The execvp Function in C
- What is execvp?
- How to Use execvp
- Error Handling with execvp
- Combining execvp with Fork
- Conclusion
- FAQ
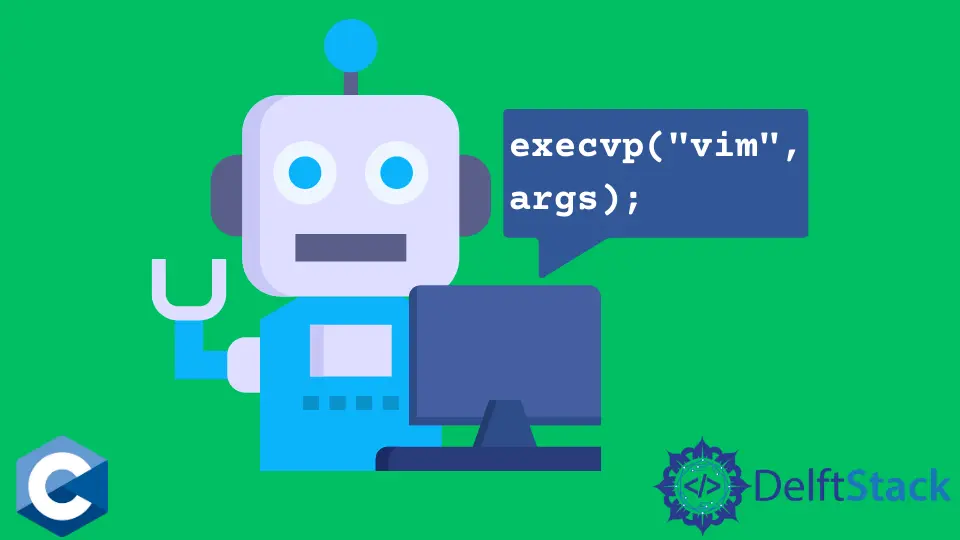
The execvp
function in C is a powerful tool for executing programs within a running process. It is part of the exec family of functions and is essential for creating new processes in Unix-like operating systems. Understanding how to use execvp
effectively can enhance your programming skills and allow you to create more dynamic applications.
This article will guide you through the ins and outs of the execvp
function, providing clear examples and explanations. Whether you’re a beginner or an experienced developer, this guide will help you harness the full potential of execvp
in your C projects.
What is execvp?
The execvp
function is used to execute a file, replacing the current process image with a new process image. It is defined in the unistd.h
header file and takes two parameters: the filename of the program to be executed and an array of arguments. The execvp
function searches for the executable in the directories listed in the environment variable PATH
, making it convenient for executing programs without needing to specify their full paths.
The function signature looks like this:
int execvp(const char *file, char *const argv[]);
- file: The name of the executable file.
- argv: An array of argument strings passed to the new program. The first element should be the name of the program itself, and the array must be terminated with a NULL pointer.
If the execution is successful, execvp
does not return; if it fails, it returns -1 and sets errno
to indicate the error.
How to Use execvp
Using execvp
is straightforward, but it requires a good understanding of how processes work in C. Below is a simple example demonstrating how to use execvp
to execute the ls
command, which lists directory contents.
#include <stdio.h>
#include <unistd.h>
int main() {
char *args[] = {"ls", "-l", NULL};
if (execvp(args[0], args) == -1) {
perror("execvp failed");
}
return 0;
}
Output:
total 0
-rw-r--r-- 1 user user 0 Oct 1 00:00 file1.txt
-rw-r--r-- 1 user user 0 Oct 1 00:00 file2.txt
In this example, we include the necessary header file unistd.h
and define the main
function. We create an array of strings called args
, where the first element is the command we want to execute (ls
), followed by its options (-l
), and we terminate the array with a NULL pointer. The execvp
function is then called with the command and arguments. If it fails, we print an error message using perror
.
This simple implementation demonstrates how execvp
can be used to execute system commands directly from a C program.
Error Handling with execvp
Error handling is crucial when using execvp
, as it can fail for various reasons, such as the command not being found or insufficient permissions. Proper error handling ensures that your program behaves predictably in these situations.
#include <stdio.h>
#include <unistd.h>
#include <errno.h>
#include <string.h>
int main() {
char *args[] = {"nonexistent_command", NULL};
if (execvp(args[0], args) == -1) {
fprintf(stderr, "Error executing command: %s\n", strerror(errno));
}
return 0;
}
Output:
Error executing command: No such file or directory
In this example, we attempt to execute a command that does not exist. When execvp
fails, we use strerror(errno)
to get a human-readable error message, which we then print to standard error. This approach helps in debugging and provides feedback to the user about what went wrong.
Combining execvp with Fork
Often, execvp
is used in conjunction with the fork
system call, which creates a new process. This combination allows you to execute a command in a child process while the parent process continues to run.
#include <stdio.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/wait.h>
int main() {
pid_t pid = fork();
if (pid == 0) {
char *args[] = {"ls", NULL};
execvp(args[0], args);
perror("execvp failed");
} else if (pid > 0) {
wait(NULL);
printf("Child process completed.\n");
} else {
perror("fork failed");
}
return 0;
}
Output:
total 0
-rw-r--r-- 1 user user 0 Oct 1 00:00 file1.txt
-rw-r--r-- 1 user user 0 Oct 1 00:00 file2.txt
Child process completed.
In this code, we first call fork()
to create a new process. If fork()
returns 0, we are in the child process, where we call execvp
to execute the ls
command. If fork()
returns a positive value, we are in the parent process, where we wait for the child process to finish using wait()
. This structure allows the parent process to continue running while the child executes the command, demonstrating a common pattern in Unix programming.
Conclusion
The execvp
function in C is a vital tool for executing external programs, allowing developers to create dynamic and interactive applications. By understanding how to use execvp
effectively, including error handling and process management with fork
, you can enhance your programming capabilities. Whether you’re developing system utilities, command-line tools, or just exploring the depths of C programming, mastering execvp
will significantly expand your toolkit.
FAQ
-
What does execvp do in C?
execvp replaces the current process image with a new process image specified by the given command and arguments. -
How do I handle errors with execvp?
You can check the return value of execvp. If it returns -1, you can use errno to determine the error and print a message. -
Can I use execvp without fork?
Yes, but using execvp without fork will replace the current process, meaning the program will terminate after execvp is called. -
What header file is required for execvp?
You need to include the unistd.h header file to use execvp in your C program. -
Can execvp execute scripts?
Yes, execvp can execute scripts as long as the script is executable and its path is correctly specified.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook