How to Create a Table in C
- Understanding Arrays in C
- Creating a One-Dimensional Table
- Creating a Two-Dimensional Table
- Using Functions to Handle Tables
- Conclusion
- FAQ
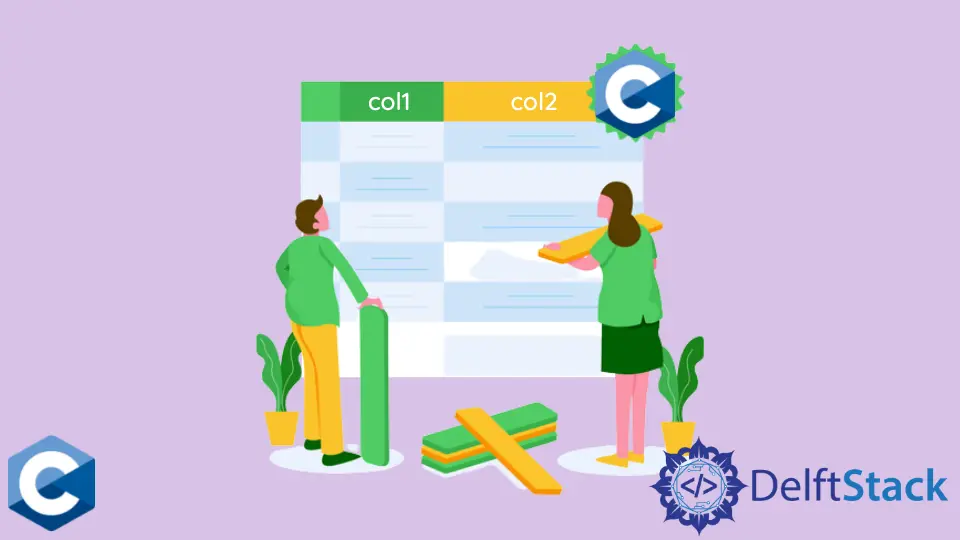
Creating tables in C can seem like a daunting task, especially for beginners. However, it’s actually quite straightforward once you understand the concept of arrays. In C, an array can be thought of as a table that holds multiple values of the same type.
This article will guide you through the steps to create tables using arrays in C, providing clear examples and explanations along the way. By the end, you’ll have a solid grasp of how to effectively use arrays to create tables, making your C programming experience more enriching and productive. Let’s dive in!
Understanding Arrays in C
Before we jump into creating tables, it’s essential to understand what arrays are. An array in C is a collection of variables that are accessed with a common name. The size of an array must be defined at the time of declaration, and it can hold multiple values of the same data type.
To declare an array in C, you can use the following syntax:
data_type array_name[array_size];
For example, if you want to create an array of integers to hold five values, you would declare it like this:
int numbers[5];
This creates a table-like structure where you can store up to five integers. You can access each element using an index, starting from zero. So, numbers[0]
refers to the first element, numbers[1]
to the second, and so on.
Creating a One-Dimensional Table
One-dimensional arrays are the simplest form of tables in C. They allow you to store data in a single row. Here’s how to create a one-dimensional table:
#include <stdio.h>
int main() {
int table[5] = {10, 20, 30, 40, 50};
for (int i = 0; i < 5; i++) {
printf("Element at index %d: %d\n", i, table[i]);
}
return 0;
}
Output:
Element at index 0: 10
Element at index 1: 20
Element at index 2: 30
Element at index 3: 40
Element at index 4: 50
In this example, we declare an array called table
that holds five integers. We initialize it with values from 10 to 50. The for
loop then iterates through the array, printing each element along with its index. This simple structure is the foundation for creating more complex tables.
Creating a Two-Dimensional Table
For more complex data, you might need a two-dimensional table, often referred to as a matrix. This allows you to store data in rows and columns. Here’s how to create a two-dimensional table in C:
#include <stdio.h>
int main() {
int table[3][3] = {
{1, 2, 3},
{4, 5, 6},
{7, 8, 9}
};
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
printf("%d ", table[i][j]);
}
printf("\n");
}
return 0;
}
Output:
1 2 3
4 5 6
7 8 9
In this example, we declare a two-dimensional array called table
with three rows and three columns. Each element is initialized to create a small matrix. The nested for
loops allow us to access each element using two indices: the first for the row and the second for the column. The output displays the matrix format, making it easier to visualize the data structure.
Using Functions to Handle Tables
To make your code cleaner and more modular, you can create functions to handle tables. This is especially useful for larger tables or when performing multiple operations. Here’s an example:
#include <stdio.h>
void printTable(int rows, int cols, int table[rows][cols]) {
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
printf("%d ", table[i][j]);
}
printf("\n");
}
}
int main() {
int table[2][4] = {
{1, 2, 3, 4},
{5, 6, 7, 8}
};
printTable(2, 4, table);
return 0;
}
Output:
1 2 3 4
5 6 7 8
Here, we define a function called printTable
that takes the number of rows, columns, and the table itself as arguments. This encapsulates the logic for printing the table, making the main
function cleaner and easier to understand. This approach also promotes code reuse, allowing you to call printTable
with different tables without rewriting the print logic.
Conclusion
Creating tables in C using arrays is a fundamental skill that can significantly enhance your programming capabilities. Whether you’re working with one-dimensional or two-dimensional arrays, understanding how to manipulate these structures opens up a world of possibilities for data organization and management. By using functions, you can streamline your code, making it more efficient and easier to maintain. With these techniques under your belt, you’re well on your way to mastering data structures in C. Keep practicing, and soon you’ll find yourself creating complex programs with ease!
FAQ
- What is an array in C?
An array in C is a collection of variables that are accessed with a common name and can hold multiple values of the same data type.
-
How do I declare a two-dimensional array in C?
You can declare a two-dimensional array using the syntaxdata_type array_name[rows][columns];
. -
Can I create a table with more than two dimensions in C?
Yes, C allows you to create multi-dimensional arrays, but they can become complex and harder to manage. -
What are the advantages of using functions with arrays?
Functions help in organizing code, making it more readable and reusable, which is especially useful for larger projects. -
How do I access elements in a two-dimensional array?
You can access elements using two indices, likearray_name[row_index][column_index]
.