Arduino Nested if Statement
- Understanding Nested If Statements
- Practical Applications of Nested If Statements
- Tips for Using Nested If Statements Effectively
- Conclusion
- FAQ
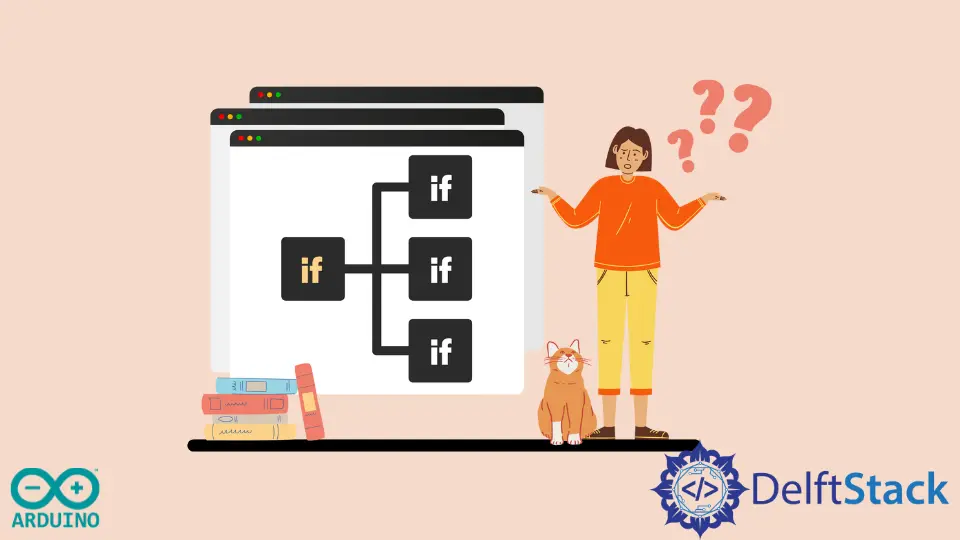
When programming with Arduino, understanding control structures is essential. One such structure is the nested if statement, which allows you to place an if statement inside another if statement. This technique is particularly useful for handling complex conditions where multiple criteria must be met before executing specific actions.
In this article, we will explore the concept of nested if statements in Arduino programming. We will provide clear examples and detailed explanations to help you grasp this fundamental programming concept. Whether you’re a beginner or looking to enhance your Arduino skills, this guide will equip you with the knowledge to effectively use nested if statements in your projects. To broaden your understanding of Arduino programming, you might also want to explore how to use subroutine in Arduino, which can help manage code more effectively.
Understanding Nested If Statements
Nested if statements allow you to evaluate multiple conditions in a structured manner. In Arduino, the syntax is straightforward. The outer if statement checks a primary condition, and if that condition is true, the inner if statement checks a secondary condition. This structure is beneficial when you need to make decisions based on multiple criteria.
Here’s a simple example to illustrate how nested if statements work:
int temperature = 30;
int humidity = 70;
if (temperature > 25) {
if (humidity > 60) {
Serial.println("It's hot and humid.");
} else {
Serial.println("It's hot but not humid.");
}
} else {
Serial.println("It's not hot.");
}
In this code, we first check if the temperature is greater than 25. If it is, we then check the humidity. Depending on whether the humidity is above or below 60, we print different messages. If the temperature is not above 25, we simply print that it’s not hot.
Output:
It's hot and humid.
This example demonstrates how nested if statements can help you make more refined decisions in your Arduino code.
Practical Applications of Nested If Statements
Nested if statements can be applied in numerous scenarios. For instance, consider a temperature and humidity monitoring system where different actions are taken based on environmental conditions. Here’s how you might implement such a system:
int temperature = 28;
int humidity = 85;
if (temperature > 25) {
if (humidity > 80) {
Serial.println("Warning: High temperature and humidity!");
} else if (humidity > 60) {
Serial.println("Warning: High temperature but normal humidity.");
} else {
Serial.println("Warning: High temperature and low humidity.");
}
} else {
Serial.println("Temperature is within the normal range.");
}
In this example, the code checks both the temperature and humidity levels. Based on the conditions, it issues different warnings. If the temperature is above 25 degrees and the humidity is above 80, it warns of high conditions. If the humidity is between 60 and 80, it gives a different warning, and if the humidity is low, it provides yet another message. If the temperature is not above 25, it simply states that the temperature is normal.
Output:
Warning: High temperature and humidity!
This structured approach allows for more precise monitoring and response in your Arduino projects, making your applications smarter and more efficient.
Tips for Using Nested If Statements Effectively
When using nested if statements in Arduino programming, there are a few best practices to keep in mind:
- Keep It Simple: While nesting can help manage complex conditions, excessive nesting can make your code difficult to read. Aim for clarity.
- Use Else If: If you find yourself nesting multiple if statements, consider using else if for simpler conditions. This can reduce complexity.
- Comment Your Code: Even though we aim for clarity, adding comments to your nested logic can help others (and your future self) understand the decision-making process.
Here’s an example that incorporates these tips:
int lightLevel = 300;
int motionDetected = 1;
if (lightLevel < 200) {
if (motionDetected) {
Serial.println("Light ON: Motion detected in low light.");
} else {
Serial.println("Light OFF: No motion in low light.");
}
} else {
Serial.println("Light OFF: Sufficient light available.");
}
In this code, we check the light level first. If it’s below 200, we then check if motion is detected. This way, we can turn the light on only when necessary. If the light level is sufficient, we simply turn the light off.
Output:
Light ON: Motion detected in low light.
By following these tips, you can create efficient and readable nested if statements in your Arduino projects.
Conclusion
Nested if statements are a powerful tool in Arduino programming, allowing you to create complex decision-making structures. By understanding how to implement and utilize these statements effectively, you can enhance the functionality of your projects. Whether you’re monitoring environmental conditions or controlling devices based on various inputs, mastering nested if statements will significantly improve your coding skills. With practice, you’ll find that these structures can make your code cleaner and more efficient.
FAQ
-
What is a nested if statement in Arduino?
A nested if statement is an if statement placed inside another if statement, allowing for multiple conditions to be evaluated in a structured way. -
When should I use nested if statements?
Use nested if statements when you need to evaluate multiple conditions that depend on one another, especially in complex decision-making scenarios. -
Can I use nested if statements in other programming languages?
Yes, nested if statements are a common feature in many programming languages, including C, C++, and Java.
-
How can I simplify my nested if statements?
Consider using else if statements for simpler conditions and avoid excessive nesting to keep your code readable. -
Are there alternatives to nested if statements?
Yes, switch statements or logical operators (AND, OR) can sometimes replace nested if statements, depending on the situation.