How to Calculate a Running Median in C++
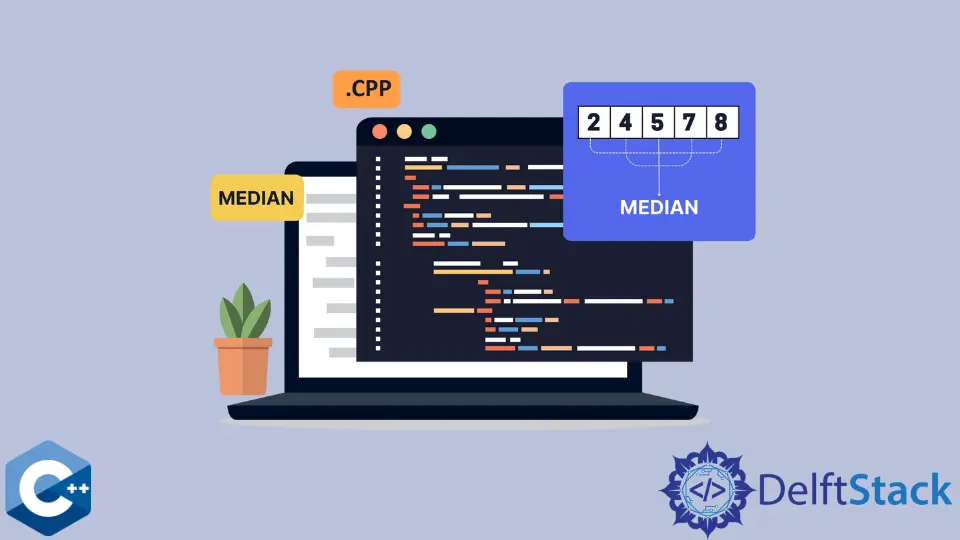
Calculating a running median is a common problem in computer science, especially when dealing with streaming data. The running median can be efficiently computed using two heaps: a max-heap for the lower half of the data and a min-heap for the upper half. This method ensures that all values less than or equal to the current median are stored in the max-heap, while those greater than or equal to the median are kept in the min-heap.
In this article, we will explore how to implement this approach in C++ to achieve optimal performance. Whether you’re working on a data analysis project or developing a real-time application, mastering the running median calculation can significantly enhance your coding skills.
Understanding the Heaps
To calculate the running median, we utilize two heaps: a max-heap and a min-heap. The max-heap stores the lower half of the numbers, allowing us to easily retrieve the maximum value, which represents the current median when combined with the min-heap. The min-heap, on the other hand, holds the upper half of the numbers, allowing us to access the minimum value quickly. This dual-heap structure enables efficient insertion and median retrieval operations, making the entire process efficient.
Implementing the Heaps in C++
Here’s a simple implementation of the running median calculation using C++. In this example, we will define a class that manages the two heaps and provides methods to add new numbers and retrieve the current median.
#include <iostream>
#include <queue>
class RunningMedian {
private:
std::priority_queue<int> maxHeap; // max-heap for the lower half
std::priority_queue<int, std::vector<int>, std::greater<int>> minHeap; // min-heap for the upper half
public:
void addNumber(int num) {
if (maxHeap.empty() || num <= maxHeap.top()) {
maxHeap.push(num);
} else {
minHeap.push(num);
}
// Balance the heaps
if (maxHeap.size() > minHeap.size() + 1) {
minHeap.push(maxHeap.top());
maxHeap.pop();
} else if (minHeap.size() > maxHeap.size()) {
maxHeap.push(minHeap.top());
minHeap.pop();
}
}
double findMedian() {
if (maxHeap.size() > minHeap.size()) {
return maxHeap.top();
}
return (maxHeap.top() + minHeap.top()) / 2.0;
}
};
int main() {
RunningMedian rm;
rm.addNumber(1);
rm.addNumber(5);
rm.addNumber(2);
std::cout << "Current Median: " << rm.findMedian() << std::endl;
return 0;
}
Output:
Current Median: 2
In this implementation, we define a class called RunningMedian
. It has two private members: a max-heap and a min-heap. The addNumber
method adds a new number to the appropriate heap based on its value. After adding, we balance the heaps to maintain their sizes. The findMedian
method retrieves the current median based on the sizes of the heaps. If the max-heap has more elements, the median is the top of the max-heap. If both heaps are equal in size, the median is the average of the tops of both heaps.
Advantages of Using Heaps
Using heaps for calculating the running median offers several advantages. First, both insertion and median retrieval operations are efficient, running in logarithmic time. This efficiency is crucial when dealing with large datasets or real-time streams. Additionally, heaps dynamically adjust their size, which means you don’t have to worry about preallocating storage or resizing arrays. This flexibility makes heaps a robust choice for applications requiring frequent updates and median calculations.
Conclusion
Calculating a running median in C++ using two heaps is an efficient and elegant solution to a common problem in data processing. By maintaining a max-heap and a min-heap, we can quickly insert new numbers and retrieve the current median. This method not only optimizes performance but also simplifies the code structure. Whether you’re working on a small project or a large-scale application, understanding how to implement this technique can significantly enhance your programming skills.
FAQ
-
what is a running median?
A running median is the median of a dataset that updates dynamically as new elements are added. -
why use heaps for calculating the running median?
Heaps allow for efficient insertion and retrieval of the median, making them ideal for handling streaming data. -
can the running median be calculated without heaps?
While it is possible, using heaps is significantly more efficient, especially for large datasets. -
what are the time complexities of adding a number and finding the median?
Both operations have a time complexity of O(log n) due to the heap structure. -
is this method suitable for all types of data?
Yes, as long as the data can be compared, this method can be applied to any numerical dataset.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook