How to wait Function in C
- Understanding the Wait Function
- Basic Example of the Wait Function
- Using Wait with Multiple Child Processes
- Error Handling with Wait
- Conclusion
- FAQ
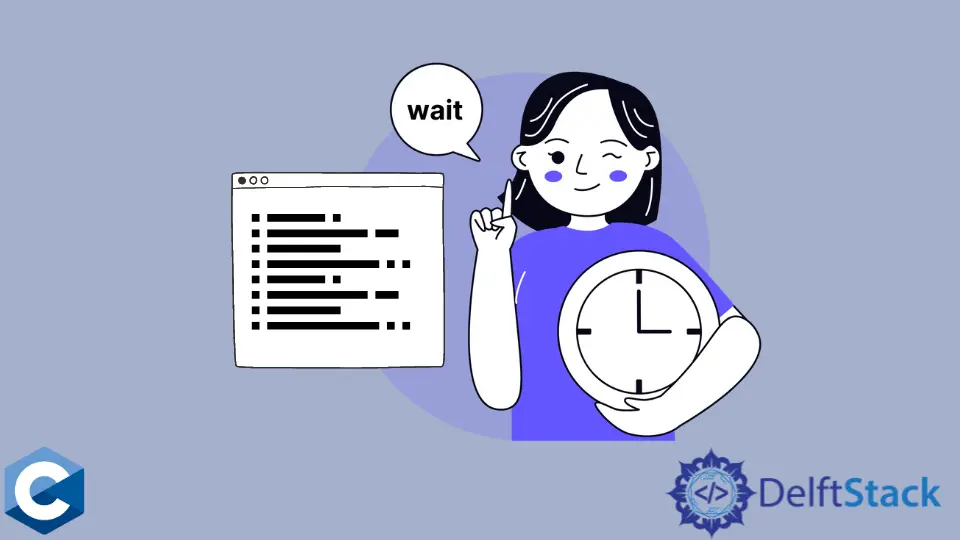
When working with processes in C, managing their execution can sometimes be a challenge. One of the most useful functions for this purpose is the wait
function. This function allows a parent process to wait for its child processes to finish executing, ensuring that resources are properly managed and that data integrity is maintained.
In this article, we will explore how to effectively use the wait
function in C. We will cover its syntax, provide code examples, and explain the underlying concepts to help you grasp its importance in process management. Whether you’re a beginner or looking to refresh your knowledge, this guide will provide valuable insights into using the wait
function in C.
Understanding the Wait Function
The wait
function is part of the POSIX standard and is included in the <sys/types.h>
and <sys/wait.h>
header files. Its primary role is to pause the execution of a parent process until one of its child processes terminates. When a child process ends, the parent can retrieve its termination status, allowing it to handle any necessary cleanup or logging.
The basic syntax of the wait
function is as follows:
pid_t wait(int *status);
Here, status
is a pointer to an integer where the exit status of the child process will be stored. The function returns the process ID of the terminated child, or -1 if an error occurs. This simple yet powerful function is crucial for maintaining orderly execution in multi-process applications.
Basic Example of the Wait Function
To illustrate how the wait
function works, let’s look at a simple example. In this case, we will create a child process using fork()
and then use wait()
to wait for the child to complete.
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/wait.h>
int main() {
pid_t pid = fork();
if (pid < 0) {
perror("Fork failed");
exit(1);
} else if (pid == 0) {
printf("Child process is executing.\n");
sleep(2); // Simulating some work in the child process
exit(0);
} else {
int status;
wait(&status);
if (WIFEXITED(status)) {
printf("Child exited with status: %d\n", WEXITSTATUS(status));
}
}
return 0;
}
Output:
Child process is executing.
Child exited with status: 0
In this example, we start by forking a new process. If the fork()
call is successful, the child process executes a simple message and then sleeps for two seconds to simulate work. Meanwhile, the parent process waits for the child to finish using wait()
. Once the child has exited, the parent retrieves the child’s exit status and prints it. This demonstrates how the wait()
function helps manage process execution effectively.
Using Wait with Multiple Child Processes
In more complex applications, you may need to manage multiple child processes. The waitpid
function allows you to wait for specific child processes, which can be particularly useful when you have multiple children running concurrently.
Here’s an example of how to use waitpid
to wait for multiple child processes:
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/wait.h>
int main() {
pid_t pid1 = fork();
pid_t pid2 = fork();
if (pid1 < 0 || pid2 < 0) {
perror("Fork failed");
exit(1);
}
if (pid1 == 0) {
printf("Child 1 is executing.\n");
sleep(1);
exit(1);
} else if (pid2 == 0) {
printf("Child 2 is executing.\n");
sleep(2);
exit(2);
} else {
int status;
waitpid(pid1, &status, 0);
if (WIFEXITED(status)) {
printf("Child 1 exited with status: %d\n", WEXITSTATUS(status));
}
waitpid(pid2, &status, 0);
if (WIFEXITED(status)) {
printf("Child 2 exited with status: %d\n", WEXITSTATUS(status));
}
}
return 0;
}
Output:
Child 1 is executing.
Child 2 is executing.
Child 1 exited with status: 1
Child 2 exited with status: 2
In this example, we create two child processes. Each child prints a message, sleeps for a different duration, and then exits with a specific status. The parent process uses waitpid()
to wait for each child process to terminate individually. This way, you can manage multiple child processes and handle their exit statuses accordingly.
Error Handling with Wait
Proper error handling is essential when using the wait
function. If you do not handle errors correctly, it can lead to undefined behavior in your program. The wait
function will return -1 if there are no child processes to wait for or if an error occurs. Therefore, it’s important to check the return value and handle errors appropriately.
Here’s a modified version of the previous example that includes error handling:
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/wait.h>
int main() {
pid_t pid = fork();
if (pid < 0) {
perror("Fork failed");
exit(1);
} else if (pid == 0) {
printf("Child process is executing.\n");
sleep(2);
exit(0);
} else {
int status;
pid_t result = wait(&status);
if (result == -1) {
perror("Wait failed");
exit(1);
}
if (WIFEXITED(status)) {
printf("Child exited with status: %d\n", WEXITSTATUS(status));
}
}
return 0;
}
Output:
Child process is executing.
Child exited with status: 0
In this example, we check the return value of wait()
. If it returns -1, we print an error message and exit the program. This ensures that any issues with waiting for the child process are handled gracefully. Proper error handling is crucial for creating robust and reliable applications.
Conclusion
The wait
function in C is a powerful tool for managing child processes. By using wait
or waitpid
, you can ensure that your parent process waits for its children to finish executing, allowing for proper resource management and data integrity. Whether you’re working on simple applications or more complex multi-process systems, understanding how to effectively use the wait
function will enhance your programming skills. With the examples and explanations provided in this article, you should feel more confident in implementing the wait
function in your own C programs.
FAQ
-
What is the purpose of the wait function in C?
The wait function allows a parent process to wait for its child processes to terminate, ensuring proper resource management. -
Can I use wait with multiple child processes?
Yes, you can use wait or waitpid to manage multiple child processes and wait for them to finish individually. -
What happens if a child process exits before the parent calls wait?
If a child process exits before the parent calls wait, the parent will still be able to retrieve its exit status when it calls wait. -
How do I handle errors when using wait?
Always check the return value of wait. If it returns -1, an error occurred, and you should handle it appropriately. -
Is wait part of the C standard library?
No, wait is part of the POSIX standard and is included in specific header files like <sys/types.h> and <sys/wait.h>.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook