How to Get Current Working Directory in C
-
Use the
getcwd
Function to Get Current Working Directory -
Properly Verify the Value Returned From the
getcwd
Function to Get Current Working Directory in C -
Use the
get_current_dir_name
Function to Get Current Working Directory
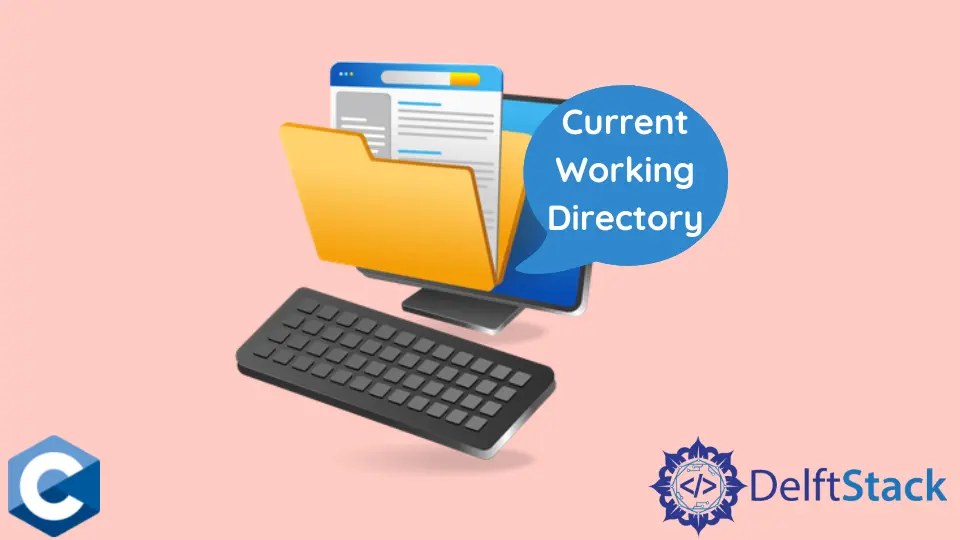
This article will explain several methods of how to get the current working directory in C.
Use the getcwd
Function to Get Current Working Directory
The getcwd
function is a POSIX compliant system call that can retrieve the current working directory of the calling program. getcwd
takes two arguments - char*
buffer where the pathname is stored and the number of bytes allocated in the given buffer. The function stores the name of the current working directory as a null-terminated string and the user is responsible for guaranteeing that the char*
address has enough space for the pathname. Note that getcwd
returns the absolute pathname of the directory.
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#ifndef MAX_BUF
#define MAX_BUF 200
#endif
int main(void) {
char path[MAX_BUF];
getcwd(path, MAX_BUF);
printf("Current working directory: %s\n", path);
exit(EXIT_SUCCESS);
}
Properly Verify the Value Returned From the getcwd
Function to Get Current Working Directory in C
The getcwd
function can fail to retrieve the correct value; thus, in such cases, it returns NULL
and sets errno
with the corresponding error codes. The user is responsible for implementing error-checking code and modifying the program’s control flow as needed for a given scenario. Note that we set errno
explicitly to zero before the getcwd
function call as it’s best practice to do so before calling to the library functions that promise to set errno
. errno
is set to ERANGE
if the size argument is less than pathname of the working directory.
#include <errno.h>
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#ifndef MAX_BUF
#define MAX_BUF 200
#endif
int main(void) {
char path[MAX_BUF];
errno = 0;
if (getcwd(path, MAX_BUF) == NULL) {
if (errno == ERANGE)
printf("[ERROR] pathname length exceeds the buffer size\n");
else
perror("getcwd");
exit(EXIT_FAILURE);
}
printf("Current working directory: %s\n", path);
exit(EXIT_SUCCESS);
}
Alternatively, we can pass the NULL
value as the first argument to getcwd
function and 0
as the second argument. The function itself will allocate a big enough buffer dynamically using malloc
and return the char
pointer to the location. Notice that the user should call the free
function on the returned pointer to deallocate the buffer memory.
#include <errno.h>
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#ifndef MAX_BUF
#define MAX_BUF 200
#endif
int main(void) {
char path[MAX_BUF];
errno = 0;
char *buf = getcwd(NULL, 0);
if (buf == NULL) {
perror("getcwd");
exit(EXIT_FAILURE);
}
printf("Current working directory: %s\n", buf);
free(buf);
exit(EXIT_SUCCESS);
}
Use the get_current_dir_name
Function to Get Current Working Directory
get_current_dir_name
is another function that can retrieve the current working directory. Note that this function requires _GNU_SOURCE
macro to be defined; on the contrary, the code will likely throw a compilation error. get_current_dir_name
takes no arguments and returns char
pointer similar to the getcwd
function. The memory is allocated automatically using malloc
, and the caller code is responsible for freeing the returned pointer.
#define _GNU_SOURCE
#include <errno.h>
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#ifndef MAX_BUF
#define MAX_BUF 200
#endif
int main(void) {
char path[MAX_BUF];
errno = 0;
char *buf = get_current_dir_name();
if (buf == NULL) {
perror("get_current_dir_name");
exit(EXIT_FAILURE);
}
printf("Current working directory: %s\n", buf);
free(buf);
exit(EXIT_SUCCESS);
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook