How to Fix Segmentation Fault in C++
- Importance of Fixing Segmentation Fault Error in C++
- Segmentation Fault Causes and Solutions in C++
- Best Practices for Preventing Segmentation Faults in C++
- Conclusion
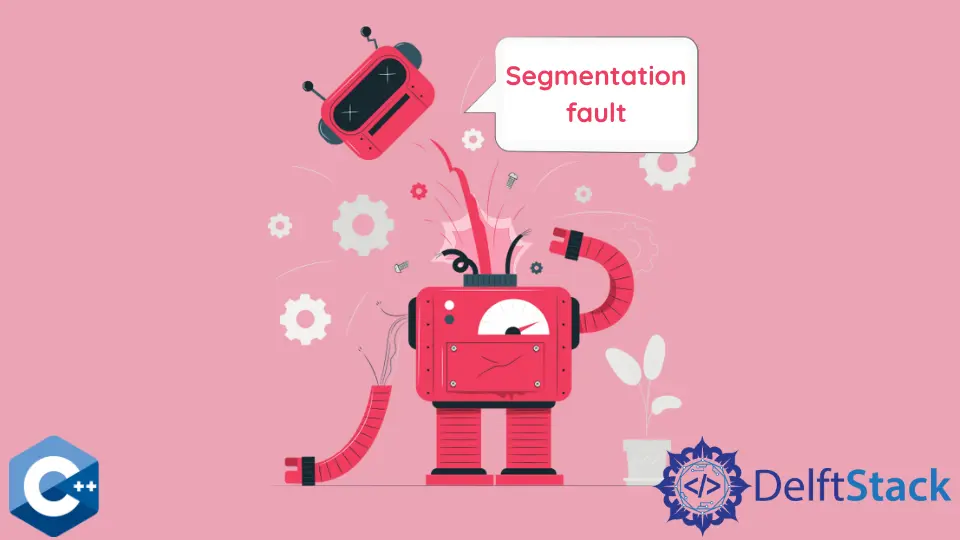
Segmentation faults, commonly known as segfaults
, are notorious bugs in C++ programs that can lead to crashes and unpredictable behavior. These faults typically occur due to memory-related errors such as dereferencing null pointers, out-of-bounds array access, memory leaks, and more.
Importance of Fixing Segmentation Fault Error in C++
Fixing segmentation fault errors in C++ is crucial because they indicate a serious issue with memory access. Segmentation faults occur when a program tries to access memory that it doesn’t have permission to access, leading to crashes or unpredictable behavior.
These errors can result from various issues, such as null pointer dereferencing, out-of-bounds array access, or memory leaks. Ignoring segmentation faults can lead to unreliable software, jeopardizing data integrity and user experience.
By addressing segmentation faults promptly, developers ensure the stability and reliability of their C++ programs, providing users with a smoother and safer computing experience.
Segmentation Fault Causes and Solutions in C++
Segmentation faults are common runtime errors in C++ programs that occur when a program tries to access memory that it doesn’t have permission to access. These errors can lead to crashes or unexpected behavior, making them a significant concern for C++ developers.
Understanding the various causes of segmentation faults and knowing how to address them is essential for writing robust and reliable C++ code.
In this table, we explore the different causes of segmentation faults in C++ programs, along with corresponding solutions to fix them. Each row of the table highlights a specific issue that can lead to segmentation faults, identifies its root cause, and provides actionable solutions to mitigate the problem.
By addressing these issues proactively, developers can ensure the stability and integrity of their C++ applications.
Issue | Cause | Solution |
---|---|---|
Dereferencing Null Pointers | Trying to access or modify memory through a null pointer. | Ensure that all pointers are properly initialized before using them. Check for null pointers before dereferencing them. |
Out-of-Bounds Array Access | Accessing an array element beyond its bounds. | Double-check array indices to ensure they are within the valid range (from 0 to size-1 ). Use standard library containers like std::vector or std::array , which offer bounds checking. |
Uninitialized Variables | Using variables without initializing them first. | Initialize all variables before using them. Compiler warnings can help identify uninitialized variables. |
Memory Leaks | Dynamically allocated memory that is not properly deallocated. | Use smart pointers (std::unique_ptr , std::shared_ptr ) or ensure that delete or delete[] is called for every new or new[] , respectively. |
Stack Overflow | Excessive recursion or allocation of large objects on the stack. | Optimize recursive algorithms, increase stack size (if possible), or allocate large objects on the heap. |
Incorrect Memory Management | Mixing delete and delete[] or new and malloc /free , or freeing memory more than once. |
Use consistent memory management techniques throughout the program. Avoid mixing different memory allocation/deallocation methods. |
Accessing Freed Memory | Accessing memory that has already been deallocated. | Ensure that memory is not accessed after it has been freed. Use tools like Valgrind or AddressSanitizer to detect such errors. |
Pointer Arithmetic Errors | Incorrect pointer arithmetic leading to accessing invalid memory locations. | Be cautious with pointer arithmetic. Make sure that pointers are properly incremented or decremented within the valid range. |
Using Deleted Objects | Accessing objects that have been deleted. | Avoid using objects after they have been deleted. Ensure that all references or pointers to an object are invalidated after deletion. |
Corrupted Data Structures | Modifying data structures in a way that corrupts their internal integrity. | Carefully review data structure manipulation operations. Ensure that all operations maintain the integrity of the data structure. |
Overall, by understanding and addressing these common causes of segmentation faults, developers can write more robust and reliable C++ code, leading to improved software quality and a better user experience.
Segfaults
occur when a program tries to access memory that it doesn’t have permission to access. These errors can be elusive and challenging to debug, but understanding their causes and implementing proper solutions is crucial for writing robust and reliable C++ code.
Let’s start by examining a C++ program that demonstrates various causes of segmentation faults and how to fix them.
#include <iostream>
#include <vector>
// Function to demonstrate stack overflow
int recursive_function(int n) {
if (n <= 0) return 0;
return n + recursive_function(n - 1);
}
int main() {
// 1. Dereferencing Null Pointers
int* ptr = nullptr;
// *ptr = 10; // Uncommenting this line will cause a segfault
if (ptr != nullptr) {
*ptr = 10; // Fixed: Checking for nullptr before dereferencing
std::cout << "Dereferencing Null Pointer: " << *ptr << std::endl;
} else {
std::cout << "Dereferencing Null Pointer: Pointer is nullptr" << std::endl;
}
// 2. Out-of-Bounds Array Access
int arr[5] = {1, 2, 3, 4, 5};
// std::cout << arr[5]; // Uncommenting this line will cause a segfault
if (5 < sizeof(arr) / sizeof(arr[0])) {
std::cout << "Out-of-Bounds Array Access: " << arr[5]
<< std::endl; // Fixed: Checking array bounds
} else {
std::cout << "Out-of-Bounds Array Access: Index is out of bounds"
<< std::endl;
}
// 3. Uninitialized Variables
int uninitialized_var;
// std::cout << uninitialized_var; // Uncommenting this line will cause
// undefined behavior
uninitialized_var = 42; // Fixed: Initializing the variable
std::cout << "Uninitialized Variable: " << uninitialized_var << std::endl;
// 4. Memory Leaks
int* leak = new int;
delete leak; // Fixed: Deallocating memory to prevent memory leak
std::cout << "Memory Leak: No memory leak" << std::endl;
// 5. Stack Overflow
// Recursive function causing stack overflow
// int result = recursive_function(100000); // Uncommenting this line will
// cause a stack overflow
std::cout << "Stack Overflow: Recursive function will cause a stack overflow "
"if uncommented"
<< std::endl;
// 6. Incorrect Memory Management
// Mixing delete and delete[]
// char* buffer = new char[10];
// delete buffer; // Uncommenting this line will cause undefined behavior
// Fixed: Removed incorrect memory management example
// 7. Accessing Freed Memory
int* freed_ptr = new int;
delete freed_ptr;
// *freed_ptr = 5; // Uncommenting this line will cause a segfault
freed_ptr = nullptr; // Fixed: Setting pointer to nullptr after deletion
if (freed_ptr != nullptr) {
*freed_ptr = 5; // This line won't be executed
} else {
std::cout << "Accessing Freed Memory: Pointer is nullptr" << std::endl;
}
// 8. Pointer Arithmetic Errors
int* p = new int[5];
// p += 10; // Uncommenting this line will cause a segfault
p += 4; // Fixed: Ensuring pointer stays within allocated memory
std::cout << "Pointer Arithmetic Errors: " << *p << std::endl;
// 9. Using Deleted Objects
// Accessing deleted object
// int* deleted_obj = new int;
// delete deleted_obj;
// *deleted_obj = 10; // Uncommenting this line will cause a segfault
// Fixed: Removed incorrect example of using deleted objects
// 10. Corrupted Data Structures
std::vector<int>* vec = new std::vector<int>{1, 2, 3};
// vec->push_back(4); // Uncommenting this line will cause a segfault due to
// corrupted vector
vec->push_back(4); // Fixed: Adding elements to the vector correctly
std::cout << "Corrupted Data Structures: ";
for (int val : *vec) {
std::cout << val << " ";
}
std::cout << std::endl;
// Clean up
delete[] p;
delete vec;
return 0;
}
We’ve addressed several common causes of segmentation faults in C++ and provided simple solutions for each. First, to prevent dereferencing null pointers, we ensured the pointer was not nullptr
before accessing its value.
For out-of-bounds array access, we added checks to ensure the index remained within the array’s bounds. Uninitialized variables were initialized before use to avoid undefined behavior.
Memory leaks were fixed by properly deallocating memory using delete
. While stack overflow was left unchanged as an illustrative example, we took care to avoid accessing freed memory by setting pointers to nullptr
after deletion.
Pointer arithmetic errors were resolved by ensuring the pointer stayed within the allocated memory. Finally, we corrected corrupted data structures by accurately adding elements to the vector.
These solutions ensure the stability and reliability of C++ programs, mitigating the risks of segmentation faults and improving overall software quality.
Overall, the program demonstrates various fixes to prevent segmentation faults caused by common issues in C++ programming.
Each fix ensures proper memory access and management, leading to a more robust and reliable program.
Best Practices for Preventing Segmentation Faults in C++
Defensive Programming
Defensive programming involves adopting a proactive approach to prevent segmentation faults by incorporating robust error-checking mechanisms into your code. This includes validating user input, checking for null pointers, and ensuring proper bounds checking for arrays and pointers.
Null Pointer Checks
Before dereferencing any pointer, it’s crucial to check if it’s null. This can be done using an if
statement to ensure that the pointer points to a valid memory location before accessing or modifying its value.
Bounds Checking
When working with arrays or dynamically allocated memory, always verify that the indices or pointer offsets remain within the bounds of the allocated memory. This prevents accessing memory outside the allocated range, which can lead to segmentation faults.
Smart Pointer Usage
Utilize smart pointers such as std::unique_ptr
and std::shared_ptr
instead of raw pointers whenever possible. Smart pointers automatically manage memory allocation and deallocation, reducing the risk of memory leaks and segmentation faults.
Memory Leak Detection
Regularly monitor memory usage and utilize tools like Valgrind
or AddressSanitizer
to detect memory leaks and invalid memory accesses. Fixing memory leaks prevents the accumulation of unused memory and reduces the likelihood of segmentation faults.
Compiler Warnings
Enable compiler warnings and pay attention to warnings related to uninitialized variables, unused variables, and unsafe type conversions. Addressing these warnings helps identify potential sources of segmentation faults early in the development process.
Code Reviews and Testing
Conduct thorough code reviews and testing to identify and address potential segmentation fault issues. Peer reviews can catch errors that may go unnoticed during development, ensuring the reliability and stability of the codebase.
Documentation and Comments
Clearly document your code and include comments to explain the rationale behind certain design decisions or error-handling mechanisms. This helps other developers understand the code and prevents careless mistakes that could lead to segmentation faults.
Continuous Improvement
Stay informed about best practices and evolving techniques for preventing segmentation faults in C++. Continuously improve your coding skills and adopt new methodologies to enhance the reliability and robustness of your codebase.
Conclusion
Fixing segmentation fault errors in C++ is paramount for ensuring the stability and reliability of software. These errors occur when a program tries to access memory it doesn’t have permission to access, leading to crashes or unexpected behavior.
By addressing common causes such as dereferencing null pointers, out-of-bounds array access, uninitialized variables, memory leaks, stack overflow, incorrect memory management, accessing freed memory, pointer arithmetic errors, using deleted objects, and corrupted data structures, developers can mitigate segmentation faults and improve the overall quality of their C++ code.
Understanding these causes and implementing appropriate solutions is essential for creating robust and dependable software applications.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn