How to Use Nested if-else Statements in C++
- Understanding Nested if-else Statements
- Benefits of Using Nested if-else Statements
- Best Practices for Nested if-else Statements
- Conclusion
- FAQ
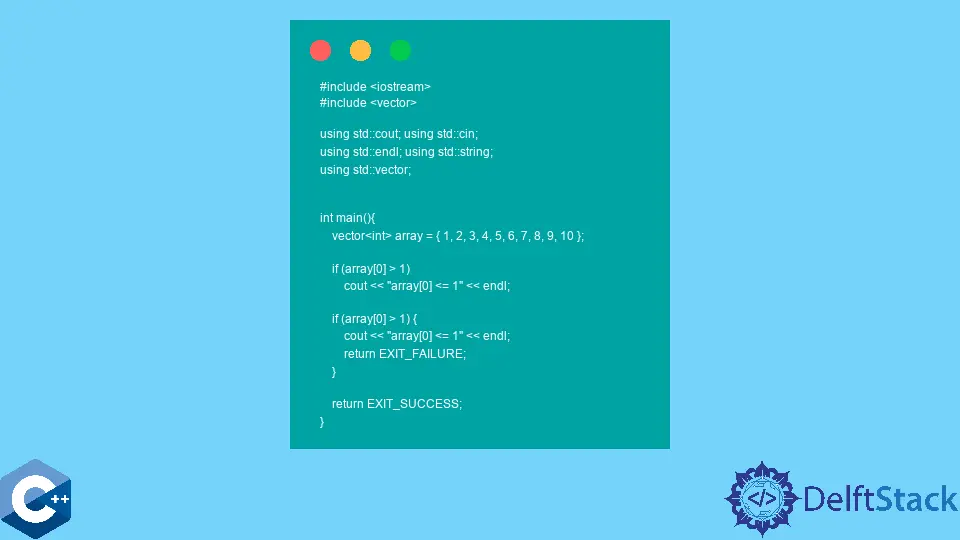
Nested if-else statements are a powerful feature in C++ that allow developers to create complex decision-making processes within their programs. When you need to evaluate multiple conditions and execute different blocks of code based on those conditions, nested if-else statements come in handy.
This article will guide you through the concept of nested if-else statements in C++, providing clear examples and explanations to help you grasp this essential programming technique. Whether you are a beginner looking to enhance your coding skills or an experienced developer seeking to refine your understanding, this guide will equip you with the knowledge you need to effectively implement nested if-else statements in your C++ programs.
Understanding Nested if-else Statements
Nested if-else statements occur when you place one if-else statement inside another. This structure allows you to evaluate multiple conditions in a hierarchical manner. For example, you might first check if a number is positive, and then, if it is, check if it is even or odd. This layering of conditions helps you create more intricate logic in your applications.
Here’s a straightforward example:
#include <iostream>
using namespace std;
int main() {
int number;
cout << "Enter a number: ";
cin >> number;
if (number > 0) {
if (number % 2 == 0) {
cout << "The number is positive and even." << endl;
} else {
cout << "The number is positive and odd." << endl;
}
} else if (number < 0) {
cout << "The number is negative." << endl;
} else {
cout << "The number is zero." << endl;
}
return 0;
}
In this example, the program prompts the user to enter a number. The first if
checks if the number is greater than zero. If true, it enters another nested if
to check if the number is even or odd. If the number is less than zero, it outputs that the number is negative, and if it’s zero, it states that the number is zero. This structure allows for clear and organized decision-making.
Output:
Enter a number: 5
The number is positive and odd.
Benefits of Using Nested if-else Statements
Using nested if-else statements provides several advantages in programming. First and foremost, they enhance the readability of your code. By clearly defining the conditions and their corresponding actions, you make it easier for others (and yourself) to understand the logic behind your decisions.
Additionally, nested if-else statements allow for more complex decision trees. In scenarios where you need to evaluate multiple conditions, this structure enables you to create detailed logic that can handle various cases. For instance, in a grading system, you might want to check not only if a score is passing or failing but also categorize it into letter grades based on ranges.
Consider this grading example:
#include <iostream>
using namespace std;
int main() {
int score;
cout << "Enter your score: ";
cin >> score;
if (score >= 90) {
cout << "You received an A." << endl;
} else if (score >= 80) {
cout << "You received a B." << endl;
} else if (score >= 70) {
cout << "You received a C." << endl;
} else if (score >= 60) {
cout << "You received a D." << endl;
} else {
cout << "You received an F." << endl;
}
return 0;
}
In this example, the program evaluates the score and categorizes it into letter grades. The nested structure allows for clear delineation between each grade category, making the logic straightforward and easy to follow.
Output:
Enter your score: 85
You received a B.
Best Practices for Nested if-else Statements
While nested if-else statements are powerful, they can also lead to code that is difficult to read and maintain if not used judiciously. Here are some best practices to keep in mind:
-
Limit Nesting Levels: Try to avoid excessive nesting. Too many levels can make your code hard to read. If you find yourself nesting too deeply, consider using switch statements or restructuring your logic.
-
Keep Conditions Simple: Each condition should be straightforward and easy to understand. Complicated conditions can confuse readers and lead to errors.
-
Use Meaningful Variable Names: This helps in understanding the purpose of each variable and makes your code self-documenting.
-
Consider Alternative Structures: In some cases, using a switch statement or polymorphism can be more effective than nested if-else statements, especially when dealing with multiple discrete values.
-
Comment Wisely: While the goal is to write self-explanatory code, a few well-placed comments can help clarify complex logic without cluttering the code.
By following these best practices, you can ensure that your use of nested if-else statements remains effective and enhances the quality of your code.
Conclusion
Nested if-else statements are a vital tool in C++ programming, allowing for complex decision-making processes that can enhance the functionality of your applications. By understanding how to implement and structure these statements effectively, you can create more organized, readable, and maintainable code. Remember to keep your conditions clear and your nesting manageable to maintain code quality. With practice, you’ll find that nested if-else statements can significantly improve your programming capabilities.
FAQ
-
What is a nested if-else statement?
A nested if-else statement is when one if-else statement is placed inside another, allowing for multiple conditions to be evaluated in a structured way. -
When should I use nested if-else statements?
Use nested if-else statements when you need to evaluate multiple conditions that depend on each other, such as checking for ranges or categories. -
Are there alternatives to nested if-else statements?
Yes, alternatives include using switch statements or employing polymorphism, especially when dealing with multiple discrete values. -
Can excessive nesting affect code readability?
Yes, excessive nesting can make code difficult to read and maintain. It’s best to limit the number of nested levels. -
How can I improve the readability of nested if-else statements?
You can improve readability by limiting nesting levels, keeping conditions simple, using meaningful variable names, and adding comments where necessary.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook