How to Use the nanosleep Function in C
- Understanding the nanosleep Function
- Example of Using nanosleep
- Handling Interrupted Sleeps
- Using nanosleep in a Loop
- Conclusion
- FAQ
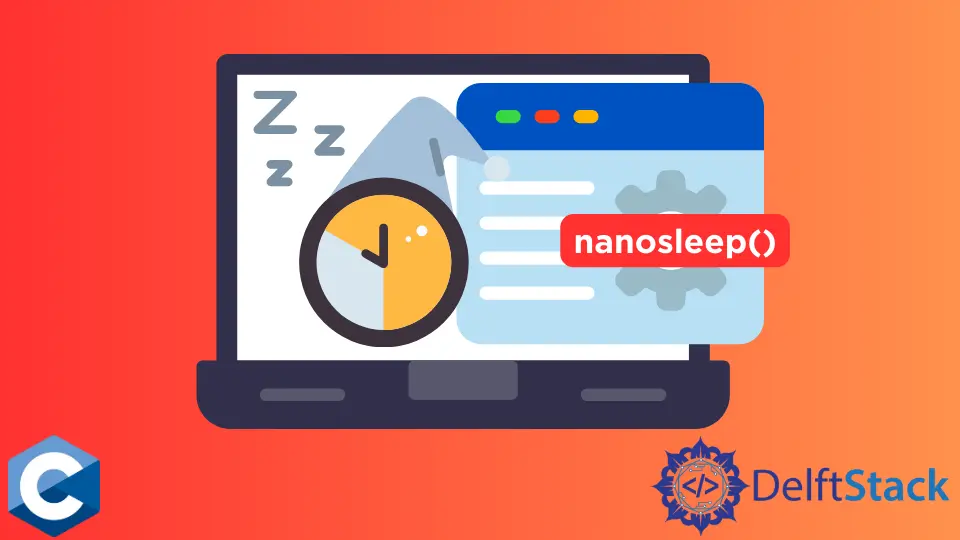
In the world of programming, timing can be crucial, especially when it comes to executing tasks at precise intervals. The nanosleep
function in C provides a way to pause the execution of a program for a specified amount of time, measured in seconds and nanoseconds. This function is particularly useful in scenarios where you need to control the timing of events, such as in real-time systems, simulations, or even simple applications that require a delay.
In this article, we will explore how to effectively use the nanosleep
function in C, providing clear examples and explanations to ensure you can implement it seamlessly in your projects.
Understanding the nanosleep Function
The nanosleep
function is part of the <time.h>
library in C. It allows you to suspend the execution of the calling thread for a specified duration, which can be defined in seconds and nanoseconds. The function prototype looks like this:
#include <time.h>
int nanosleep(const struct timespec *req, struct timespec *rem);
req
: This is a pointer to atimespec
structure that specifies the desired sleep duration.rem
: This is a pointer to atimespec
structure that, if the sleep is interrupted, will contain the remaining time.
The function returns 0
on success and -1
on error, with errno
set to indicate the error.
Example of Using nanosleep
Let’s dive into a simple example to illustrate how to use the nanosleep
function. In this example, we will create a program that pauses the execution for two seconds.
#include <stdio.h>
#include <time.h>
int main() {
struct timespec req, rem;
req.tv_sec = 2; // seconds
req.tv_nsec = 0; // nanoseconds
printf("Sleeping for 2 seconds...\n");
nanosleep(&req, &rem);
printf("Awake now!\n");
return 0;
}
When you run this program, it will display a message indicating that it is sleeping for two seconds, then pause the execution, and finally print a message when it resumes.
Output:
Sleeping for 2 seconds...
Awake now!
In this code, we first include the necessary header files. The timespec
structure is initialized with a sleep duration of two seconds. The nanosleep
function is then called, which effectively pauses the program for the specified time. It’s important to note that if the sleep is interrupted by a signal, the remaining time will be stored in the rem
variable.
Handling Interrupted Sleeps
In some cases, your program may be interrupted during the sleep period, such as when a signal is received. To handle this situation gracefully, you can check the rem
variable to see how much time is left and decide whether to continue sleeping or handle the interruption. Here’s an example:
#include <stdio.h>
#include <time.h>
#include <signal.h>
void handle_signal(int signal) {
printf("Signal %d received. Resuming...\n", signal);
}
int main() {
struct timespec req, rem;
req.tv_sec = 5; // seconds
req.tv_nsec = 0; // nanoseconds
signal(SIGINT, handle_signal);
printf("Sleeping for 5 seconds...\n");
if (nanosleep(&req, &rem) == -1) {
printf("Sleep interrupted. Remaining time: %ld seconds and %ld nanoseconds\n", rem.tv_sec, rem.tv_nsec);
}
printf("Awake now!\n");
return 0;
}
When you run this program and send an interrupt signal (like pressing Ctrl+C), it will print a message indicating that the sleep was interrupted and show the remaining time.
Output:
Sleeping for 5 seconds...
^C
Signal 2 received. Resuming...
Sleep interrupted. Remaining time: 0 seconds and 0 nanoseconds
Awake now!
In this example, we set up a signal handler for SIGINT
to catch interrupt signals. If the sleep is interrupted, the program will print the remaining time, allowing you to take appropriate action.
Using nanosleep in a Loop
Another common use case for nanosleep
is within a loop where you want to perform an action at regular intervals. For instance, you might want to print a message every second for a total of five seconds. Here’s how you can achieve that:
#include <stdio.h>
#include <time.h>
int main() {
struct timespec req;
req.tv_sec = 1; // seconds
req.tv_nsec = 0; // nanoseconds
for (int i = 0; i < 5; i++) {
printf("Message %d\n", i + 1);
nanosleep(&req, NULL);
}
return 0;
}
This program will print a message every second for five seconds.
Output:
Message 1
Message 2
Message 3
Message 4
Message 5
In this loop, we call nanosleep
after printing each message, which effectively creates a delay between the outputs. This is useful for creating timed events or animations in terminal applications.
Conclusion
The nanosleep
function in C is a powerful tool for managing time in your programs. Whether you need to pause execution for a specific duration, handle interruptions, or create timed loops, this function provides the flexibility you need. By understanding how to use nanosleep
, you can enhance the functionality of your applications, ensuring they behave as expected in time-sensitive scenarios. Experiment with the examples provided, and soon you’ll be using nanosleep
like a pro!
FAQ
-
what is the purpose of the nanosleep function?
The nanosleep function is used to suspend the execution of a program for a specified time, measured in seconds and nanoseconds. -
can nanosleep be interrupted?
Yes, nanosleep can be interrupted by signals, and if interrupted, it will return -1 and set a remaining time in the rem variable. -
how do I include the nanosleep function in my C program?
You need to include the<time.h>
header file in your C program to use the nanosleep function. -
what happens if I pass a negative value to nanosleep?
Passing a negative value to nanosleep is not valid and will likely result in an error. -
can I use nanosleep for precise timing in real-time systems?
Yes, nanosleep can be used for precise timing, but for high-resolution timing needs, other methods may be more appropriate.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook