How to Define and Use Negative Infinity in C++
-
Use Negative Product of
numeric_limits::infinity()
in C++ -
Use the
INFINITY
Definition From thecmath
Library
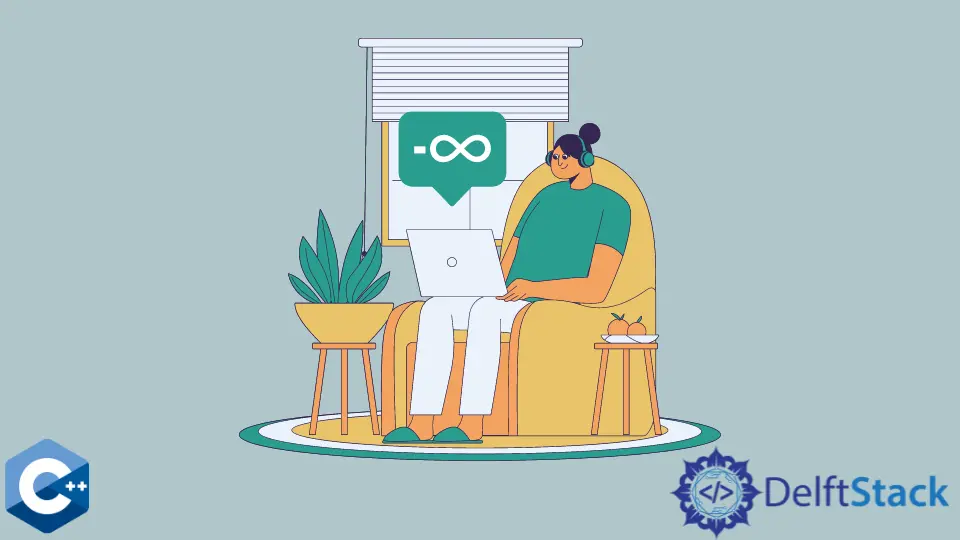
This article discusses the problem of representing negative infinity in C++ and lists some possible solutions.
C++ uses the IEEE-754 standard to represent the floating-point numbers. There are three floating-point data types: float
, double
, and long double
.
All of these variable types allow the storage of numerical values that cannot be stored in the int
data type.
Infinity is written as inf
in C++ and results in dividing a positive numeric value by a null value or by calculating a numerical value greater than what can be stored in 64 bits.
The unsigned or positive floating infinity value is defined in various C++ libraries such as cmath
and limits
, but there is no standard for negative infinity in C++.
Since there is no standard way to represent negative infinity in C++, here are several reliable methods to represent and use negative infinity in C++.
Use Negative Product of numeric_limits::infinity()
in C++
Use the limits
library in C++.
#include <iostream>
#include <limits>
using namespace std;
int main() {
float f = numeric_limits<float>::infinity();
float negInf = f * -1;
cout << "The value of f is = " << f << endl;
cout << "The value of negInf is = " << negInf << endl;
cout << "The value of f + negInf is = " << f + negInf << endl;
return 0;
}
In the code snippet above, the infinity
method from the limits
library returns the value of positive infinity.
The syntax is as follows.
numeric_limits<T>::infinity()
In the definition above, the T
in between the angled brackets stands for template class and, in actual implementation, is replaced by the data type with which you want to use the numeric_limits
methods. In the code snippet above, the float
data type is used, but double
can also be used similarly.
However, the infinity()
method is valid only for non-integral data types as integral data types like int
and bool
are inherently finite.
The output for the code above is as follows.
The value of f is = inf
The value of negInf is = -inf
The value of f + negInf is = nan
As seen in the example above, using the infinity()
method, the value of positive infinity is assigned to the float
type variable f
. Then a very simple and intuitive approach, namely multiplying f
by -1, is used, and the result is stored in the variable named negInf
, which results in the value of negative infinity being stored in negInf
.
As seen in the output above, this valid method assigns a negative infinity value to a variable. To further test this method, f
and negInf
are added together, resulting in nan
being returned, which stands for Not-a-Number
and indicates an incalculable value.
This result is consistent with the IEEE-754 rules for infinity values and hence computationally safe.
Use the INFINITY
Definition From the cmath
Library
The cmath
library is another C++ library with many useful methods and functions to deal with common mathematical operations and transformations.
The library also includes its definition of the infinity value, which is named INFINITY
and can be used in much the same way as numeric_limits<T>::infinity()
and can directly be assigned to both float
and double
data types.
Using the same method as in the previous example, multiplying the value of unsigned or positive infinity by a negative value returns us to the negative infinity value.
#include <cmath>
#include <iostream>
using namespace std;
int main() {
long double f = INFINITY;
long double negInf = f * -1;
cout << "The value of f is = " << f << endl;
cout << "The value of negInf is = " << negInf << endl;
cout << "The value of f + negInf is = " << f + negInf << endl;
return 0;
}
The output for the code above is as follows.
The value of f is = inf
The value of negInf is = -inf
The value of f + negInf is = nan
As can be seen, both methods produce the same output, and both can be safely used to implement negative infinity in C++.