How to Filter Options in a Select List in Angular
- Understanding ng-options
- Implementing Filtering with ng-options
- Enhancing User Experience with Custom Filters
- Conclusion
- FAQ
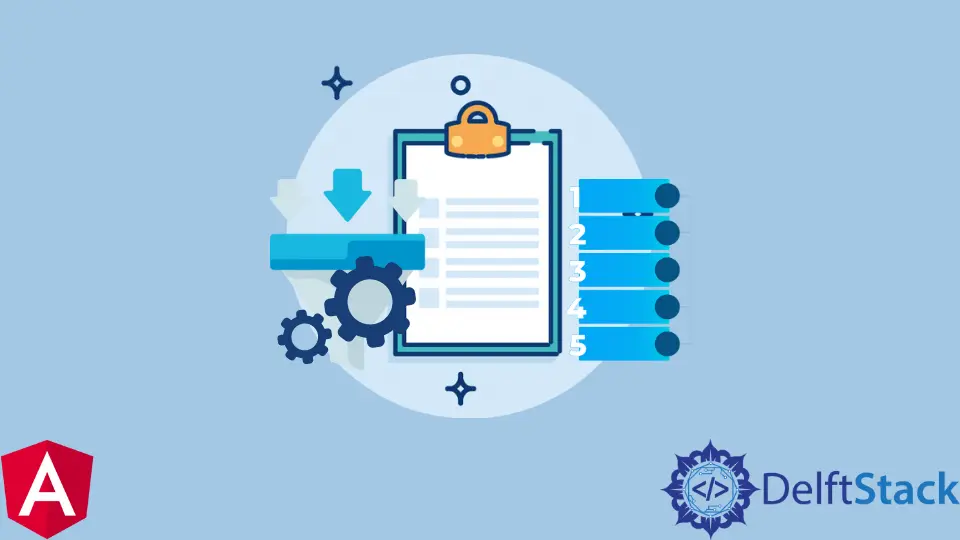
Creating dynamic and user-friendly applications is a hallmark of modern web development, and Angular offers powerful tools to achieve this. One such tool is the ng-options
directive, which simplifies the creation of dropdown lists in Angular applications. This directive allows developers to bind a model to an array of items, enabling users to select from a list of options easily.
In this article, we will explore how to filter options in a select list using Angular’s ng-options
directive. By the end, you’ll have a solid understanding of how to implement this functionality in your Angular applications, enhancing user experience and interactivity.
Understanding ng-options
Before diving into filtering options, it’s essential to grasp what ng-options
is and how it works. The ng-options
directive is designed to create a dropdown list that is bound to an array of objects in your Angular model. This binding allows for seamless updates to the view whenever the model changes, making it an efficient way to handle user input.
To use ng-options
, you typically define an array in your Angular controller and then bind it to a <select>
element in your HTML. Here’s a basic example to illustrate this:
<select ng-model="selectedItem" ng-options="item.name for item in items">
</select>
In this example, items
is an array of objects, and selectedItem
is the model that stores the user’s selection. The ng-options
directive uses the expression item.name for item in items
to display the names of the items in the dropdown.
Implementing Filtering with ng-options
Now that we understand the basics of ng-options
, let’s explore how to filter options in a select list. Filtering allows users to narrow down the choices, making it easier to find specific items, especially when dealing with large datasets.
To implement filtering, we can use an input field that binds to a search term and then filter the array based on that term. Here’s how to do it:
Step 1: Set Up Your Angular Controller
First, we need to define our items and a search term in the Angular controller. This will allow us to filter the options based on user input.
angular.module('app', []).controller('MainCtrl', function($scope) {
$scope.items = [
{ id: 1, name: 'Apple' },
{ id: 2, name: 'Banana' },
{ id: 3, name: 'Cherry' },
{ id: 4, name: 'Date' },
{ id: 5, name: 'Elderberry' }
];
$scope.searchTerm = '';
});
In this code snippet, we create an Angular module and controller, defining an array of fruit items and a search term string.
Step 2: Create the HTML Structure
Next, we’ll create the HTML structure to allow users to input their search term and display the filtered list in the dropdown.
<div ng-app="app" ng-controller="MainCtrl">
<input type="text" ng-model="searchTerm" placeholder="Search for fruits...">
<select ng-model="selectedItem" ng-options="item.name for item in items | filter:searchTerm">
</select>
</div>
In this HTML, we have an input field bound to searchTerm
, which filters the items
array. The filter
pipe processes the array, allowing us to display only the items that match the search term.
The combination of the input field and the ng-options
directive creates a dynamic dropdown that updates in real-time as the user types. This approach enhances user experience by simplifying the selection process.
Enhancing User Experience with Custom Filters
While the built-in filter functionality is powerful, we can also create custom filters for more complex scenarios. Custom filters can be particularly useful when you need specific filtering criteria that are not easily handled by the default filter.
Step 1: Define a Custom Filter
Let’s create a custom filter function in our Angular controller:
angular.module('app', []).controller('MainCtrl', function($scope) {
$scope.items = [
{ id: 1, name: 'Apple' },
{ id: 2, name: 'Banana' },
{ id: 3, name: 'Cherry' },
{ id: 4, name: 'Date' },
{ id: 5, name: 'Elderberry' }
];
$scope.searchTerm = '';
$scope.customFilter = function(item) {
return item.name.toLowerCase().includes($scope.searchTerm.toLowerCase());
};
});
In this code, we define a customFilter
function that checks if the item’s name includes the search term, ignoring case sensitivity.
Step 2: Update the HTML to Use the Custom Filter
Now, we need to modify our HTML to utilize this custom filter function:
<div ng-app="app" ng-controller="MainCtrl">
<input type="text" ng-model="searchTerm" placeholder="Search for fruits...">
<select ng-model="selectedItem" ng-options="item.name for item in items | filter:customFilter">
</select>
</div>
By replacing the default filter with our customFilter
, we allow for more nuanced filtering based on specific criteria.
Output:
The dropdown now reflects filtered options based on the custom filtering logic.
Using custom filters enhances the flexibility of your application, allowing for tailored user experiences that meet specific needs.
Conclusion
Filtering options in a select list using Angular’s ng-options
directive is a straightforward yet powerful way to enhance user interactivity in your applications. By implementing both built-in and custom filters, you can create a more dynamic and user-friendly experience. Whether you’re working with a small dataset or a large collection of items, these techniques will help users find what they need quickly and efficiently. As you develop your Angular applications, consider how filtering can improve usability and streamline the selection process.
FAQ
-
What is ng-options in Angular?
ng-options is a directive that simplifies creating dropdown lists by binding a model to an array of items. -
How can I filter items in a dropdown using Angular?
You can filter items using the filter pipe in conjunction with ng-options and an input field bound to a search term. -
Can I create custom filters in Angular?
Yes, you can create custom filter functions to handle more complex filtering logic, providing greater control over the filtering process.
-
Is filtering in Angular performance-intensive?
Filtering performance depends on the dataset size. For large datasets, consider optimizing your filter logic or using pagination. -
How do I handle case sensitivity in filtering?
You can manage case sensitivity by converting both the search term and the item values to lowercase before comparison.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook