How to Retrieve Command-Line Arguments in C++
- Understanding Command-Line Arguments in C++
- Accessing Command-Line Arguments
- Validating Command-Line Arguments
- Conclusion
- FAQ
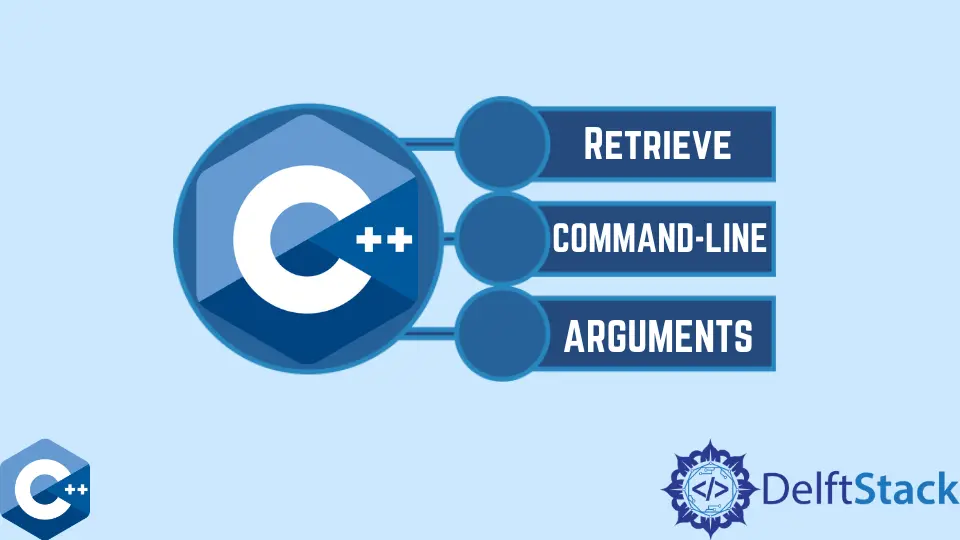
Command-line arguments are an essential feature in programming that allows users to pass information to a program at runtime. In C++, this can be particularly useful for customizing the behavior of applications without altering the source code. Whether you’re building a simple console application or a complex system utility, knowing how to retrieve and utilize command-line arguments can greatly enhance user experience and flexibility.
This article will guide you through the process of retrieving command-line arguments in C++, providing clear explanations and practical code examples to help you get started.
Understanding Command-Line Arguments in C++
In C++, command-line arguments are passed to the main
function, which can accept two parameters: int argc
and char *argv[]
. Here, argc
(argument count) is an integer that represents the number of command-line arguments passed, including the program name itself. The argv
(argument vector) is an array of C-style strings that holds the actual arguments.
Here’s a simple example to illustrate this:
#include <iostream>
int main(int argc, char *argv[]) {
std::cout << "Number of arguments: " << argc << std::endl;
for (int i = 0; i < argc; i++) {
std::cout << "Argument " << i << ": " << argv[i] << std::endl;
}
return 0;
}
When you compile and run this program with command-line arguments, it will display the number of arguments and each argument itself.
Output:
Number of arguments: 3
Argument 0: ./program
Argument 1: arg1
Argument 2: arg2
In this example, if you run the program as ./program arg1 arg2
, argc
will be 3, and argv
will contain the program name and the two arguments. This is a foundational concept in C++ that allows for dynamic input, making your applications more versatile.
Accessing Command-Line Arguments
Once you know how to retrieve command-line arguments, accessing them is straightforward. The argv
array allows you to index into the arguments based on their position. Each argument is treated as a string, so you can manipulate them as needed.
Consider the following example where we check if a specific argument is provided:
#include <iostream>
#include <cstring>
int main(int argc, char *argv[]) {
if (argc > 1 && strcmp(argv[1], "--help") == 0) {
std::cout << "Usage: ./program [options]" << std::endl;
} else {
std::cout << "No help requested." << std::endl;
}
return 0;
}
In this code, we check if the first argument is --help
. If it is, we display a usage message.
Output:
No help requested.
If you run the program with ./program --help
, it will display the usage message. This simple check demonstrates how you can tailor your program’s functionality based on user input, enhancing the overall user experience.
Validating Command-Line Arguments
Validating command-line arguments is crucial to ensure that your program behaves as expected. You can check the number of arguments, their types, or even their ranges. This helps in preventing errors and guiding users to provide the correct inputs.
Here’s an example that validates a numeric argument:
#include <iostream>
#include <cstdlib>
int main(int argc, char *argv[]) {
if (argc != 2) {
std::cout << "Usage: ./program <number>" << std::endl;
return 1;
}
int number = std::atoi(argv[1]);
if (number < 0) {
std::cout << "Please enter a positive number." << std::endl;
} else {
std::cout << "You entered: " << number << std::endl;
}
return 0;
}
In this example, we expect one argument, which should be a number. If the user provides a negative number or no argument at all, the program will display an appropriate message.
Output:
Usage: ./program <number>
When run with a valid argument like ./program 5
, the output will be:
You entered: 5
Validating inputs helps you catch errors early and provides clear feedback to users, making your application more robust.
Conclusion
Retrieving command-line arguments in C++ is a powerful feature that enhances the interactivity and usability of your programs. By understanding how to access, manipulate, and validate these arguments, you can create applications that respond dynamically to user input. Whether you’re building a simple utility or a complex application, mastering command-line arguments will undoubtedly elevate your programming skills. So, go ahead and experiment with these concepts in your next C++ project!
FAQ
-
What are command-line arguments in C++?
Command-line arguments are inputs passed to a C++ program at runtime, allowing users to customize its behavior. -
How do I access command-line arguments in C++?
You can access command-line arguments using theargc
andargv
parameters in themain
function. -
Can I validate command-line arguments?
Yes, you can validate command-line arguments by checking their count and type before processing them. -
What happens if I pass too many arguments?
If you pass more arguments than expected, your program can handle it by checkingargc
and providing an appropriate message. -
Are command-line arguments always strings?
Yes, command-line arguments are passed as strings, but you can convert them to other types as needed.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook