Lambda Function in C++
- Syntax of Lambda Function in C++
- Implement a Lambda Function Using Regular Function Pointer in C++
-
Implement a Lambda Function Using
std::function
in C++ -
Implement a Lambda Function Using
auto
in C++ - Uses of a Lambda Function in C++
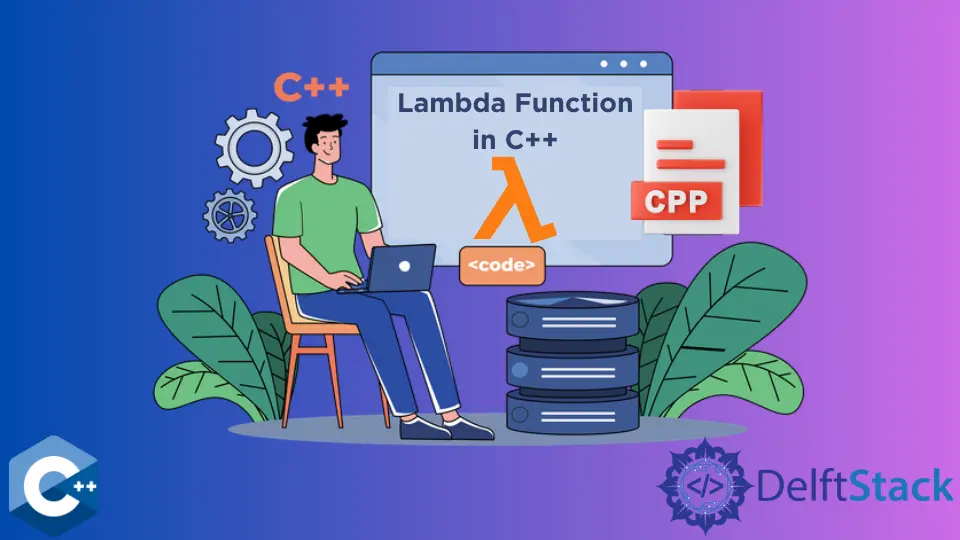
This article will discuss the syntax and implementation of Lambda functions in C++ in detail, along with relevant examples.
In C++, Lambda functions, or Anonymous functions, are defined anonymously inside another function. It is written inline in the source code and is passed as an argument to another function that takes a callable object.
This makes the creation of functions easy and quick for C++ programmers. These functions have no name and are not reused.
Syntax of Lambda Function in C++
General syntax:
[Capture List](Parameter List) { Function Body; }
The Capture List
tells the compiler that a lambda function is created.
To use any variable inside the function body, first, we need to capture it inside the capture list. Without doing so, it will be out of the scope of the function body.
The variable captured can be accessed either as a copy or as a reference. Capturing the variable is not considered the passing of an argument in a normal function.
Here, a
is captured by value:
auto f = [a]() { return a * 9; };
And here, a
is captured by reference:
auto f = [&a]() { return a++; };
The Parameter List
, as the name suggests, it takes arguments inside it like a normal function. It’s optional to write if there are no arguments to be passed.
With the ()
:
auto call_foo = [x]() { x.foo(); };
Without the ()
:
auto call_foo2 = [x] { x.foo(); };
Both these lambda functions above have the same meaning.
The Function Body
contains the code to be executed just like any other regular function.
int mul = 5;
auto ans = [mul](int a) { return a * mul; };
std::out << ans(2);
Output:
5
Example of Lambda function in C++:
#include <iostream>
#include <string>
using namespace std;
int main() {
auto sum = [](int a, int b) { return a + b; };
cout << "Sum of two integers:" << sum(5, 5) << endl;
return 0;
}
Output:
Sum of two integers: 10
We used the auto
keyword to indicate that the parameter’s data type would be determined at runtime. This will further be discussed in the following sections.
Implement a Lambda Function Using Regular Function Pointer in C++
We can implement a lambda function in C++ using a function pointer.
int main() {
double (*addNum1)(double, double){[](double a, double b) { return (a + b); }};
addNum1(1, 2);
}
Output:
3
Here, *addNum1
is a standard function pointer that works only with an empty capture clause. After that, the type of parameters is declared - double
, double
.
This is called a function prototype. You can observe an empty capture clause that indicates that a Lambda function is created for the compiler.
Furthermore, two variables are passed in the parameter list, double a
and double b
. And, inside the function body, the addition of these variables is returned.
After the main function is closed, the function addNum(1,2)
is called and returns 3 as the output.
Implement a Lambda Function Using std::function
in C++
std::function addNum{[](double a, double b) { return (a + b); }};
addNumbers2(3, 4);
Output:
7
The std::function
passes around lambda functions as parameters and return values. It allows you to declare the exact types for the argument list and the return value in the template.
Here, two variables are passed in the parameter list, double a
and double b
. And, inside the function body, the addition of these variables is returned.
After the main function is closed, the function addNum(1,2)
is called and returns 7 as the output.
Implement a Lambda Function Using auto
in C++
auto addNum{[](double a, double b) { return (a + b); }};
addNum(5, 6);
return 0;
Output:
11
We can’t use lambdas directly since they don’t have a type. As a result, when we create a lambda function, the compiler constructs a unique type of parameters.
Although we don’t know what type of lambda function it is, there are numerous methods to save it for usage after definition. The only method to use the lambda’s real type is through auto
.
Lambda functions can be used anywhere in the C++ program after declaring it as auto
.
Uses of a Lambda Function in C++
Lambda expressions are commonly used to encapsulate algorithms to pass them to another function.
A lambda function can be executed immediately after definition: [ ](double a, double b)
.
{return (a + b)}(); // immediately executed lambda expression
{ return (a + b) } // simple code block
As we can see, lambda expressions are an effective tool for restructuring complicated functions.
The process of explicit parameterization may then be carried out step by step, with intermediate testing in between. Once the code block has been appropriately parameterized, relocate it to an external location and make it a normal function.
Lambda expressions also allow building named nested functions, which can help remove unnecessary logic. When giving a non-trivial function as a parameter to another function, using named lambdas is easier for the eyes.
auto algo = [&](double x, double m, double b) -> double { return mx + b; };
int l = algorithm(1, 2, 3), m = algorithm(4, 5, 6);