How to Instantiate a Template Class in C++
- Understanding Template Classes
- Instantiating a Template Class with Different Data Types
- Using Multiple Template Parameters
- Specializing Template Classes
- Conclusion
- FAQ
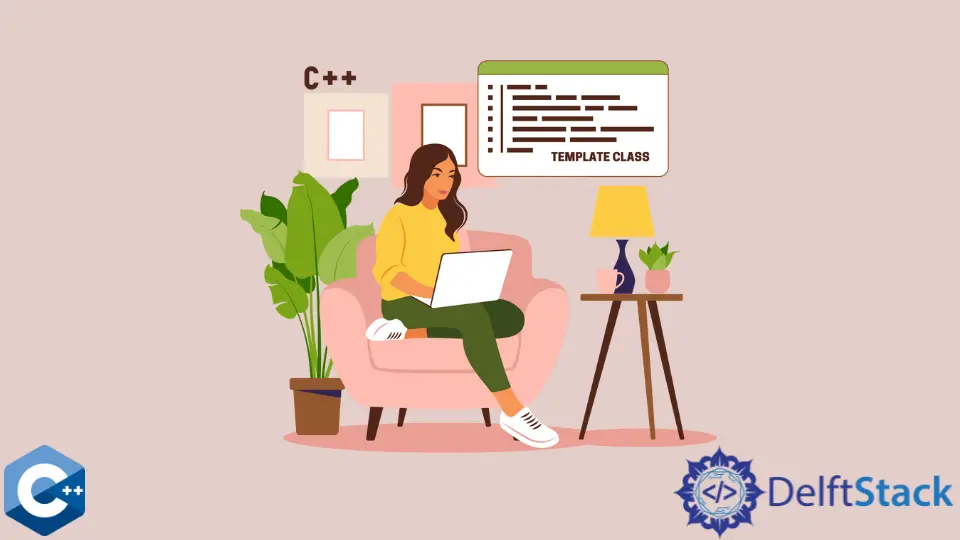
When diving into the world of C++, you’ll quickly encounter the concept of templates. Template classes allow developers to create flexible and reusable code that can work with any data type. This feature is particularly powerful because it promotes code efficiency and reduces repetition.
In this article, we’ll explore how to instantiate a template class by specifying the data types and parameters for all the template class methods. Whether you’re a beginner or an experienced programmer, understanding template classes is crucial for writing effective C++ code. Let’s get started!
Understanding Template Classes
Template classes in C++ are a powerful feature that allows developers to define a class with placeholder types. This means you can create a single class definition that can operate with any data type specified at the time of instantiation. The syntax for defining a template class is straightforward, but instantiating it correctly is where many developers face challenges.
To define a template class, you use the template
keyword followed by template parameters enclosed in angle brackets. Here’s a quick example of a simple template class:
template <typename T>
class Box {
public:
Box(T value) : value(value) {}
T getValue() { return value; }
private:
T value;
};
In this example, we define a template class Box
that can hold any data type. The constructor takes a value of type T
, and we have a method to retrieve that value.
Instantiating a Template Class with Different Data Types
To instantiate a template class, you need to specify the data type that you want to use. For example, if you want to create a Box
that holds an integer, you would do it like this:
int main() {
Box<int> intBox(123);
std::cout << intBox.getValue() << std::endl;
return 0;
}
Output:
123
In this code, we create an instance of Box
called intBox
, specifying int
as the data type. When we call the getValue()
method, it returns the integer stored in the box. Similarly, you can instantiate the same class for different data types, like double
or std::string
:
Box<double> doubleBox(45.67);
std::cout << doubleBox.getValue() << std::endl;
Box<std::string> stringBox("Hello, World!");
std::cout << stringBox.getValue() << std::endl;
Output:
45.67
Hello, World!
Here, we create two more instances of the Box
class: one for a double
and another for a std::string
. Each instance works seamlessly, demonstrating the versatility of template classes.
Using Multiple Template Parameters
Sometimes, you might want to define a class that can take multiple types. This is where multiple template parameters come into play. Here’s how you can define and instantiate a template class with two types:
template <typename T, typename U>
class Pair {
public:
Pair(T first, U second) : first(first), second(second) {}
T getFirst() { return first; }
U getSecond() { return second; }
private:
T first;
U second;
};
int main() {
Pair<int, std::string> pair(1, "One");
std::cout << pair.getFirst() << ", " << pair.getSecond() << std::endl;
return 0;
}
Output:
1, One
In this example, we define a Pair
class that takes two template parameters, T
and U
. We then create an instance of Pair
that holds an int
and a std::string
. The getFirst()
and getSecond()
methods allow us to retrieve the values stored in the pair.
Specializing Template Classes
Template specialization allows you to define a specific behavior for a particular data type. This can be very useful when you need different functionality for a specific type. Here’s how you can specialize a template class:
template <typename T>
class Box {
public:
Box(T value) : value(value) {}
void display() {
std::cout << value << std::endl;
}
private:
T value;
};
// Specialization for char
template <>
class Box<char> {
public:
Box(char value) : value(value) {}
void display() {
std::cout << "Character: " << value << std::endl;
}
private:
char value;
};
int main() {
Box<int> intBox(5);
intBox.display();
Box<char> charBox('A');
charBox.display();
return 0;
}
Output:
5
Character: A
In this example, we define a template class Box
and then provide a specialization for char
. The specialized Box
class has a different display()
method that formats the output differently. This flexibility allows you to tailor the behavior of your template classes based on specific data types.
Conclusion
Instantiating template classes in C++ is a powerful technique that enhances code reusability and flexibility. By understanding how to define and instantiate template classes, as well as how to use multiple parameters and specializations, you can write more efficient and maintainable code. Whether you’re working on a small project or a large application, mastering template classes will significantly improve your programming skills. So, dive into templates, experiment with different data types, and see how they can elevate your C++ coding experience!
FAQ
-
What is a template class in C++?
A template class is a blueprint for creating classes that can operate with any data type, defined using thetemplate
keyword. -
How do I instantiate a template class?
You instantiate a template class by specifying the data type within angle brackets when creating an object. -
Can I use multiple template parameters?
Yes, you can define a template class with multiple parameters by separating them with commas within the angle brackets. -
What is template specialization?
Template specialization allows you to provide a specific implementation for a particular data type, enabling custom behavior for that type. -
Are template classes type-safe?
Yes, template classes are type-safe, as the compiler checks the data types during compilation, reducing runtime errors.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook