How to Convert Byte to Integer in Arduino
- Understanding Data Types in Arduino
-
Converting Byte to Integer Using the
int()
Function - Handling Larger Byte Arrays
- Practical Applications of Byte to Integer Conversion
- Conclusion
- FAQ
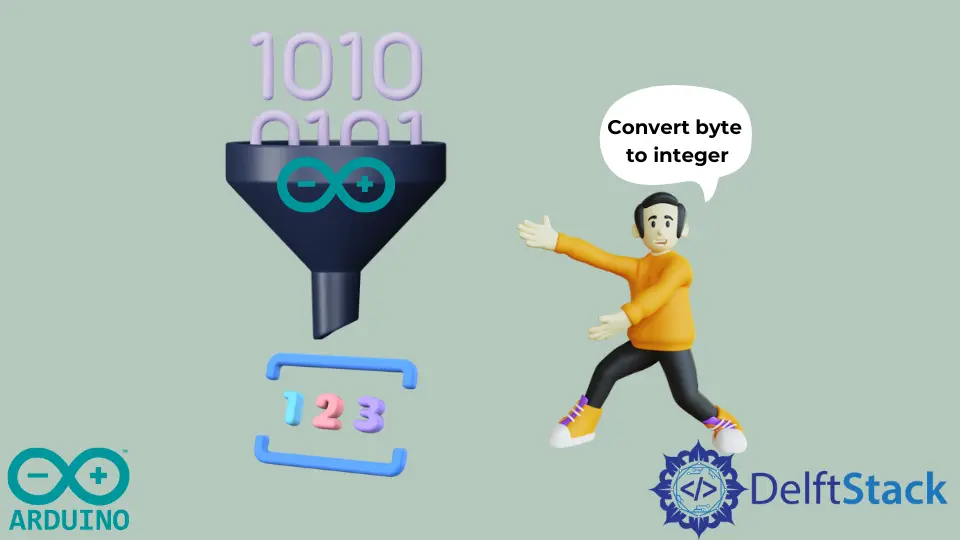
Converting a byte to an integer in Arduino is a fundamental task that many developers encounter. Understanding how to perform this conversion can enhance your ability to manipulate data effectively in your projects. In Arduino, the int()
function is a straightforward way to achieve this conversion. Whether you’re working on a simple LED control project or a more complex sensor application, knowing how to handle data types efficiently is crucial.
This article will walk you through the process of converting byte variables into integer variables using practical examples and clear explanations. Let’s dive into the details and make your Arduino programming experience smoother and more efficient.
Understanding Data Types in Arduino
Before we jump into the conversion process, it’s essential to grasp the significance of data types in Arduino programming. Arduino supports several data types, including byte
, int
, float
, and more. A byte
can hold values from 0 to 255, while an int
can store a much broader range, typically from -32,768 to 32,767 in a standard Arduino setup. This capability allows you to perform various calculations and operations with different ranges of numbers.
When you’re working with sensors or controlling devices, you may receive data in the form of bytes. However, to perform arithmetic operations or comparisons, you often need to convert these bytes into integers. The int()
function simplifies this process, enabling you to manage your data more effectively.
Converting Byte to Integer Using the int()
Function
The most common method to convert a byte to an integer in Arduino is by using the int()
function. This function takes a byte value as an argument and returns its integer equivalent. Here’s a simple example to illustrate this process:
byte myByte = 150;
int myInt = int(myByte);
Serial.begin(9600);
Serial.print("The byte value is: ");
Serial.println(myByte);
Serial.print("The converted integer value is: ");
Serial.println(myInt);
Output:
The byte value is: 150
The converted integer value is: 150
In this example, we start by defining a byte variable named myByte
with a value of 150. We then convert this byte to an integer using the int()
function and store the result in myInt
. The Serial
commands are used to print both the original byte value and the converted integer value to the Serial Monitor. This simple yet effective method allows you to easily handle byte-to-integer conversions in your Arduino projects.
Handling Larger Byte Arrays
In some cases, you may need to convert an entire array of bytes into integers. This situation is common when dealing with sensor data or communication protocols that return multiple bytes. To achieve this, you can loop through the byte array and convert each byte individually. Here’s how you can do it:
byte byteArray[] = {10, 20, 30, 40, 50};
int intArray[5];
for (int i = 0; i < 5; i++) {
intArray[i] = int(byteArray[i]);
}
Serial.begin(9600);
for (int i = 0; i < 5; i++) {
Serial.print("Byte value: ");
Serial.print(byteArray[i]);
Serial.print(" converted to Integer: ");
Serial.println(intArray[i]);
}
Output:
Byte value: 10 converted to Integer: 10
Byte value: 20 converted to Integer: 20
Byte value: 30 converted to Integer: 30
Byte value: 40 converted to Integer: 40
Byte value: 50 converted to Integer: 50
In this example, we define a byte array called byteArray
containing five byte values. We also create an integer array called intArray
to store the converted integer values. By using a for
loop, we iterate through each byte in byteArray
, convert it to an integer using the int()
function, and store the result in the corresponding index of intArray
. Finally, we print both the original byte values and their converted integer equivalents to the Serial Monitor.
Practical Applications of Byte to Integer Conversion
Understanding how to convert byte data to integers can significantly enhance your Arduino projects. For instance, if you’re working with a temperature sensor that returns byte values, converting these values to integers allows you to perform calculations, such as averaging multiple readings or comparing values to set thresholds for alerts. Additionally, when dealing with communication protocols like I2C or SPI, you may often receive data in byte format that needs conversion for processing.
Moreover, this conversion is crucial in applications involving data logging, where you might need to store sensor readings in a more manageable integer format. By mastering the byte-to-integer conversion process, you can improve the efficiency and functionality of your Arduino projects, making them more robust and versatile.
Conclusion
Converting a byte to an integer in Arduino is a straightforward yet vital skill for any developer. By utilizing the int()
function, you can easily handle data conversions that are essential for effective programming. Whether you’re working with individual bytes or arrays, understanding these conversions allows you to manipulate data more efficiently and build more complex applications. With the knowledge gained from this article, you are now equipped to tackle various programming challenges in your Arduino projects.
FAQ
-
What is the range of a byte in Arduino?
A byte can hold values from 0 to 255. -
Can I convert multiple bytes to integers at once?
Yes, you can loop through an array of bytes and convert each one to an integer. -
Why do I need to convert bytes to integers?
Converting bytes to integers allows for more complex calculations and comparisons in your Arduino projects. -
Is the
int()
function the only way to convert bytes?
While theint()
function is the most common method, you can also use type casting to achieve the same result. -
What happens if I try to convert a byte greater than 255 to an integer?
Since the byte can only hold values from 0 to 255, attempting to use a byte greater than this range will lead to an error or unexpected behavior.