How to Use the bzero Function in C
-
Use the
bzero
Function to Zero Out the Memory Region in C -
Use
explicit_bzero
Function to Zero Out the Memory Region in C -
Use
memset
Function to Zero Out the Memory Region in C
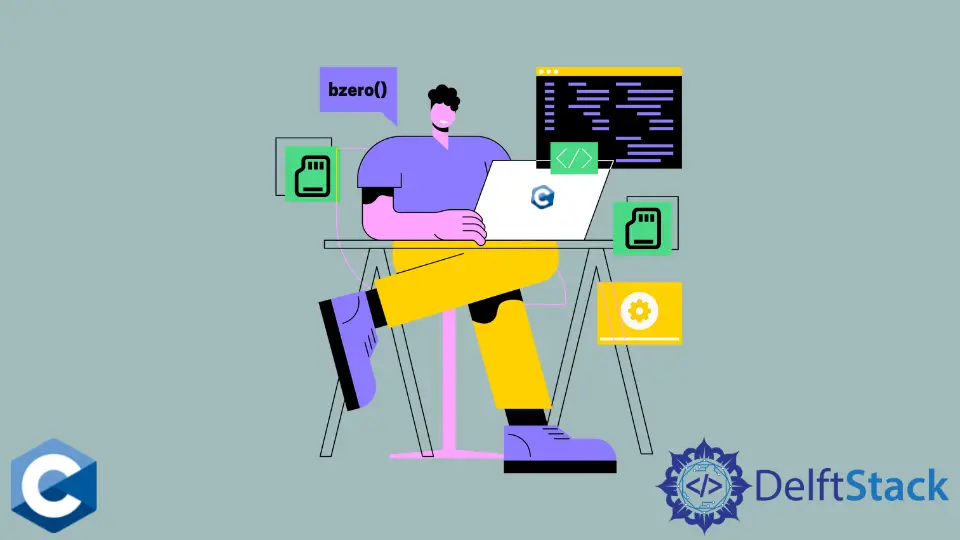
This article will demonstrate multiple methods about how to use the bzero
function in C.
Use the bzero
Function to Zero Out the Memory Region in C
Memory management is one of C programming’s core tasks as the user is required to interact with the basic memory structures and operate on them. Thus, zeroing out the memory region is the common operation used in many scenarios. Sometimes, dynamic memory should be overwritten with zeros to clear it from garbage values. Sometimes, there are struct
containing multiple bit-mask values that need to be explicitly zeroed out before its members’ initialization. In this example, we demonstrate the code that zeroes out the socket address structure that is later used to bind to the given socket. The bzero
function can be used to erase the given memory area with zero bytes (\0
). It takes two arguments, the starting address of the memory region and the number of bytes that need to be zeroed out.
#include "stdio.h"
#include "stdlib.h"
#include "string.h"
#include "sys/socket.h"
#include "sys/un.h"
#include "unistd.h"
const char *SOCKNAME = "/tmp/mysocket";
int main(int argc, char *argv[]) {
int sfd;
struct sockaddr_un addr;
sfd = socket(AF_UNIX, SOCK_STREAM, 0);
if (sfd == -1) {
perror("socket");
exit(EXIT_FAILURE);
}
bzero(&addr, sizeof(struct sockaddr_un));
addr.sun_family = AF_UNIX;
strncpy(addr.sun_path, SOCKNAME, sizeof(addr.sun_path) - 1);
if (bind(sfd, (struct sockaddr *)&addr, sizeof(struct sockaddr_un)) == -1) {
perror("bind");
exit(EXIT_FAILURE);
}
if (close(sfd) == -1) {
perror("close");
exit(EXIT_FAILURE);
}
exit(EXIT_SUCCESS);
}
Use explicit_bzero
Function to Zero Out the Memory Region in C
Another method to overwrite the memory region with zeros is to use the explicit_bzero
function. In contrast to the bzero
function, explicit_bzero
guarantees that the memory area is overwritten even if compiler optimizations deduce that the function is unnecessary
. Note that this function is a nonstandard extension of the C language and may not be included in some compilers.
#include "stdio.h"
#include "stdlib.h"
#include "string.h"
#include "sys/socket.h"
#include "sys/un.h"
#include "unistd.h"
const char *SOCKNAME = "/tmp/mysocket";
int main(int argc, char *argv[]) {
int sfd;
struct sockaddr_un addr;
sfd = socket(AF_UNIX, SOCK_STREAM, 0);
if (sfd == -1) {
perror("socket");
exit(EXIT_FAILURE);
}
explicit_bzero(&addr, sizeof(struct sockaddr_un));
addr.sun_family = AF_UNIX;
strncpy(addr.sun_path, SOCKNAME, sizeof(addr.sun_path) - 1);
if (bind(sfd, (struct sockaddr *)&addr, sizeof(struct sockaddr_un)) == -1) {
perror("bind");
exit(EXIT_FAILURE);
}
if (close(sfd) == -1) {
perror("close");
exit(EXIT_FAILURE);
}
exit(EXIT_SUCCESS);
}
Use memset
Function to Zero Out the Memory Region in C
memset
is part of the standard C library and also recommended method for most scenarios between these three functions. bzero
is depreciated function and should not be used in modern codebases. Although, memset
operation can be optimized out by the compiler as opposed to the explicit_bzero
.
memset
takes three arguments:
- The memory address.
- Constant byte to fill memory with.
- The number of bytes to overwrite.
memset
returns a pointer to the memory area and can be used in chained function calls.
#include "stdio.h"
#include "stdlib.h"
#include "string.h"
#include "sys/socket.h"
#include "sys/un.h"
#include "unistd.h"
const char *SOCKNAME = "/tmp/mysocket4";
int main(int argc, char *argv[]) {
int sfd;
struct sockaddr_un addr;
sfd = socket(AF_UNIX, SOCK_STREAM, 0);
if (sfd == -1) {
perror("socket");
exit(EXIT_FAILURE);
}
memset(&addr, 0, sizeof(struct sockaddr_un));
addr.sun_family = AF_UNIX;
strncpy(addr.sun_path, SOCKNAME, sizeof(addr.sun_path) - 1);
if (bind(sfd, (struct sockaddr *)&addr, sizeof(struct sockaddr_un)) == -1) {
perror("bind");
exit(EXIT_FAILURE);
}
if (close(sfd) == -1) {
perror("close");
exit(EXIT_FAILURE);
}
exit(EXIT_SUCCESS);
}
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook