How to Clear the Console in C++
- Method 1: Using system(“CLS”) on Windows
- Method 2: Using system(“CLEAR”) on Linux and macOS
- Method 3: Using ANSI Escape Codes
- Conclusion
- FAQ
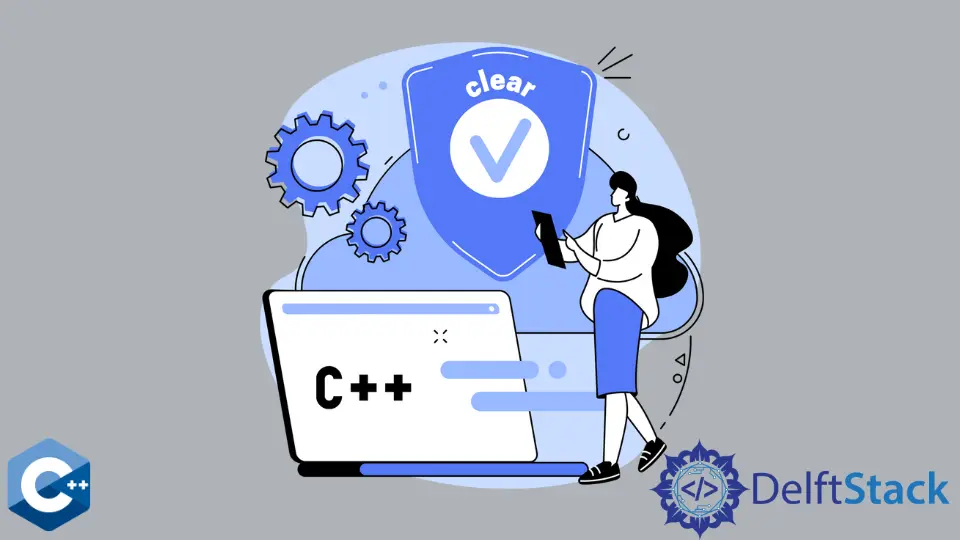
When working on C++ projects, you may find yourself needing to clear the console to enhance readability or remove clutter from previous outputs. This can be particularly useful during debugging or when you want to refresh the display for a new set of outputs. While C++ doesn’t have a built-in function for clearing the console, there are several methods you can use depending on your operating system.
In this article, we will explore different ways to clear the console in C++, providing you with practical code examples to implement in your projects. Whether you are a beginner or an experienced programmer, you’ll find these techniques helpful in managing your console output effectively.
Method 1: Using system(“CLS”) on Windows
If you are developing on a Windows platform, the simplest way to clear the console is by using the system("CLS")
command. This command invokes the Windows command line to clear the console screen. Here’s how you can implement it in your code:
#include <iostream>
#include <cstdlib>
int main() {
std::cout << "This is some output." << std::endl;
system("CLS");
std::cout << "The console has been cleared!" << std::endl;
return 0;
}
Output:
This is some output.
After executing the system("CLS")
command, the console is cleared, and you will see:
The console has been cleared!
In this example, we include the <cstdlib>
header, which allows us to use the system
function. The system("CLS")
command sends a signal to the command prompt to clear the screen. This method is straightforward and works efficiently on Windows systems. However, using system
is generally discouraged in production code due to potential security risks and portability issues.
Method 2: Using system(“CLEAR”) on Linux and macOS
For users on Linux or macOS, the command to clear the console is slightly different. You would use system("CLEAR")
. Here’s how you can implement this in your C++ code:
#include <iostream>
#include <cstdlib>
int main() {
std::cout << "This is some output." << std::endl;
system("clear");
std::cout << "The console has been cleared!" << std::endl;
return 0;
}
Output:
This is some output.
After executing the system("clear")
command, the console is cleared, and you will see:
The console has been cleared!
Just like the Windows method, we include the <cstdlib>
header for the system
function. The command system("clear")
instructs the terminal to clear the screen. While this method is effective for Linux and macOS users, it shares the same drawbacks as the Windows method regarding security and portability.
Method 3: Using ANSI Escape Codes
Another way to clear the console that works across different operating systems is by using ANSI escape codes. This method is more portable and does not rely on the system
function. Here’s how to implement it:
#include <iostream>
int main() {
std::cout << "This is some output." << std::endl;
std::cout << "\033[2J\033[1;1H"; // Clear the screen and move the cursor to the top left
std::cout << "The console has been cleared!" << std::endl;
return 0;
}
Output:
This is some output.
After executing the ANSI escape code, you will see:
The console has been cleared!
In this example, we use the escape sequence \033[2J
to clear the entire screen and \033[1;1H
to move the cursor to the top-left corner of the console. This method is advantageous because it works on many terminal emulators and is not dependent on the underlying operating system. However, it may not work in all environments, such as some IDEs or Windows Command Prompt without proper configuration.
Conclusion
Clearing the console in C++ can greatly enhance the user experience, especially during interactive applications or while debugging. While methods like system("CLS")
and system("clear")
are straightforward and easy to implement, using ANSI escape codes provides a more portable solution. Depending on your development environment and requirements, you can choose the method that best suits your needs. Whichever approach you decide to use, mastering console management is an essential skill for any C++ programmer.
FAQ
-
What is the best way to clear the console in C++?
Using ANSI escape codes is often considered the best method as it is more portable across different operating systems. -
Can I use system(“CLS”) on Linux?
No,system("CLS")
is specific to Windows. On Linux, you should usesystem("clear")
. -
Are there any security risks associated with using the system command?
Yes, usingsystem
can pose security risks, especially if user input is involved. It is better to use safer alternatives when possible. -
Will ANSI escape codes work in all terminal applications?
While ANSI escape codes work in many terminal applications, they may not function correctly in some IDEs or older terminal emulators. -
Is there a built-in function in C++ to clear the console?
No, C++ does not provide a built-in function to clear the console. You must use external commands or ANSI codes.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook