C++ Call Parent Method
- Understanding Class Inheritance in C++
- Using the Scope Resolution Operator
-
Using the
this
Pointer - Overriding Parent Methods
- Using Function Pointers
- Conclusion
- FAQ
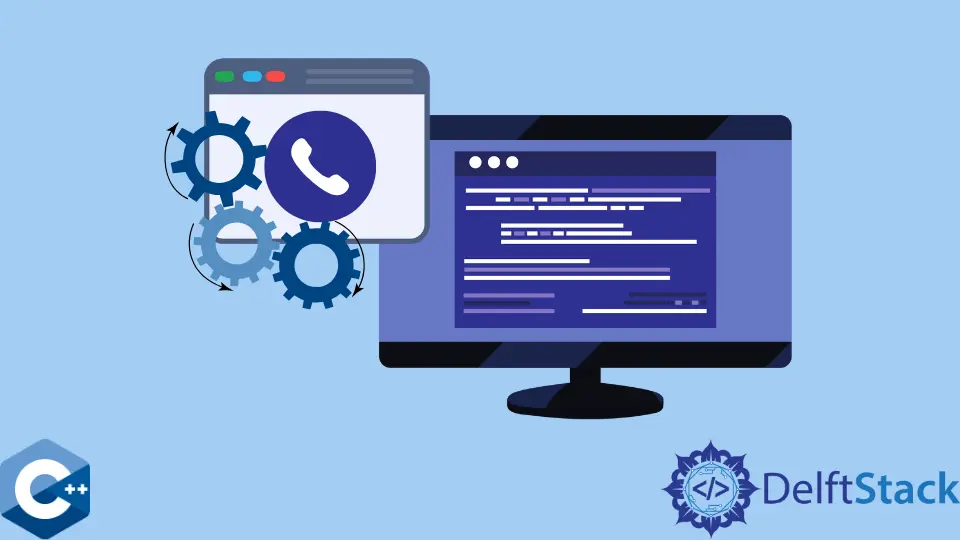
Calling a function in C++ is a fundamental skill that every programmer should master. It involves transferring control to a specific function, allowing you to execute a block of code when needed. However, the process of calling a function is not limited to just one method. In fact, there are multiple ways to achieve this, especially when dealing with class hierarchies and inheritance.
In this article, we’ll explore how to call a parent method in C++, focusing on various approaches and providing clear examples. Whether you are a beginner or an experienced developer, understanding these techniques will enhance your programming toolkit and improve your code organization.
Understanding Class Inheritance in C++
Before diving into the specifics of calling parent methods, let’s briefly cover the concept of class inheritance in C++. Inheritance allows a class to inherit properties and behaviors (methods) from another class, known as the parent or base class. This is a powerful feature that promotes code reuse and establishes a natural hierarchy in your code structure.
When a child class inherits from a parent class, it can access the parent’s public and protected members. However, there may be instances where you want to call a method defined in the parent class from the child class. This is where the ::
operator comes into play.
Using the Scope Resolution Operator
One of the most straightforward ways to call a parent method in C++ is by using the scope resolution operator ::
. This operator allows you to specify the class from which you want to call the method, making it clear that you are invoking a function from the parent class.
Here’s a simple example illustrating this method:
#include <iostream>
using namespace std;
class Parent {
public:
void display() {
cout << "This is the Parent class method." << endl;
}
};
class Child : public Parent {
public:
void callParentMethod() {
Parent::display();
}
};
int main() {
Child child;
child.callParentMethod();
return 0;
}
Output:
This is the Parent class method.
In this example, we have a Parent
class with a method called display()
. The Child
class inherits from Parent
and has its own method called callParentMethod()
. Inside this method, we call the parent’s display()
method using Parent::display()
. This clearly indicates that we are invoking the method from the Parent
class, ensuring that the correct function is executed.
Using the this
Pointer
Another way to call a parent method in C++ is by using the this
pointer. The this
pointer is an implicit pointer that points to the object for which the member function is called. When you want to access a method from the parent class, you can use the this
pointer along with the scope resolution operator.
Here’s how you can utilize this approach:
#include <iostream>
using namespace std;
class Parent {
public:
void display() {
cout << "This is the Parent class method." << endl;
}
};
class Child : public Parent {
public:
void callParentMethod() {
this->display();
}
};
int main() {
Child child;
child.callParentMethod();
return 0;
}
Output:
This is the Parent class method.
In this code snippet, we use this->display()
to call the display()
method from the Parent
class. The this
pointer refers to the current object of the Child
class. This method can be particularly useful when you have overridden a method in the child class and still want to access the parent’s implementation.
Overriding Parent Methods
In some cases, you might want to override a parent method in the child class while still having the option to call the parent’s version of that method. This can be achieved through the use of the override
keyword in C++11 and later.
Here’s an example demonstrating this concept:
#include <iostream>
using namespace std;
class Parent {
public:
virtual void display() {
cout << "This is the Parent class method." << endl;
}
};
class Child : public Parent {
public:
void display() override {
cout << "This is the Child class method." << endl;
Parent::display();
}
};
int main() {
Child child;
child.display();
return 0;
}
Output:
This is the Child class method.
This is the Parent class method.
In this example, the display()
method is overridden in the Child
class. Inside this method, we still call the parent’s display()
method using Parent::display()
. This allows us to extend the functionality of the parent method while still retaining its original behavior.
Using Function Pointers
For more advanced scenarios, you can also use function pointers to call parent methods. This method allows for dynamic method calls and can be particularly useful in callback scenarios or when dealing with arrays of functions.
Here’s a simple implementation:
#include <iostream>
using namespace std;
class Parent {
public:
void display() {
cout << "This is the Parent class method." << endl;
}
};
class Child : public Parent {
public:
void callParentMethod() {
void (Parent::*funcPtr)() = &Parent::display;
(this->*funcPtr)();
}
};
int main() {
Child child;
child.callParentMethod();
return 0;
}
Output:
This is the Parent class method.
In this code, we define a function pointer funcPtr
that points to the display()
method of the Parent
class. We then call this function pointer using (this->*funcPtr)()
, which invokes the parent method. This approach is less common but provides flexibility in certain programming scenarios.
Conclusion
Mastering how to call parent methods in C++ is crucial for effective object-oriented programming. Whether you choose to use the scope resolution operator, the this
pointer, method overriding, or function pointers, each method has its unique advantages. By understanding these techniques, you can write cleaner, more organized code that leverages the power of inheritance. As you continue to explore C++, keep these methods in mind to enhance your programming skills and improve your code structure.
FAQ
-
how do I call a parent method in C++?
You can call a parent method using the scope resolution operator, thethis
pointer, or by overriding the method in the child class. -
what is the scope resolution operator in C++?
The scope resolution operator::
is used to specify the context in which a function or variable is defined, allowing you to call methods from a specific class. -
can I override a parent method in C++?
Yes, you can override a parent method in C++ by defining a method with the same name and signature in the child class. Use theoverride
keyword for clarity. -
what is the purpose of the
this
pointer in C++?
Thethis
pointer is an implicit pointer that refers to the current object for which a member function is called, allowing you to access its members. -
when should I use function pointers in C++?
Function pointers are useful for dynamic method calls, callbacks, and when working with arrays of functions, providing flexibility in your code.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook