The std::back_inserter Function Template in C++
-
Use
std::back_inserter
to Construct an Iterator That Appends Elements at the End of the Container -
Use
std::back_inserter
Withstd::set_intersection
Algorithm
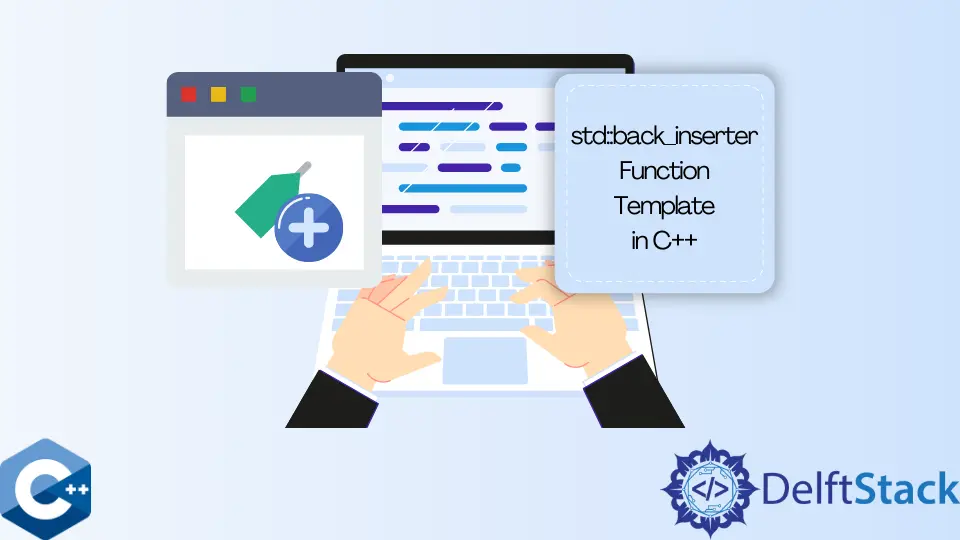
This article will explain how to utilize the std::back_inserter
function template in C++.
Use std::back_inserter
to Construct an Iterator That Appends Elements at the End of the Container
Iterators, generally, provide a common interface to access different container types. In fact, this interface is thoroughly utilized by the STL algorithms. Note, though, each container usually implements a custom behavior that’s suited for the internal data structure.
Additionally, we have a concept of iterator adapters, which provide special functionality on top of the common iterator interface. Namely, insert iterators, which represent iterator adapters, substitute element assignment operation with insertion and let STL algorithms add new elements to the given container rather than overwrite them.
There exist three predefined insert iterators: back inserters, front inserters, and general inserters. In this case, we demonstrate a back inserter to append elements at the end of the container. Notice that back inserters can be applied to the containers that have the push_back
member function.
The following example code shows how to apply the std::fill_n
algorithm to the vector
container using the std::back_inserter
function that automatically constructs a corresponding back inserter iterator.
#include <iostream>
#include <iterator>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::vector;
template <typename T>
void printRange(std::vector<T> v) {
for (const auto &item : v) {
cout << item << "; ";
}
cout << endl;
}
int main() {
vector<int> v1 = {0, 1, 2, 3, 4, 5, 6, 7, 8, 9};
std::fill_n(std::back_inserter(v1), 2, 100);
cout << "v1: ";
printRange(v1);
return EXIT_SUCCESS;
}
Output:
v1: 0; 1; 2; 3; 4; 5; 6; 7; 8; 9; 100; 100;
Use std::back_inserter
With std::set_intersection
Algorithm
Alternatively, we can use the std::back_inserter
with the std::set_intersection
algorithm to store elements from two collections into the destination container without reserving the size in advance. In the next code snippet, we apply the method to the std::vector
container.
#include <algorithm>
#include <iostream>
#include <iterator>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::vector;
template <typename T>
void printRange(std::vector<T> v) {
for (const auto &item : v) {
cout << item << "; ";
}
cout << endl;
}
int main() {
vector<int> v1 = {0, 1, 2, 3, 4, 5, 6, 7, 8, 9};
vector<int> v2 = {1, 2, 3, 4};
vector<int> v3;
std::set_intersection(v1.begin(), v1.end(), v2.begin(), v2.end(),
std::back_inserter(v3));
cout << "v3: ";
printRange(v3);
return EXIT_SUCCESS;
}
Output:
v3: 1; 2; 3; 4;
Additionally, we can use back_inserter
with string
objects as the latter implement the STL container interface. Thus, with the help of the std::set_union
algorithm, we can append the portions of strings to another string object.
#include <algorithm>
#include <iostream>
#include <iterator>
using std::cin;
using std::cout;
using std::endl;
using std::string;
int main() {
string s1("hello");
string s2("there");
string s3;
std::set_union(s1.begin(), s1.end(), s2.begin(), s2.end(),
std::back_inserter(s3));
cout << s3 << endl;
return EXIT_SUCCESS;
}
Output:
hellothere
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook