How to Stop a Loop Arduino
-
Stop the
void loop()
UsingSleep_n0m1
Library -
Stop the
void loop()
Using theexit(0)
Statement -
Stop the
void loop()
Using an Infinite Loop -
Stop the
void loop()
Using thereturn
Statement - Conclusion
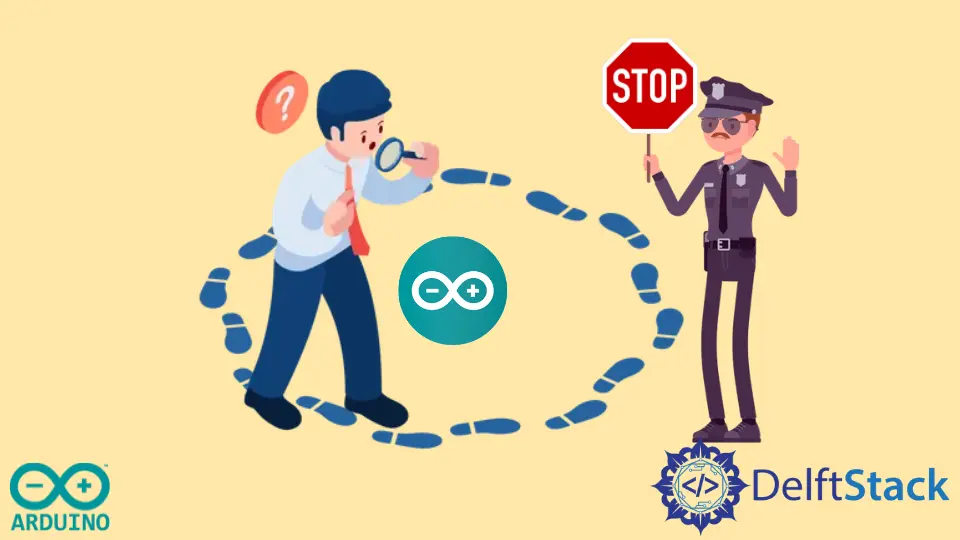
This guide explores various methods to halt the execution of the void loop()
in Arduino.
There are two types of loops in Arduino: the default void loop()
and user-created loops. User-created loops can be terminated using the break
method, while the default loop can be stopped using different approaches.
Stop the void loop()
Using Sleep_n0m1
Library
One effective method to gracefully terminate a loop involves using the Sleep_n0m1
library. This library provides a convenient way to put the Arduino into various sleep modes, conserving power consumption during periods of inactivity.
To use this library, include the header file (#include <Sleep_n0m1.h>
) and create an instance of the Sleep
class. The library facilitates the selection of different sleep modes and allows specifying the duration of the sleep using the sleepDelay()
method.
Code Example:
#include <Sleep_n0m1.h>
Sleep sleep;
unsigned long sleepTime; // set the duration for Arduino sleep
void setup() {
sleepTime = 50000; // set sleep time in ms
}
void loop() {
// Your Code
sleep.pwrDownMode(); // set sleep mode to Power Down
sleep.sleepDelay(sleepTime); // sleep for the specified duration
}
In this example, we began by including the Sleep_n0m1
library using #include <Sleep_n0m1.h>
. Next, we created an instance of the Sleep
class called sleep
.
We then declared an unsigned long variable, sleepTime
, to determine the duration for which the Arduino will sleep. In the setup()
function, we initialized sleepTime
with the desired sleep duration specified in milliseconds.
The loop()
function is where our code execution occurs. You can replace the comment Your Code
with the specific operations or tasks you want the Arduino to perform.
Within the loop()
function, two important lines implement the Sleep_n0m1
library functionality. The line sleep.pwrDownMode();
sets the sleep mode to Power Down, indicating the deepest sleep mode available, which minimizes power consumption.
The subsequent line, sleep.sleepDelay(sleepTime);
instructs the Arduino to sleep for the specified duration.
This combination of sleep mode selection and sleep duration allows the Arduino to effectively pause its operations, conserving power until it is either manually reset or a timer event triggers a wakeup.
It’s essential to use the Sleep_n0m1
library judiciously, incorporating it at the appropriate point in your code where extended periods of inactivity are expected.
Stop the void loop()
Using the exit(0)
Statement
Another straightforward and immediate way to cease the execution of the void loop()
in Arduino is by using the exit(0)
statement. It is a simple yet powerful command that can be placed within the loop()
function.
When encountered, it causes the immediate termination of the loop, effectively stopping any further execution of code within the loop. The argument 0
passed to exit()
is not strictly necessary for its functionality but is commonly included to indicate a successful termination.
Code Example:
void loop() {
// All of your code here
// exit the loop
exit(0); // 0 is required to prevent an error.
}
In this concise example, we are operating within the loop()
function, where the main code execution takes place. The comment All of your code here
serves as a placeholder for the specific tasks or operations you want the Arduino to perform.
The crucial line in this example is exit(0);
, strategically placed after your code. When the Arduino reaches this line, it immediately halts the execution of the loop()
, preventing any subsequent code from being processed.
The 0
passed as an argument is a convention to indicate successful termination, although it is not strictly required for the exit()
statement to function.
It’s important to note that using exit(0)
comes with a caveat: once encountered, the Arduino will cease all operations and remain inactive until manually reset. Therefore, careful consideration is required to ensure that this method aligns with the intended functionality of your Arduino sketch.
In scenarios where an immediate and unconditional termination of the loop is necessary, the exit(0)
statement provides a direct and effective solution. However, its use should be approached with caution, considering the potential consequences of abruptly halting the Arduino’s execution.
Stop the void loop()
Using an Infinite Loop
In certain situations, we encounter challenges where conventional methods may not be directly applicable. One such versatile approach to stopping a loop in Arduino involves utilizing an infinite loop.
While this method might seem counterintuitive at first, it can be a pragmatic solution, especially when dealing with diverse Arduino boards.
An infinite loop is essentially a loop that continues indefinitely, providing a continuous execution environment for the code within it.
By inserting an infinite loop at the end of the void loop()
, we create a scenario where the Arduino processes the existing code and then enters the infinite loop, effectively halting any further execution until a manual reset occurs.
Code Example:
void loop() {
// All of your code
while (1) {
// Infinite loop
}
}
Here, the loop()
function begins with the comment All of your code
. This is where you insert the specific tasks or operations you want the Arduino to perform.
Following this code block, we encounter a while
loop with the condition while (1)
. This condition, 1
being a constant that always evaluates to true, creates an infinite loop.
Once the Arduino finishes processing the code above the infinite loop, it enters this loop and remains there indefinitely. The effect is akin to a pause in execution, as the Arduino will not proceed beyond the infinite loop until it is manually reset.
While the infinite loop method ensures the Arduino stops processing further code, it comes with a trade-off—continuous power consumption. Unlike other methods that allow the Arduino to enter low-power states, the infinite loop keeps the Arduino awake, drawing power continuously.
Stop the void loop()
Using the return
Statement
There’s yet another effective method to halt the execution of the void loop()
: the return
statement. Typically used within functions, this statement serves the purpose of exiting a function prematurely.
When applied within the void loop()
, it effectively halts the execution of the loop, allowing for an immediate exit. Similar to the exit(0)
statement, the return
statement can be utilized to terminate the loop under specific circumstances.
Code Example:
bool exit_condition = false;
void setup() { Serial.begin(9600); }
void loop() {
// Your code here
if (exit_condition) {
return;
}
delay(1000); // Pause for 1 second (1000 milliseconds)
}
In this example, the loop()
function begins with the comment Your code here
, indicating the section where you insert the tasks or operations you want the Arduino to perform. Following this section, there’s an if
statement that checks a specified condition (exit_condition
).
If this condition evaluates to true
, the return
statement is executed, immediately exiting the void loop()
.
The return
statement functions as an abrupt exit, ensuring that any code appearing after it within the loop will not be processed. This can be particularly useful when there’s a need to terminate the loop based on a specific criterion.
It’s worth noting that the return
statement doesn’t require an argument, unlike in functions where a value may be returned.
Conclusion
Each method has its considerations, and the choice depends on the specific requirements of the Arduino sketch. The Sleep_n0m1
library is suitable for conserving power, exit(0)
provides immediate termination, an infinite loop can be versatile, and the return
statement allows conditional exits.
Careful consideration is essential to align the chosen method with the intended functionality of the Arduino sketch.