How to Convert Enum to String in C++
-
Use
const char*
Array to Convert an Enum to a String in C++ - Use Custom Defined Function to Convert an Enum to a String in C++
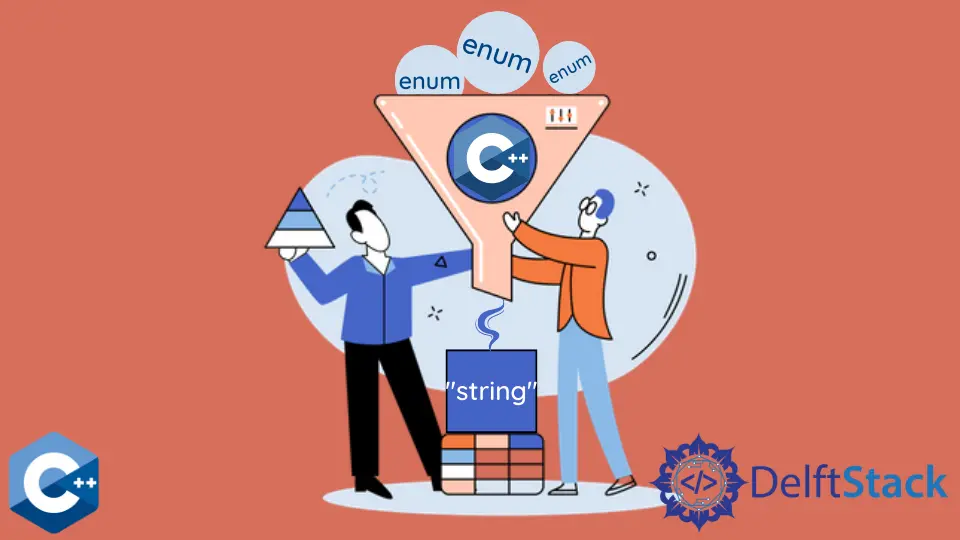
This article will explain several methods of converting an enumeration type to a string variable in C++.
Use const char*
Array to Convert an Enum to a String in C++
enum
is a built-in type, which can be used to declare small named integers usually formed as an array. This mechanism provides a less error-prone and more readable way of representing a set of integer values.
Enumeration elements may have a positional value ( like Banana
has 0
in our example code ), or it can have an explicit value (declared as enum Fruit { Banana = 34, ...}
).
This example shows how implicit values of enum
elements can be utilized to access corresponding string values from an array. Notice that we initialize random input
variable with the value from 1-4 range at the beginning of the main
function to demonstrate the real-world scenario better.
#include <chrono>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
enum Fruit { Banana, Coconut, Mango, Carambola, Total };
static const char *enum_str[] = {"Banana Pie", "Coconut Tart", "Mango Cookie",
"Carambola Crumble"};
int main() {
std::srand(std::time(nullptr));
int random = rand() % Total;
auto input = static_cast<Fruit>(random);
switch (input) {
case Banana:
cout << enum_str[Banana] << endl;
break;
case Coconut:
cout << enum_str[Coconut] << endl;
break;
case Mango:
cout << enum_str[Mango] << endl;
break;
case Carambola:
cout << enum_str[Carambola] << endl;
break;
case Total:
cout << "Incorrect ingredient!" << endl;
break;
}
return EXIT_SUCCESS;
}
Output (*random
):
Banana Pie
Use Custom Defined Function to Convert an Enum to a String in C++
Alternatively, we can define our own function, which takes an integer as a parameter and returns a string value. The string variable is initialized with a const char*
value from enum_str
array inside the function. If you pass the Total
value as an argument to the getStringForEnum
function, it will return some garbage value since the enum_str
array only has 4 elements.
#include <chrono>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
enum Fruit { Banana, Coconut, Mango, Carambola, Total };
static const char *enum_str[] = {"Banana Pie", "Coconut Tart", "Mango Cookie",
"Carambola Crumble"};
string getStringForEnum(int enum_val) {
string tmp(enum_str[enum_val]);
return tmp;
}
int main() {
string todays_dish = getStringForEnum(Banana);
cout << todays_dish << endl;
return EXIT_SUCCESS;
}
Output:
Banana Pie
The inclusion of the Total
element in the above enum
type can have practical usage. For one thing, it represents the number of elements in the enumeration that can be utilized as a loop parameter, as shown in the following sample code. The first loop extracts every string from the enum_str
array and pushes them to the enum_strigs
vector variable. The second iteration prints out the contents of enum_strigs
to console.
#include <chrono>
#include <iostream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
enum Fruit { Banana, Coconut, Mango, Carambola, Total };
static const char *enum_str[] = {"Banana Pie", "Coconut Tart", "Mango Cookie",
"Carambola Crumble"};
string getStringForEnum(int enum_val) {
string tmp(enum_str[enum_val]);
return tmp;
}
int main() {
vector<string> enum_strigs{};
enum_strigs.reserve(Total);
for (int i = 0; i < Total; ++i) {
enum_strigs.push_back(getStringForEnum(i));
}
for (const auto &item : enum_strigs) {
cout << item << endl;
}
return EXIT_SUCCESS;
}
Output:
Banana Pie
Coconut Tart
Mango Cookie
Carambola Crumble
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook