How to Use the goto Statement in C
-
Use the
goto
Statement to Implement a Loop in C -
Use the
goto
Statement to Get Out of Nested Loops in C
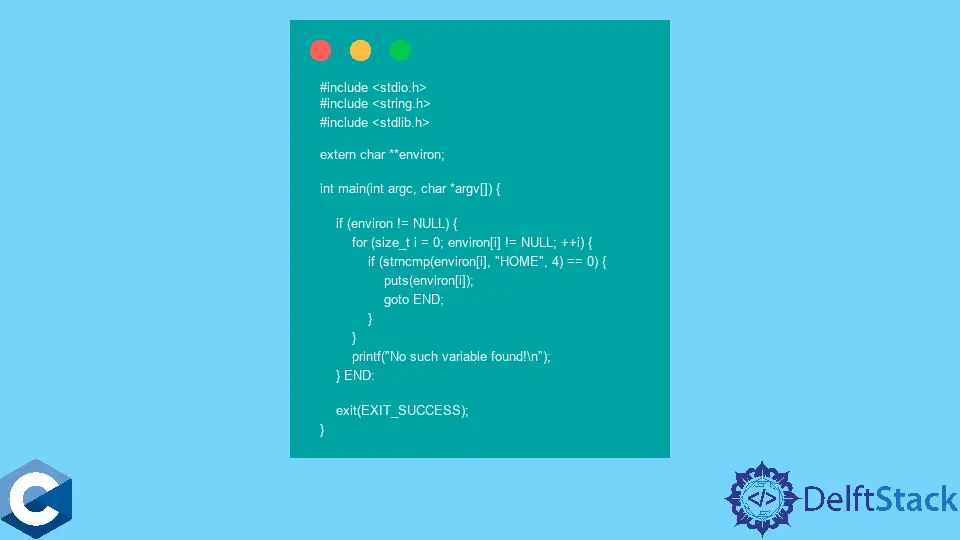
This article will demonstrate multiple methods about how to use the goto
statement in C.
Use the goto
Statement to Implement a Loop in C
The goto
keyword is part of the C language, and it provides a construct to do an unconditional jump. if
and switch
statements are examples of conditional jumps. goto
construct consists of two parts: goto
call and label name. Any statement in code can be preceded by a label, which is just an identifier followed by a colon. goto
call forces the code execution to jump to the first statement after the label. goto
can only jump to the label inside the same function and labels are visible in the entire function regardless on which line they are defined.
In the following example, we demonstrate a simple loop that compares the variable score
to 1000
on each cycle. If score
is less than equal, it increments and jumps to the comparison statement again. Once the if
statement is true, another goto
call is invoked, and execution jumps to the EXIT
label, leading to the program’s normal termination. This example code, similar to many others, can be reimplemented without goto
statements, making them relatively easy to read. Generally, there has been a vigorous debate about the goto
statement, some consider it utterly harmful to readable code, and others still see some practical use cases for it.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
extern char **environ;
int main(int argc, char *argv[]) {
int score = 1;
START:
if (score > 1000) goto EXIT;
score += 1;
goto START;
EXIT:
printf("score: %d\n", score);
exit(EXIT_SUCCESS);
}
Output:
score: 1001
Use the goto
Statement to Get Out of Nested Loops in C
The goto
statement can be useful to change control flow if the conditional statement inside a loop is satisfied, and some code should be skipped as well. The following code sample demonstrates a similar scenario, where the environment variable array is accessed and searched. Notice that the outer if
statement checks if the pointer is valid and only then proceeds to execute the loop. The loop itself has another conditional inside it, which checks for each environment variable’s specific string. If the string is found, we can break out of the loop, not waste any more processing resources, and skip the following printf
statement. This creates a useful case for the goto
call to be included inside the inner if statement that would make the program jump outside of the outer if
statement, continuing to execute the rest of the code.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
extern char **environ;
int main(int argc, char *argv[]) {
if (environ != NULL) {
for (size_t i = 0; environ[i] != NULL; ++i) {
if (strncmp(environ[i], "HOME", 4) == 0) {
puts(environ[i]);
goto END;
}
}
printf("No such variable found!\n");
}
END:
exit(EXIT_SUCCESS);
}
Output:
HOME=/home/username
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook