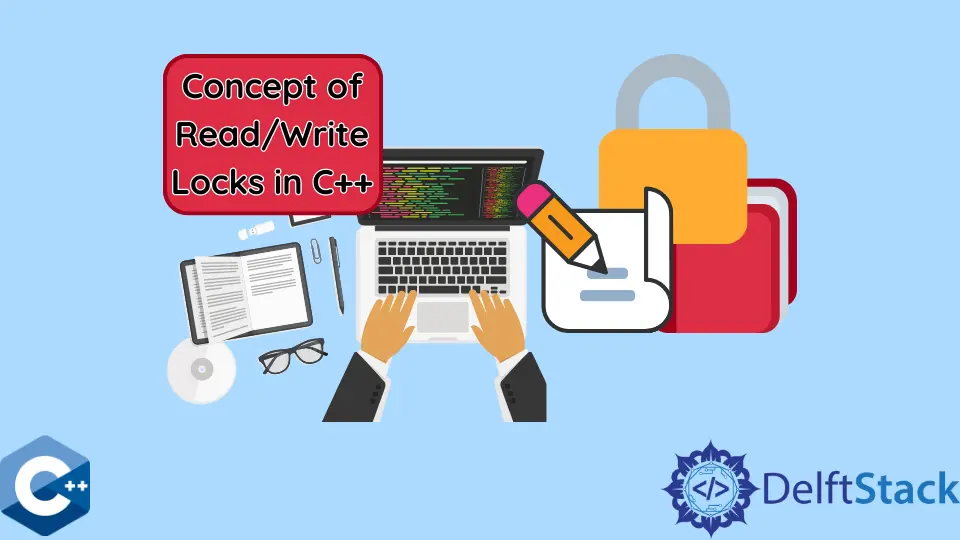
In the world of multi-threaded programming, managing access to shared resources is crucial. Enter the reader/writer lock—a powerful synchronization mechanism that allows multiple threads to read a resource concurrently while ensuring that only one thread can write to that resource at any given time. This concept is particularly useful in scenarios where read operations vastly outnumber write operations, optimizing performance without compromising data integrity.
In this article, we will explore the concept of read/write locks in C++, delve into their implementation, and examine their advantages and potential pitfalls. Whether you are a novice or an experienced developer, understanding this synchronization technique will enhance your ability to write efficient, thread-safe applications.
Understanding Read/Write Locks
Before diving into implementation, it’s essential to grasp what read/write locks are. A read/write lock allows multiple threads to read a shared resource simultaneously while preventing any thread from writing to that resource during the read operation. Conversely, when a thread wants to write to the resource, it must acquire the write lock, blocking all other threads from reading or writing until the lock is released. This balance between read and write operations enhances performance, especially in scenarios where reads are frequent and writes are rare.
Implementing Read/Write Locks in C++
C++ provides several mechanisms for implementing read/write locks, with the std::shared_mutex
and std::unique_lock
being the most common. The std::shared_mutex
allows multiple readers or a single writer to access the resource, while the std::unique_lock
ensures exclusive access for writing. Below is a simple example showcasing how to implement read/write locks in C++.
#include <iostream>
#include <shared_mutex>
#include <thread>
#include <vector>
class SharedResource {
int data;
std::shared_mutex rw_mutex;
public:
SharedResource() : data(0) {}
void read() {
std::shared_lock lock(rw_mutex);
std::cout << "Reading data: " << data << std::endl;
}
void write(int value) {
std::unique_lock lock(rw_mutex);
data = value;
std::cout << "Writing data: " << data << std::endl;
}
};
void reader(SharedResource& resource) {
resource.read();
}
void writer(SharedResource& resource, int value) {
resource.write(value);
}
int main() {
SharedResource resource;
std::vector<std::thread> threads;
for (int i = 0; i < 5; ++i) {
threads.emplace_back(reader, std::ref(resource));
}
threads.emplace_back(writer, std::ref(resource), 42);
for (auto& thread : threads) {
thread.join();
}
return 0;
}
Output:
Reading data: 0
Reading data: 0
Reading data: 0
Reading data: 0
Reading data: 0
Writing data: 42
In this example, we define a SharedResource
class that encapsulates a shared integer data
and a std::shared_mutex
for synchronization. The read
method acquires a shared lock, allowing multiple threads to read concurrently. The write
method acquires a unique lock, ensuring that only one thread can write at a time. In the main
function, we create multiple reader threads and a single writer thread to demonstrate the lock’s functionality.
Advantages of Using Read/Write Locks
Implementing read/write locks offers several advantages. First, they significantly improve performance in read-heavy applications by allowing multiple threads to access the resource simultaneously. This concurrency reduces bottlenecks and enhances throughput. Second, read/write locks maintain data integrity by ensuring that write operations are exclusive, preventing data races and inconsistencies. Lastly, they provide a flexible mechanism for managing access to shared resources, making them suitable for various applications, from databases to file systems.
However, it’s essential to use read/write locks judiciously. Overusing them can lead to complexity and potential deadlocks if not managed correctly. Therefore, developers must evaluate the specific needs of their applications and choose the appropriate synchronization mechanism.
Potential Pitfalls
While read/write locks can enhance performance, they are not without their challenges. One significant issue is the risk of writer starvation, where reader threads continually acquire locks, preventing writers from accessing the resource. This scenario can lead to performance degradation and unresponsive applications. To mitigate this, developers can implement strategies such as prioritizing writer access or limiting the number of concurrent readers.
Another potential pitfall is the increased complexity in code management. As the number of threads increases, so does the potential for race conditions and deadlocks. Developers must be vigilant in their design to ensure that locks are acquired and released appropriately.
In summary, while read/write locks can provide significant benefits, they require careful consideration and implementation to avoid pitfalls.
Conclusion
Understanding the concept of read/write locks in C++ is essential for any developer working with multi-threaded applications. By allowing multiple threads to read concurrently while ensuring exclusive access for writing, read/write locks optimize performance and maintain data integrity. However, they come with their own set of challenges, including potential writer starvation and increased complexity. By being aware of these pitfalls and implementing best practices, developers can leverage read/write locks to create efficient, thread-safe applications. As you continue to explore multi-threading in C++, remember that the right synchronization mechanism can make all the difference in the performance and reliability of your applications.
FAQ
-
What is a read/write lock?
A read/write lock is a synchronization mechanism that allows multiple threads to read a shared resource concurrently while ensuring that only one thread can write to that resource at a time. -
Why would I use a read/write lock instead of a mutex?
Read/write locks allow for greater concurrency in read-heavy scenarios, as multiple threads can read simultaneously without blocking each other, unlike a mutex which allows only one thread to access the resource at a time.
-
Can read/write locks lead to deadlocks?
Yes, improper management of read/write locks can lead to deadlocks, especially if locks are not acquired and released in a consistent order. -
How do I prevent writer starvation when using read/write locks?
You can implement strategies such as prioritizing writer access or limiting the number of concurrent readers to ensure that writers are not indefinitely blocked by readers. -
Are read/write locks available in all C++ standards?
Read/write locks, specificallystd::shared_mutex
, were introduced in C++17. Ensure that your compiler supports this version or later to use them.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook