How to Add Message to Assert in C++
- Assert in C++
- Advantages of Assert in C++
- Display Custom Message in Assert in C++
- Customize the Custom Message in Assert in C++
- Conclusion
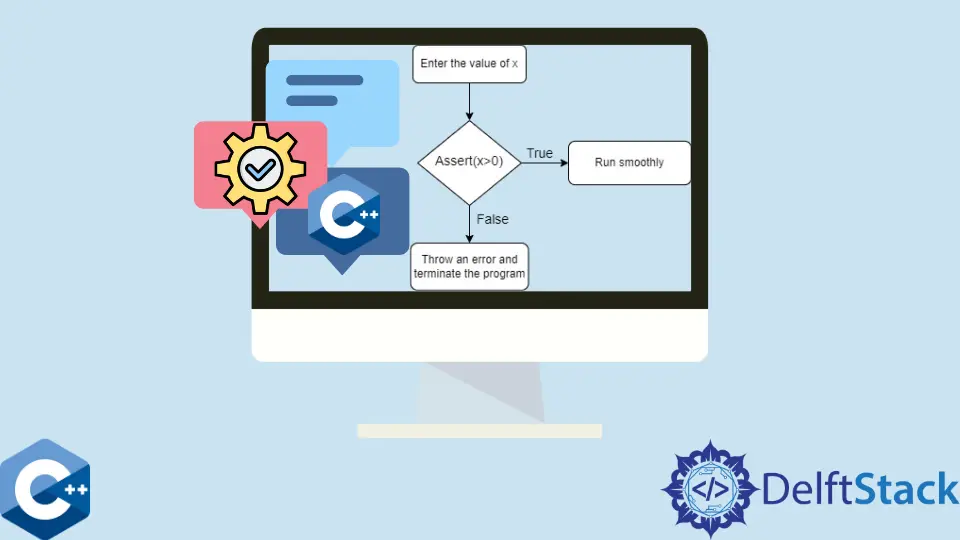
Asserts are one of the errors handling mechanisms in C++ that helps developers and testers debug and test the application.
These are boolean expressions, using the test of the logical expressions in the program and return true
if no error is detected; otherwise, the program is terminated, and an error is displayed.
It is used when we have some constraints that should be satisfied to run properly or otherwise terminated.
Assert in C++
Let’s say the car’s speed should not be less than 0 and greater than the speed of light.
Assert(speed<0 && speed> speed_of_light)
- We have two constraints in this expression that should not be violated.
- Experience has shown that assertions in programming are one of the effective and quickest methods to detect and correct bugs in programs.
- Experience has shown that writing assertions while programming is one of the quickest and most effective ways to detect and correct bugs.
Asserts are preprocessors macros used to evaluate conditionals expression in the programs.
Assert(Condition)
assert(x < 10)
This expression tells us x
should be less than 10
to run smoothly; otherwise, the program will be terminated and throw an error.
For instance, we are running a program with two conditional paths: true
and false
. For the true
path, the program will run smoothly without throwing an error, while in the false
path, the program will be terminated and display an error.
The error typically consists of the failed conditional expression, the path of the program, and the line number where the error occurred.
This helps us get to know the occurrence of the error, which makes testing and debugging more efficient and easy.
Code Example:
#include <cassert>
int main() {
int x = 3;
x = 7;
assert(x == 3);
return 0;
}
Output:
Assertion failed: x==3, file E:\Client Project \Codes\assertCodes.cpp,, line 7
Advantages of Assert in C++
Assertions are very effective and handy error handling mechanisms to help us in many ways to detect errors in the code. It used the test-driven approach, which says we write test cases before development and then start development according to the test cases.
Similarly, we write some piece of code in the assert macro, which the program should pass through it without causing an error.
Some advantages of using assert in testing and debugging.
- Assertions help us in error detection.
- It provides better observability.
- It also helps us identify the cause of the error and the path of error with line number.
- Custom error messages can be added; other testers and programmers can also understand the error more humanized.
- It helps us in the error-free logic building.
- Efficient testing and debugging.
Display Custom Message in Assert in C++
We can also use methods to display custom messages in the assert macro, which is an effective and handy option. It indicated and explained the errors in a more human-friendly manner.
Small teams are working on each part of the software in large software organizations, some are designing, and some are coding and testing. So writing asserts macros with custom messages can be very helpful for the other person to analyze and understand the nature and cause of the error.
Code Example:
#include <cassert>
int main() {
int dividend = 4;
int divisor = 0;
assert(("Message: Anything divided by 0 is undefined", divisor != 0));
int divison = dividend / divisor;
return 0;
}
Output:
Assertion failed: ( "Message: Anything divided by 0 is undefined", divisor != 0), file E:\Client Project \Codes\assertCodes.cpp,, line 6
In this program, we are dividing 4 by 0 (4/0), but as we know, we cannot divide any number by 0, so as expected, this will throw an error. To improve the readability of the error, we have added a custom message.
Customize the Custom Message in Assert in C++
#include <cassert>
#include <iostream>
using namespace std;
bool print_if_false(const bool assertion, const char* message) {
if (!assertion) {
cout << message << endl;
}
return assertion;
}
int main() {
int dividend = 4;
int divisor = 0;
assert(print_if_false(divisor != 0,
"Message: Anything divided by 0 is undefined"));
return 0;
}
Output:
Message: Anything divided by 0 is undefined
Assertion failed: print_if_false(divisor != 0,"Message: Anything divided by 0 is undefined" ), file E:\Client Project \Codes\assertCodes.cpp, line 18
This is the same example as the above, but it is customized. The functionalities are the same overall.
We have created our customized function to display the message on a separate line.
The purpose is to improve readability and know how we can customize assert macros to display custom messages.
Conclusion
In this article, we have understood the assert()
statement in C++, how it works, and the advantages of assert()
. In addition to it, we have seen the syntax of assert()
and how to display customized messages to improve the readability of the error.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn