The extern Keyword in C
-
What is the
extern
Keyword? -
Using
extern
with Variables -
Using
extern
with Functions - Best Practices for Using Extern
- Conclusion
- FAQ
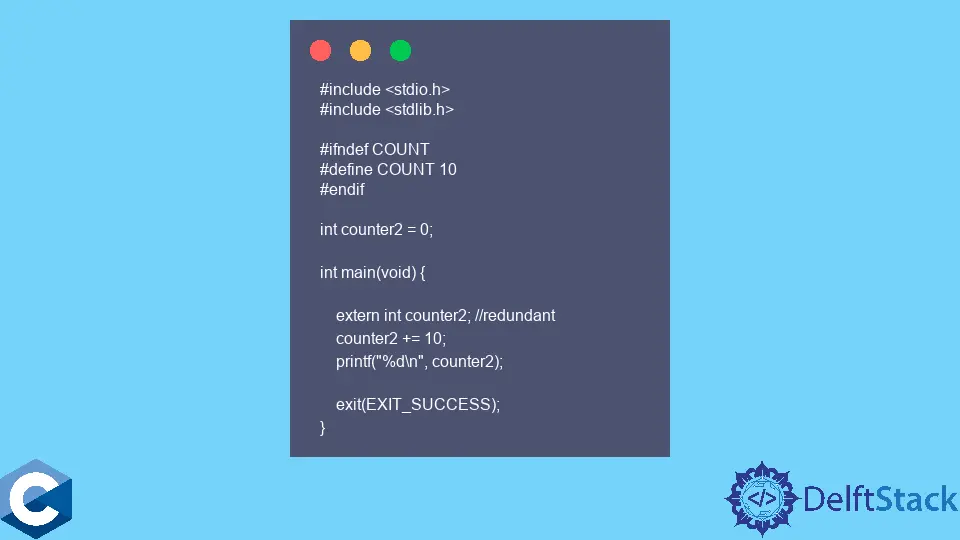
Understanding the extern
keyword in C is crucial for any programmer looking to manage variable scope effectively. This keyword plays a significant role in linking and sharing variables across multiple files, making it easier to organize and maintain large codebases.
In this article, we will delve into the ins and outs of the extern
keyword, providing you with practical examples and explanations to enhance your programming skills. By the end of this guide, you will have a clear understanding of how to use extern
in your C programs, allowing for better modularization and code reuse. Let’s dive in!
What is the extern
Keyword?
The extern
keyword in C is primarily used to declare a variable or function that is defined in another file or translation unit. This allows different files to share the same variable without creating multiple instances. By using extern
, you can manage the scope of your variables efficiently, ensuring that they are accessible wherever needed.
For instance, if you have a variable defined in one file and you want to use it in another, you can declare it with the extern
keyword. This tells the compiler that the variable exists, but its memory allocation will occur elsewhere. This is particularly useful in large projects where multiple source files are involved.
Using extern
with Variables
To illustrate how to use the extern
keyword with variables, let’s consider a simple example involving two files: file1.c
and file2.c
. In file1.c
, we will define a variable, while in file2.c
, we will declare it using extern
.
file1.c
#include <stdio.h>
int globalVar = 10;
void display() {
printf("Global Variable: %d\n", globalVar);
}
file2.c
#include <stdio.h>
extern int globalVar;
int main() {
printf("Accessing Global Variable from another file: %d\n", globalVar);
return 0;
}
When you compile and run these files together, you will see how file2.c
accesses the variable defined in file1.c
.
Output:
Accessing Global Variable from another file: 10
In this example, globalVar
is defined in file1.c
and declared in file2.c
using extern
. This allows file2.c
to access the variable without creating a new instance, demonstrating the power of the extern
keyword in managing variable scope across multiple files.
Using extern
with Functions
In addition to variables, the extern
keyword can also be used with functions. This allows you to define a function in one file and call it from another. Let’s see how this works with the same two files.
file1.c
#include <stdio.h>
void greet() {
printf("Hello from file1.c!\n");
}
file2.c
#include <stdio.h>
extern void greet();
int main() {
greet();
return 0;
}
When you compile and run these files together, you will see that file2.c
successfully calls the greet
function defined in file1.c
.
Output:
Hello from file1.c!
In this case, the greet
function is declared in file2.c
using the extern
keyword, allowing it to access the function defined in file1.c
. This illustrates how extern
facilitates function sharing across different files, promoting modular programming practices.
Best Practices for Using Extern
While the extern
keyword is powerful, there are some best practices to keep in mind to avoid common pitfalls:
- Limit Scope: Use
extern
sparingly to avoid creating dependencies between files. Keep your variables and functions as local as possible. - Consistent Naming: Maintain a consistent naming convention for your variables to avoid confusion. This is especially important in larger projects.
- Documentation: Document the purpose of
extern
declarations to help other developers understand the code better. - Use Header Files: Consider using header files for
extern
declarations. This keeps your code organized and allows for easier maintenance.
By following these best practices, you can effectively manage variable and function scope in your C programs, leading to cleaner and more maintainable code.
Conclusion
The extern
keyword is an essential tool in C programming that enables the sharing of variables and functions across multiple files. By understanding and effectively using extern
, you can enhance the modularity and organization of your code, making it easier to manage and maintain. Whether you are working on a small project or a large codebase, mastering the extern
keyword will undoubtedly improve your programming skills. So, go ahead, experiment with extern
, and see how it can transform your approach to coding in C!
FAQ
-
What does the
extern
keyword do in C?
Theextern
keyword is used to declare a variable or function that is defined in another file, allowing for sharing across multiple files. -
Can I use
extern
for local variables?
No,extern
is intended for global variables and functions. Local variables cannot be shared across files. -
How do I declare an
extern
variable?
You declare anextern
variable by specifying the keyword followed by the variable type and name, e.g.,extern int myVar;
. -
Is it necessary to initialize an
extern
variable?
No,extern
variables should not be initialized at the point of declaration. They should be defined elsewhere. -
Can I use
extern
with functions in C?
Yes, you can useextern
with functions to declare them in one file while defining them in another.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook