Arduino memcpy and memmove
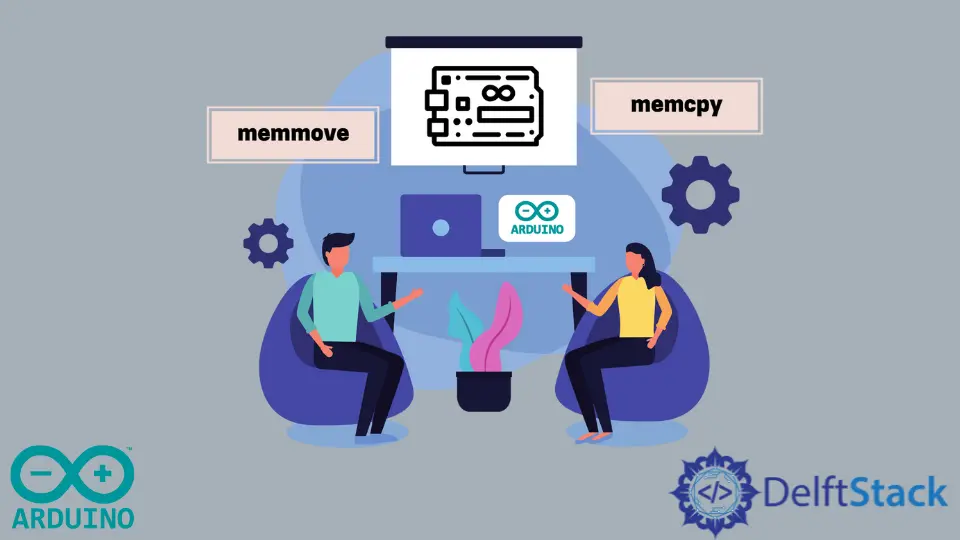
When working with Arduino, efficient memory management is crucial for performance and stability. Two functions that come in handy for copying blocks of memory are memcpy()
and memmove()
. These functions allow developers to manipulate data in memory with ease. Understanding how to use memcpy()
and memmove()
effectively can help you optimize your Arduino projects, whether you’re transferring data between variables or managing arrays.
In this article, we’ll explore these functions, provide examples, and discuss their differences, ensuring you have a solid grasp of memory copying in the Arduino environment.
What is memcpy()?
memcpy()
is a standard library function used to copy a specified number of bytes from one memory location to another. It’s particularly useful when you want to transfer data quickly without worrying about overlapping memory areas. The syntax is straightforward:
void *memcpy(void *dest, const void *src, size_t n);
Here, dest
is the destination address where the data will be copied, src
is the source address from which data is copied, and n
is the number of bytes to copy.
Example of using memcpy()
Let’s look at a simple example of using memcpy()
in an Arduino sketch:
#include <Arduino.h>
void setup() {
Serial.begin(9600);
char source[] = "Hello, Arduino!";
char destination[20];
memcpy(destination, source, sizeof(source));
Serial.println(destination);
}
void loop() {
// Empty loop
}
Output:
Hello, Arduino!
In this example, we declare a source character array containing the string “Hello, Arduino!” and a destination character array with enough space to hold the copied data. By calling memcpy()
, we copy the contents of the source array to the destination array. The result is printed to the Serial Monitor, showing the copied string.
Using memcpy()
is efficient when you’re sure that the source and destination do not overlap. However, if they do, you might run into unexpected results. This is where memmove()
comes into play.
What is memmove()?
memmove()
is similar to memcpy()
, but it has one significant advantage: it can handle overlapping memory regions safely. This means you can use memmove()
when the source and destination pointers point to the same memory area, and it will still function correctly. The syntax is as follows:
void *memmove(void *dest, const void *src, size_t n);
Just like memcpy()
, dest
is the destination address, src
is the source address, and n
is the number of bytes to transfer.
Example of using memmove()
Here’s an example demonstrating how to use memmove()
in an Arduino sketch:
#include <Arduino.h>
void setup() {
Serial.begin(9600);
char data[] = "Arduino Programming";
memmove(data + 8, data, 7);
Serial.println(data);
}
void loop() {
// Empty loop
}
Output:
Arduino Arduino
In this example, we have a character array data
containing the string “Arduino Programming.” We use memmove()
to copy the first seven characters of data
(the word “Arduino”) to a new position within the same array. This demonstrates how memmove()
can safely handle overlapping memory regions. The result is printed, showing that the word “Arduino” now appears twice in the string.
Key Differences Between memcpy() and memmove()
While both memcpy()
and memmove()
serve the purpose of copying memory, their key differences lie in how they handle overlapping memory areas and performance.
- Overlapping Regions:
memcpy()
does not handle overlapping memory areas safely, which can lead to corrupted data. In contrast,memmove()
is designed to manage overlapping regions effectively. - Performance:
memcpy()
is generally faster thanmemmove()
because it does not need to consider memory overlap. If you are sure that your source and destination do not overlap,memcpy()
is the better choice for speed.
Conclusion
Understanding how to use memcpy()
and memmove()
is essential for effective memory management in Arduino projects. These functions allow you to copy data efficiently, whether you’re transferring between variables or managing arrays. By knowing when to use each function, you can optimize your code and avoid potential pitfalls related to overlapping memory regions. With the examples provided, you should now feel more confident in implementing these functions in your Arduino sketches.
FAQ
-
What is the main purpose of memcpy() and memmove()?
memcpy() and memmove() are used to copy blocks of memory from one location to another in a program. -
Can I use memcpy() for overlapping memory areas?
No, memcpy() should not be used for overlapping memory areas as it can lead to data corruption. Use memmove() in such cases. -
Which function is faster, memcpy() or memmove()?
memcpy() is generally faster than memmove() because it does not account for overlapping memory regions. -
What happens if I use memcpy() with overlapping memory?
Using memcpy() with overlapping memory can result in undefined behavior and corrupted data. -
Are memcpy() and memmove() available in Arduino?
Yes, both functions are available in Arduino as part of the standard C library.