How to Read JSON File in C++
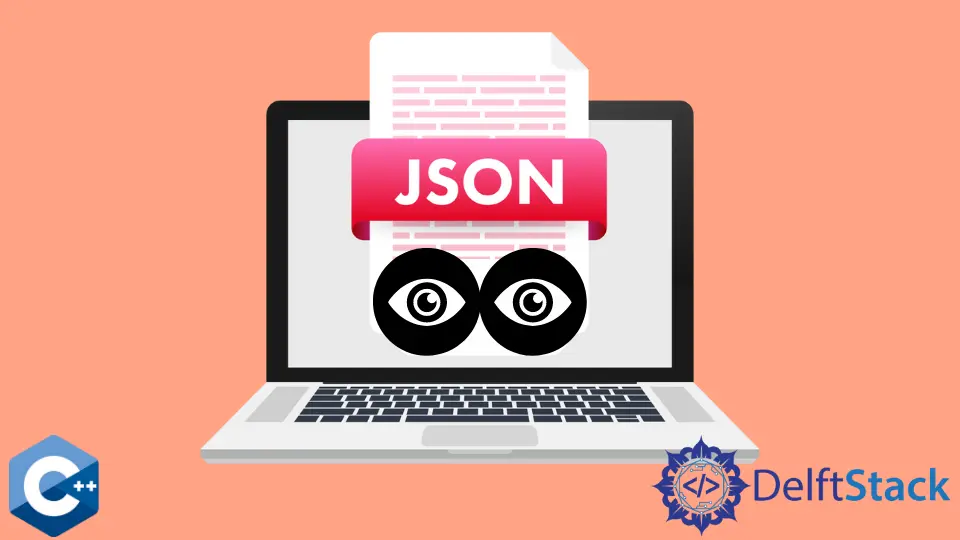
This tutorial will explain the concept of making a JSON file and then reading data from that file in the compiler. We will use C++ language and the jsoncpp
library.
This article uses Linux operating system to do the said task. However, it can also be done on Windows operating system in the C++ compiler.
We have to install the required libraries for reading JSON files. We will see how to install Linux on virtual machines and then install the essential libraries to execute our program.
We must include the following libraries in the code snippet for reading JSON files.
#include <jsoncpp/json/json.h>
#include <jsoncpp/json/value.h> //This library is used for interacting with JSON file
#include <fstream> // This library is used for reading and writing data to the files
#include <iostream> // This library defines standard input/ output objects
#include <string> // This library is used to store text
JSON File
JSON stands for JavaScript Object Notation
. JSON is used to store and exchange data.
It is not related to JavaScript. JSON is a data format used between the client and the server computer for sending and receiving data.
We have different objects in JSON. Pairs of keys
and their values
are enclosed in the curly braces.
JSON is a lightweight data format. JSON does not support comments
and namespaces
.
The format of JSON is below.
var person={"firstname":"Ali","Lastname":"Ahmad"};
Six datatypes are allowed in JSON.
- String
- Number
- Boolean
- Array
- Object
- Null
{
"name": "ali",
"age" : 30,
"married": false,
"kids" : ,
"hobbies": ["music","sports"],
"vehicle":{
{"type": "car", "vname": "swift"},
{"type": "bike", "vname": "honda"}
}
};
The first one is String
, the second one is Number
, the third one is Boolean
, the fourth one is Null
, the fifth one is Array
, and the sixth one is an Object
. This is the human-readable format.
Read Data From JSON File
First, we must download and install Ubuntu on your Windows by following these steps. Steps to install Ubuntu on your machine by Virtual Box.
After installing Ubuntu, we will get this screen.
We have to search the terminal by clicking on the icon having nine dots. Then a search bar will appear, and we must type terminal
on that screen.
Then after getting this terminal, we first have to install the compiler by typing the following command.
sudo apt install gcc
After this, you must enter the login password and give Y
for yes to install the packages.
After this installation, we must install a library for JSON in dev
.
sudo apt install libjson-cpp-dev
After installing this library of JSON, we have to open Text Editor
and write the following code in the JSON file.
{
"Name":"Alice",
"Dob" : "17th october 2022",
"College" : "Kips"
}
The following code snippet will explain how to read JSON files in C++.
#include <jsoncpp/json/json.h>
#include <jsoncpp/json/value.h>
#include <fstream>
#include <iostream>
#include <string>
using namespace std;
int main() {
// Using fstream to get the file pointer in file
ifstream file("file.json");
Json::Value actualJson;
Json::Reader reader;
// Using the reader, we are parsing the json file
reader.parse(file, actualJson);
// The actual json has the json data
cout << "Total json data:\n" << actualJson << endl;
// accessing individual parameters from the file
cout << "Name:" << actualJson["Name"] << endl;
cout << "Dob:" << actualJson["Dob"] << endl;
cout << "College:" << actualJson["College"] << endl;
return 0;
}
First, this code includes the libraries of input/output functions, reading data from files, and reading characters. Then JSON.h
is the header you will need to access all the functions.
Then we use fstream
to get the file pointer in the file. After opening the JSON file, we used the function Reader
to parse the file for reading file contents.
Then all the data in the JSON file is displayed on the screen using the output function in C++. Then we accessed the value of the individual parameter from the JSON file and displayed it on the screen.
The above code is used to read data from the JSON file. After writing the above code, we must compile and execute it using the command.
g++ test.cpp -ljsoncpp
./a.out
Output: