How to Use typedef enum in C
-
Use
enum
to Define Named Integer Constants in C -
Use
typedef enum
to Define Custome Type for Object Containing Named Integer Constants
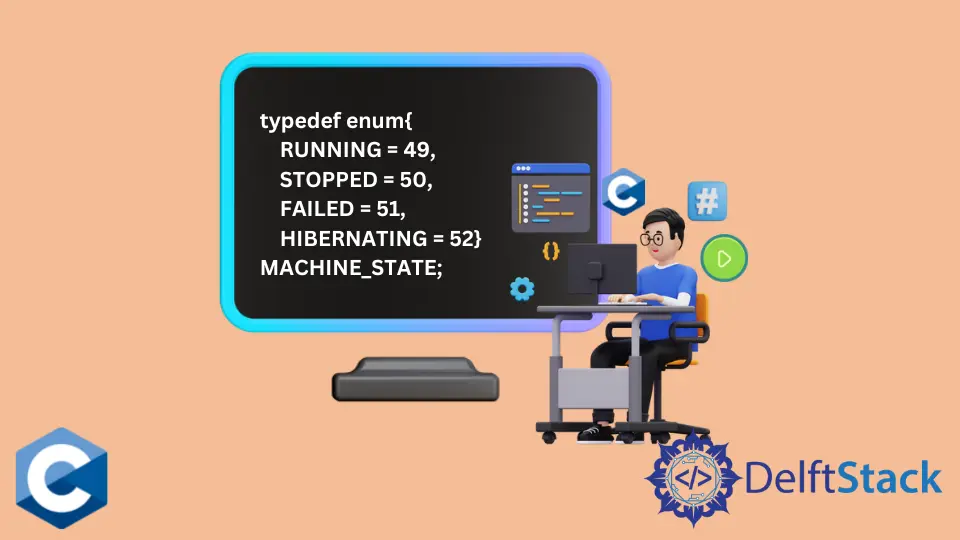
This article will demonstrate multiple methods about how to use typedef enum
in C.
Use enum
to Define Named Integer Constants in C
enum
keyword defines a special type called enumeration. Enumerations are basically just integral values that have names as variables but are read-only objects and can’t be modified at run-time.
There are two ways to construct an enum
object; one is to declare each member without assigning explicit value but automatically deduced values based on the position; the other is to declare the members and assign the explicit values.
In the below example, we assign custom values to each of them and named the object - STATE
. Next, we can use member names of the STATE
object in the code as signed integers and evaluate them in expressions. The following example code demonstrates the multiple conditional statements that check if the input integer is equal to the defined constant and print the corresponding suitable string once it’s matched.
#include <stdio.h>
#include <stdlib.h>
enum STATE { RUNNING = 49, STOPPED = 50, FAILED = 51, HIBERNATING = 52 };
int main(void) {
int input1;
printf("Please provide integer in range [1-4]: ");
input1 = getchar();
if (input1 == STOPPED) {
printf("Machine is stopped\n");
} else if (input1 == RUNNING) {
printf("Machine is running\n");
} else if (input1 == FAILED) {
printf("Machine is in failed state\n");
} else if (input1 == HIBERNATING) {
printf("Machine is hibernated\n");
}
exit(EXIT_SUCCESS);
}
Output:
Please provide integer in range [1-4]: 2
Machine is stopped
Use typedef enum
to Define Custome Type for Object Containing Named Integer Constants
The typedef
keyword is used to name user-defined objects. Structures often have to be declared multiple times in the code. Without defining them using typedef
each declaration would need to start with the struct
/enum
keyword, which makes the code quite overloaded for readability.
Note though, typedef
merely creates a new alias name for the given type rather than creating a new type. Many integer types like size_t
, uint
and others are just typedef
of other built-in types. Thus, the user can declare multiple name aliases for built-in types and then chain additional typedef
declarations using already created aliases.
#include <stdio.h>
#include <stdlib.h>
typedef enum {
RUNNING = 49,
STOPPED = 50,
FAILED = 51,
HIBERNATING = 52
} MACHINE_STATE;
int main(void) {
int input1;
MACHINE_STATE state;
printf("Please provide integer in range [1-4]: ");
input1 = getchar();
state = input1;
switch (state) {
case RUNNING:
printf("Machine is running\n");
break;
case STOPPED:
printf("Machine is stopped\n");
break;
case FAILED:
printf("Machine is in failed state\n");
break;
case HIBERNATING:
printf("Machine is hibernated\n");
break;
default:
break;
}
exit(EXIT_SUCCESS);
}
Output:
Please provide integer in range [1-4]: 2
Machine is stopped
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook