How to Set Focus on the Input Field in Angular
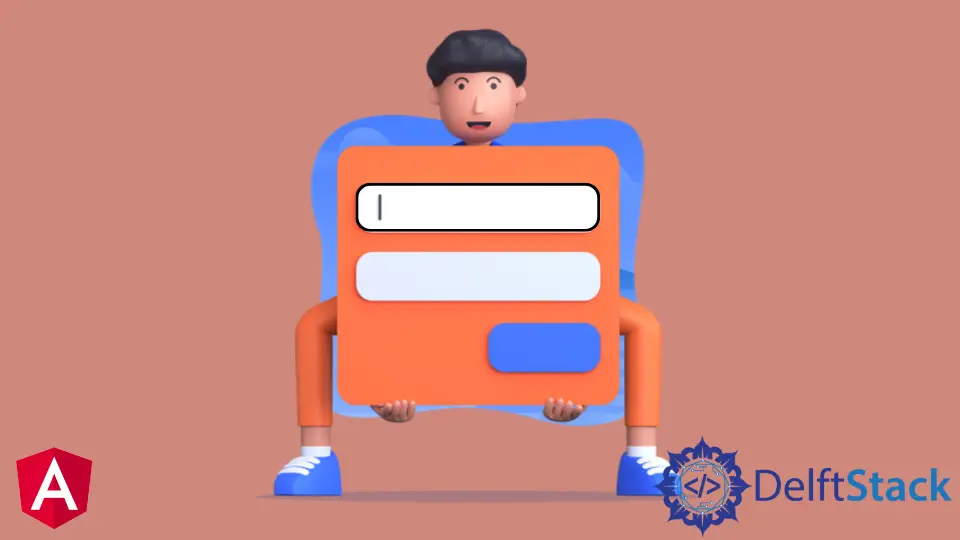
In Angular, you can use the ng-model
directive to focus on input fields for any HTML element and bind the input field’s value to a variable in the application model.
The ng-model
directive also provides an event handler that focuses on the input field when the user has filled it out. It fires an event when the user leaves this field, triggering validation logic or other behaviors.
This article will demonstrate setting the focus on the input field in Angular.
Steps to Set Focus on the Input Field in Angular
You need to follow the following steps to set focus in Angular.
-
Create an Angular component.
-
Add the input component to your component.
-
Create a
Focus
directive to manage the input field. -
Then, add this directive to the provider’s list by importing it into
app.module.ts
. -
After that, it’s an excellent approach to use the
ngBlur
directive to lose focus when the user leaves the input field.
Let’s apply the above steps and set focus in the Angular app.
JavaScript code:
var app = angular.module('Adil', []);
var Demo = function ($scope) {
};
app.directive('focusMe', function() {
return {
scope: { trigger: '=focusIn' },
link: function(scope, element) {
scope.$watch('trigger', function(value) {
if(value === true) {
element[0].focus();
scope.trigger = false;
}
});
}
};
});
HTML code:
<html ng-app="Adil">
<head>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.0.4/angular.js"></script>
<script src="example.js"></script>
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@4.4.1/dist/css/bootstrap.min.css" integrity="sha384-Vkoo8x4CGsO3+Hhxv8T/Q5PaXtkKtu6ug5TOeNV6gBiFeWPGFN9MuhOf23Q9Ifjh" crossorigin="anonymous">
</head>
<body>
<div ng-controller="Demo">
<button class="btn" ng-click="showForm=true;focusInput=true">click here</button>
<div ng-show="showForm">
<input type="text" focus-me="focusInput">
<br>
<button class="btn" ng-click="showForm=false">Click here to save the input field</button>
<p>
The black border around the corner shows that the input field is focused
</p>
</div>
</div>
</body>
</html>
Click here to check the live demonstration of the code above.
Here in this example, when you write something in the box, it shows a black border around the corner of the box, which indicates that it is focused.
Why is it necessary to set focus in Angular? Putting a focus on the input component in Angular makes sure that the user’s input is valid.
This ensures that the user doesn’t have typos and has entered all the necessary information. It can also save time when entering data into a form and provide a better experience for the user.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook