The Forward Declaration and Difference Between Struct and Typedef Struct in C
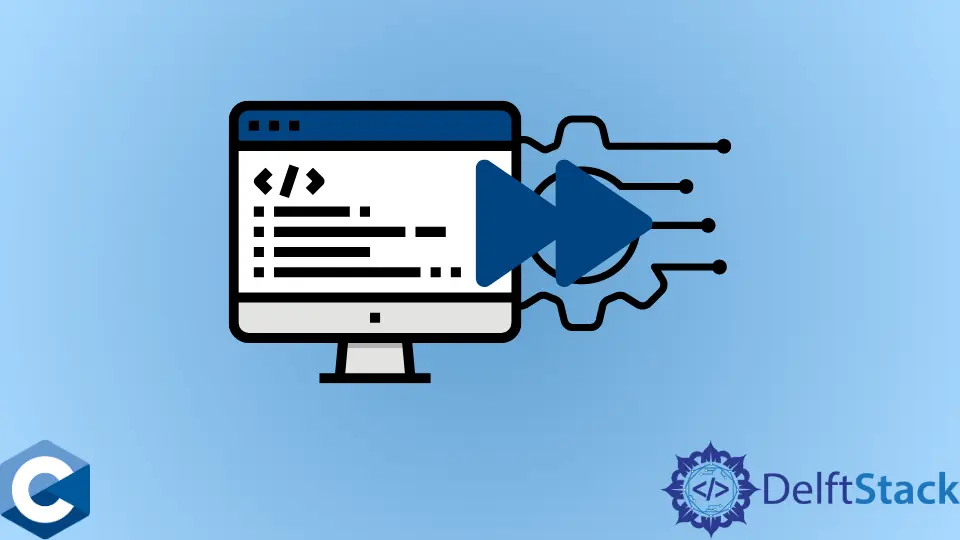
This tutorial will explain the concept of struct
and the use of the typedef
keyword. We will also see the concept of the forward declaration
in C.
Let’s start by creating a C project.
Create a C project
-
The first step is to install a compiler. Steps to download and install the C compiler.
-
In the next step, create an empty project in C language by clicking on the
File
in the menu bar. -
Save the file before compiling.
-
Execute the code. By clicking on
compile and run
. -
An execution screen will appear.
Struct in C Language
Struct is used in C language to make the related data members
group. The members in the struct don’t have the same datatype, and the group contains different variables kept in one place.
As we know, that array has the same type of members, but in a struct, the data members can be of different types like int
, float
, and char
. The following code snippet will describe how to make a struct in C language.
In this code, we have declared the variable in the local scope
in the main function
by writing the struct name
and its variable
.
Code Example:
#include <stdio.h>
struct Student { // Structure declaration
int rollno; // Member (int variable)
char gender; // Member (char variable)
}; // End the structure with a semicolon
int main() {
struct Student s1; // Making a variable of a struct
s1.rollno = 3; // assigning value to every member using dot operator
s1.gender = 'M';
printf("The name and gender of a student is %d and %c", s1.rollno, s1.gender);
}
Output:
The name and gender of a student is 3 and M
We will declare the variable in the global scope in the following code.
Code Example:
#include <stdio.h>
struct Student { // Structure declaration
int rollno; // Member (int variable)
char gender; // Member (char variable)
} s1; // Declared a variable s1 before ending the struct with a semicolon
int main() {
s1.rollno = 3; // calling a member using a variable
s1.gender = 'M';
printf("The name and gender of a student is %d and %c", s1.rollno, s1.gender);
}
Output:
The name and gender of a student is 3 and M
Again this will give the same output, but in the above code snippet, we have declared the variable s1
globally. We don’t have to define a struct in the main()
function.
typedef
in C
typedef
is used in the C language to rename our existing datatype with a new one.
Syntax:
typedef<existing_name><alias_name>
First, we have to write the reserved word of typedef
, then the existing name in C language, and the name we want to assign. After using typedef
, we will use the alias_name
in the whole program.
Code:
#include <stdio.h>
void main() {
typedef int integer; // assigning int a new name of the integer
integer a = 3; // variable is declared by integer
integer b = 4;
printf("The values of a and b are %d and %d", a,
b); // print value of a and b on the screen
}
Output:
The values of a and b are 3 and 4
typedef
Struct in C
We have seen that we must write the whole struct definition in the main()
function. Instead of writing struct student
every time, we can replace the old type using typedef with a new type.
Typedef
helps us to create our type in C language.
Code Example:
#include <stdio.h> // including header file of input/output
#include <string.h> // including header file of string
typedef struct Books { // old type
char title[30]; // data members of the struct book
char author[30];
char subject[50];
int id;
} Book; // new type
void main() {
Book b1; // variable of a new type
strcpy(b1.title, "C Programming"); // copy string in title
strcpy(b1.author, "Robert Lafore"); // copy string in author
strcpy(b1.subject, "Typedef Struct in C"); // copy string in subject
b1.id = 564555; // assigning id to variable
printf("Book title is : %s\n", b1.title); // printing book title on screen
printf("Book author is : %s\n", b1.author);
printf("Book subject is : %s\n", b1.subject);
printf("Book id is : %d\n", b1.id);
}
Output:
Book title is : C Programming
Book author is : Robert Lafore
Book subject is : Typedef Struct in C
Book id is : 564555
In the above code, we’ve defined a new struct type at the end of the struct before the semicolon. Then, we used that new type in the main()
function.
This typedef
has reduced the effort of defining struct every time in the main()
function to make a variable.
Forward Declaration in C
The forward declaration is a declaration that precedes an actual definition of a Struct. The definition is unavailable, but we can reference the declared type due to the forward declaration, which is a beforehand declaration.
This method is used to define and declare a function. Instead of defining a function on top of the main()
function, we can declare it on the top and define it at the bottom, called forward declaration.
Code Example:
#include <stdio.h>
int add(int x, int y); // (prototype)function declaration
void main() {
int n1, n2, sum;
scanf("%d %d", &n1, &n2); // taking numbers from user
sum = add(n1, n2); // call to the add function
printf("The sum of n1 and n2 is %d ", sum); // display sum on the screen
}
int add(int x, int y) // function definition
{
int result;
result = x + y;
return result; // returning the result to the main function
}
The code snippet above shows that we’ve declared a function prototype at the top of the main()
function. We call the add()
function in the main before defining it, but it works properly because the prototype has already been defined.
This is due to forward declaration.
Output:
4 5
The sum of n1 and n2 is 9