How to Enable HTML5 Mode in AngularJS
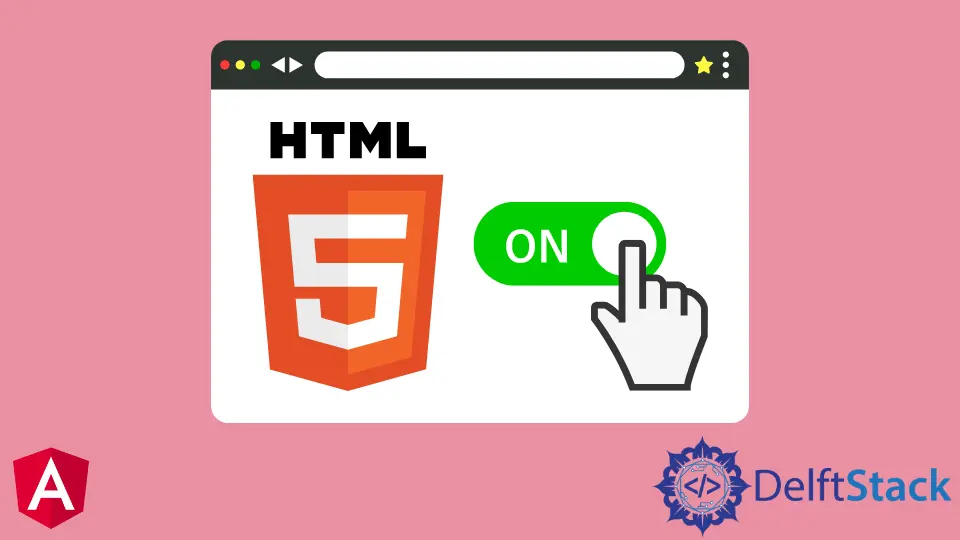
This article will guide you on enabling HTML5 mode with deep linking on your AngularJS application.
Uses of HTML5 Mode in AngularJS
The $locationProvider.html5Mode
is a way to tell the browser that it needs to use HTML5 mode for URLs instead of the older HTTP protocol. This will allow browsers to use features like pushState
, which is not supported by older browsers.
Furthermore, we should use it for AngularJS applications because it has some advantages over the older model:
$locationProvider.html5Mode
allows the developer to use HTML5’s History API for URL navigation and history management in their application, which provides a robust and reliable solution for handling URLs in-browser applications.- It’s easier to debug, and we can inspect every URL from within Chrome Developer Tools.
- It also offers better performance, especially when navigating between pages.
- It provides a bookmarkable experience to the users and enables features like share links, deep linking, etc.
Steps to Enable HTML5 Mode in AngularJS
The following steps will show you how to set up your AngularJS application for html5Mode
:
-
Include the
ngHref
library in your application’s module. -
Create a new service provider that overrides the
$locationProvider()
function and setshtml5Mode
as true. Setting this property to false will disablehtml5Mode
and use the default browser’s location handling.
TypeScript code:
var app = angular.module('Deo', ['ui.router']);
app.controller('Sample', function($scope, $state) {
$scope.name = 'demo';
});
app.config(function($stateProvider, $urlRouterProvider, $locationProvider) {
$locationProvider.html5Mode(true);
$stateProvider
.state('Home', {
url: '/Home',
template: '<h3>Home tab is active</h3>',
})
.state('About', {
url: '/About',
template: '<h3>About tab is active</h3>'
})
;
})
HTML code:
<!DOCTYPE html>
<html ng-app="Deo">
<head>
<meta charset="utf-8" />
<script>document.write('<base href="' + document.location + '" />');</script>
<script data-require="angular.js@1.3.x" src="https://cdnjs.cloudflare.com/ajax/libs/angular.js/1.3.7/angular.js" data-semver="1.3.7"></script>
<script data-require="ui-router@*" data-semver="0.2.13" src="//rawgit.com/angular-ui/ui-router/0.2.13/release/angular-ui-router.js"></script>
<script src="app.component.js"></script>
</head>
<body ng-controller="Sample">
<h2>Example of Angular html5Mode</h2>
{{state.current.name}}<br>
<ul>
<li><a ui-sref="Home">Home</a>
<li><a ui-sref="About">About</a>
</ul>
<div ui-view=""></div>
</body>
</html>
Click here to check the live demonstration of the code above.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook