Static Function in C++
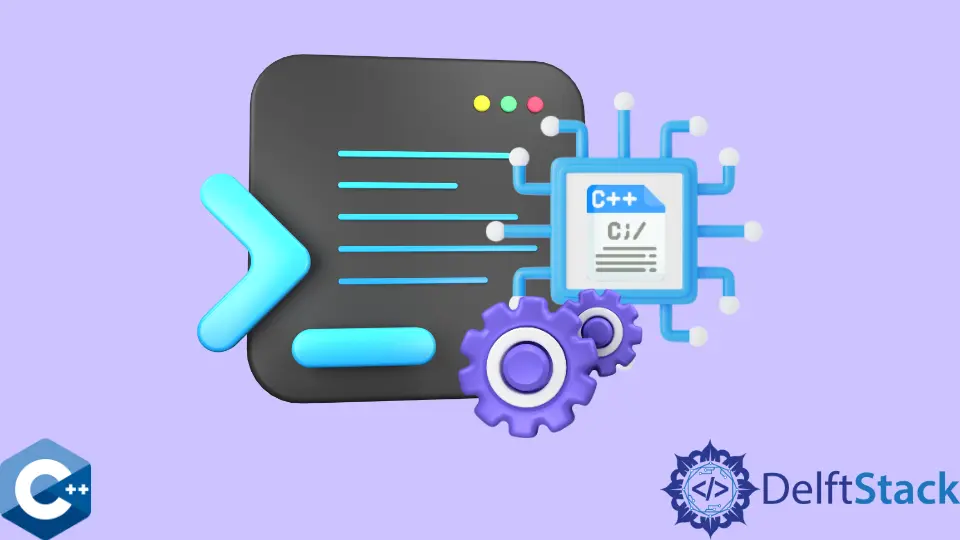
This article will demonstrate how to use static member functions of the class in C++.
Use the static
Member Functions to Access private
static
Member Variables
The static
keyword can be used in C++ to declare members of the class associated with the class itself rather than any particular instance.
Static member variables are declared inside the class body but can’t be initialized at the same time unless they are constexpr
qualified, const
qualified integral types, or const
qualified enum
. So, we need to initialize non-const static members outside of the class definition as we would any other global variable.
Notice that, even if the given static member has a private
specifier, it can be accessed in global scope using the class scope resolution operator as BankAccount::rate
is initialized in the following code snippet. Once the rate
member is initialized at the program start, it lives until the program terminates. If the user does not explicitly initialize static members, the compiler will use the default initializer.
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
class BankAccount {
private:
// static double rate = 2.2; / Error
static double rate;
double amount;
public:
explicit BankAccount(double n) : amount(n) {}
static double getRate() { return rate; }
static void setNewRate(double n) { rate = n; }
};
double BankAccount::rate = 2.2;
int main() {
BankAccount m1(2390.32);
BankAccount m2(1210.51);
return EXIT_SUCCESS;
}
BankAccount::rate
is not stored in every BankAccount
object. There is only one rate
value associated with the BankAccount
class, and consequently, every instance will access the same value at the given moment. This behavior is very useful in class design, but in this case, we will focus on the static
member functions.
The latter ones are usually used to access static member variables as member access control specifiers apply when the users of the class want to interact with them.
Namely, if we want to retrieve the value of the rate
which has a private
specifier, we need to have a corresponding public
member function. Also, we don’t want to access these members with individual calls objects, as there is only one value for the class itself.
So, we implement a function named getRate
, a static member function and returns the value of rate
. Then we can access the value of rate
directly from the main
function without constructing an object of BankAccount
type. Note that static member functions do not have this
implicit pointer available to them, and they can’t access/modify non-static members of the class.
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
class BankAccount {
private:
static double rate;
double amount;
public:
explicit BankAccount(double n) : amount(n) {}
static double getRate() { return rate; }
static void setNewRate(double n) { rate = n; }
};
double BankAccount::rate = 2.2;
int main() {
cout << BankAccount::getRate() << endl;
BankAccount::setNewRate(2.4);
cout << BankAccount::getRate() << endl;
return EXIT_SUCCESS;
}
Output:
2.2
2.4
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook