How to Use Bitwise Shift Operations in C
- Understanding Bitwise Shift Operations
- Using Left Shift Operator
- Using Right Shift Operator
- Right Shift - Arithmetic vs Logical Shift Difference in C
- Practical Applications of Bitwise Shifts
- Conclusion
- FAQ
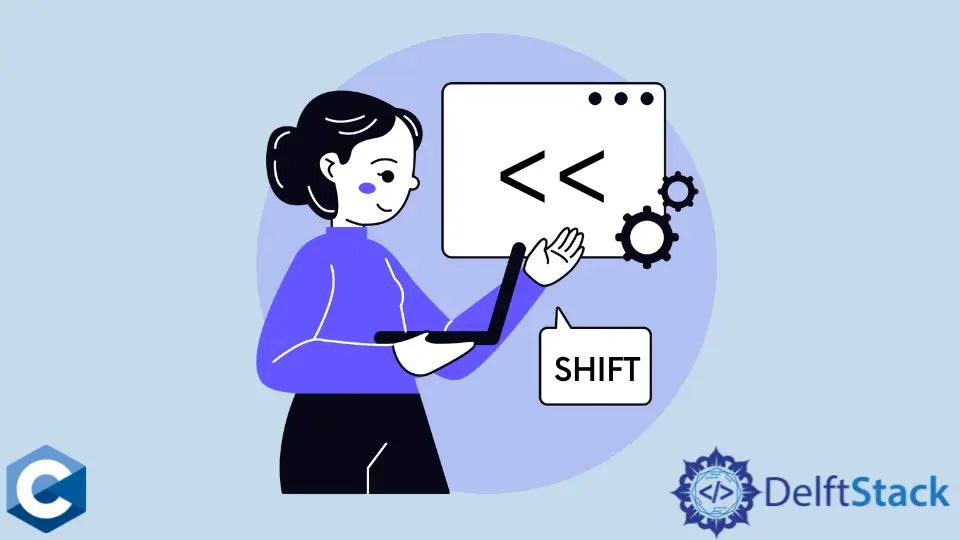
Bitwise shift operations are fundamental in C programming, allowing you to manipulate data at the bit level. These operations can significantly enhance performance, especially in applications that require low-level data processing, such as graphics programming, embedded systems, and cryptography.
In this article, we’ll explore how to effectively use bitwise shift operations in C. We will cover both left and right shifts, providing clear examples and explanations. Whether you’re a beginner looking to grasp the basics or an experienced programmer wanting to refine your skills, this guide will illuminate the practical applications of bitwise shifts in C.
Understanding Bitwise Shift Operations
Bitwise shift operations involve moving bits to the left or right within a binary representation of a number. In C, you can perform two types of shifts: left shift (<<
) and right shift (>>
). The left shift operator moves bits to the left, effectively multiplying the number by two for each shift. Conversely, the right shift operator moves bits to the right, which divides the number by two for each shift, discarding any remainder.
For example, if you have a binary number 00000001
(which is 1 in decimal) and you apply a left shift (1 << 1
), the result will be 00000010
(which is 2 in decimal). This operation is not just limited to integers; it can also be applied to other data types, making it versatile.
Using Left Shift Operator
The left shift operator (<<
) is a powerful tool in C that allows you to multiply an integer by powers of two. When you apply a left shift, you are essentially adding zeros to the right of the binary representation of the number. This operation is efficient and often used in scenarios where performance is critical.
Here’s a simple example demonstrating the left shift operator:
#include <stdio.h>
int main() {
int num = 5; // Binary: 00000000 00000000 00000000 00000101
int result = num << 2; // Shift left by 2 bits
printf("Original number: %d\n", num);
printf("After left shift: %d\n", result);
return 0;
}
Output:
Original number: 5
After left shift: 20
In this code, we start with the number 5, which is represented in binary as 00000000 00000000 00000000 00000101
. When we left shift this number by 2 bits, we get 00000000 00000000 00000000 00010100
, which equals 20 in decimal. This operation effectively multiplies the original number by 4 (2^2).
Using Right Shift Operator
The right shift operator (>>
) is equally useful, allowing you to divide an integer by powers of two. When you apply a right shift, the bits are shifted to the right, and the leftmost bits are filled based on the sign of the number (for signed integers). This operation can be particularly useful in algorithms where you need to quickly halve a number or extract specific bits.
Here’s an example of using the right shift operator:
#include <stdio.h>
int main() {
int num = 20; // Binary: 00000000 00000000 00000000 00010100
int result = num >> 2; // Shift right by 2 bits
printf("Original number: %d\n", num);
printf("After right shift: %d\n", result);
return 0;
}
Output:
Original number: 20
After right shift: 5
In this example, we start with the number 20, represented in binary as 00000000 00000000 00000000 00010100
. When we right shift this number by 2 bits, we get 00000000 00000000 00000000 00000101
, which equals 5 in decimal. This operation effectively divides the original number by 4 (2^2).
Right Shift - Arithmetic vs Logical Shift Difference in C
It should be mentioned that signed
and unsigned
integers are represented differently underneath the hood. Namely, signed
ones are implemented as two’s complement values. As a result, the most significant bit of negative numbers is 1
called sign bit, whereas positive integers start with 0
as usual. Thus, when we shift the negative numbers right logically, we lose their sign and get the positive integer. So, we need to differentiate logical and arithmetic shifts, the latter of which preserves the most significant bit. Even though there’s difference between concepts, C does not provide separate operators. Additionally, the C standard does not specify the behavior, as it is defined by hardware implementation. As shown in the following example output, the underlying machine conducted the arithmetic shift and preserved the integer’s negative value.
#include <stdio.h>
#include <stdlib.h>
void binary(unsigned n) {
unsigned i;
for (i = 1 << 31; i > 0; i /= 2) (n & i) ? printf("1") : printf("0");
}
int main(int argc, char *argv[]) {
int n2 = -24;
binary(n2);
printf(" : %d\n", n2);
n2 >>= 3;
binary(n2);
printf(" : %d\n", n2);
exit(EXIT_SUCCESS);
}
Output:
11111111111111111111111111101000 : -24
11111111111111111111111111111101 : -3
Practical Applications of Bitwise Shifts
Bitwise shifts are not just theoretical concepts; they have practical applications in various programming scenarios. For instance, they are often used in low-level programming tasks, such as manipulating flags, performing arithmetic operations efficiently, and optimizing algorithms.
In graphics programming, bitwise shifts can be used to manipulate pixel data, allowing for faster computations when rendering images. In embedded systems, where resources are limited, using bitwise operations can save valuable processing time and memory.
Moreover, bitwise shifts are also used in cryptography for operations such as hashing and encryption, where manipulating data at the bit level is essential for security.
Conclusion
Bitwise shift operations in C provide a powerful means to manipulate data efficiently. Understanding how to use the left and right shift operators can enhance your programming skills and allow you to write more optimized code. Whether you’re working on performance-critical applications or learning the fundamentals of C, mastering bitwise shifts is an invaluable skill. As you continue your programming journey, keep these operations in mind, as they open up a world of possibilities in data manipulation.
FAQ
-
What are bitwise shift operations in C?
Bitwise shift operations are techniques used to manipulate bits in a binary number by shifting them left or right. -
How does the left shift operator work?
The left shift operator (<<
) shifts bits to the left, effectively multiplying the number by powers of two for each shift. -
What does the right shift operator do?
The right shift operator (>>
) shifts bits to the right, dividing the number by powers of two for each shift. -
Can bitwise shifts be used with negative numbers?
Yes, but the behavior of the right shift operator can vary based on whether it is an arithmetic or logical shift, depending on the compiler. -
Where are bitwise shifts commonly used?
Bitwise shifts are commonly used in low-level programming, graphics, embedded systems, and cryptography.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook