How to Bind Select Element to Object in Angular 2
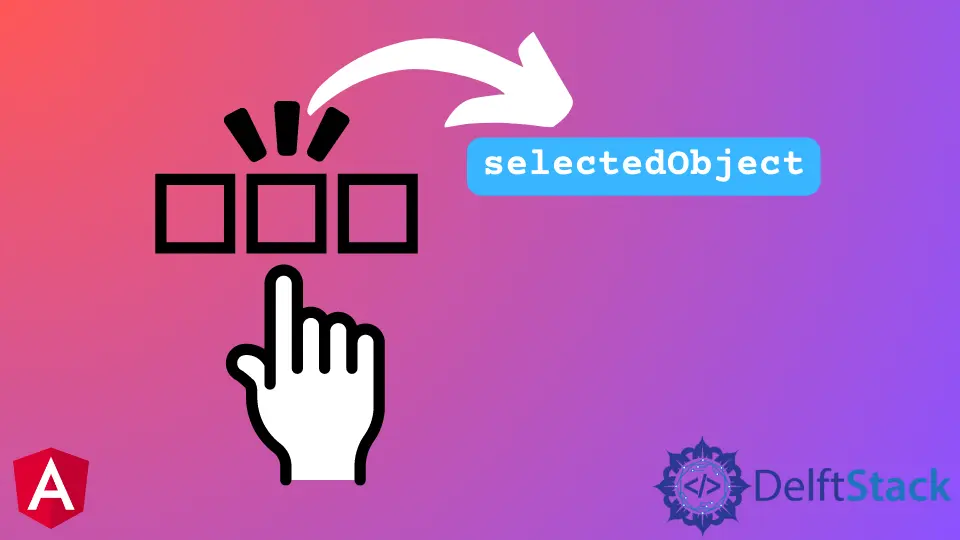
Angular 2 is a popular framework that is used to create web apps. One of the most common tasks in Angular 2 is binding a select element to an object.
This article will learn how to use ng-for
and ng-value
directives to bind a select element with an object in Angular 2.
Use the ng-for
and the ng-value
to Bind a Select Element to an Object in Angular 2
Ng-For
is a built-in template directive that enables it simple to loop over a collection of items, such as an array or an object, and build a template for each one.
*ngFor="let <value> of <collection>"
The ng-value
directive binds the select element to an object. It specifies the property’s name on the object that should map to the select element’s value.
<input ng-value="expression"></input>
Sometimes, beginners get confused between the ng-value
and value
. So if you are working on binding a select element with an object in Angular 2, you must know the difference between ng-value
and value
.
the Difference Between the ng-value
and the value
in Angular
The difference is that value
is always a string, whereas ngValue
allows you to pass an object. For example, you have a use case where you need to show the names of the items in the dropdown.
And when picking a single object from the menu, you must select the object’s id to search the database. It would help to use ngValue
in such a scenario because it needs an Object.
Furthermore, the object model you want to populate should be defined in your object.
Click here if you want to get more information about ng-value
.
Steps to Bind a Select Element to an Object in Angular 2
Some basic steps to follow are given below.
- Add the
ng-model
attribute to the select element. - Add the
selected
attribute to the object you want to bind to. - Bind the object property to the
select
element with a curly brace syntax,{{obj.property}}
.
Let’s have an example that will help us understand all the steps in detail.
First of all, we will create a component named AppComponent
. Then we will create an interface object by the name of example
that shows id
and name
.
In this case, both id
and name
store strings. After the two steps above, it’s time to modify the options by adding some information.
Complete /app.component.ts
code is given below.
import { Component } from '@angular/core';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: [ './app.component.css' ]
})
export class AppComponent {
name = 'Demo';
selectedObject : example;
SimpleExample = [
{id: 'Welcome to New York City', name: 'New York'},
{id: 'Welcome to Japan', name: 'Japan'},
{id: 'Welcome to Singapore', name: 'Singapore'},
{id: 'Welcome to Delft', name: 'Delft'}
]
}
interface example{
id:string;
name:string;
}
In the component.ts
file, we’ve added a variable selectedObject
, an object example
, and we will bind it to the select
element using ngModel
.
Another variable, SimpleExample
, includes a collection of objects used to display ngValue
select options.
Complete /app.component.html
code is given below.
<h2>Angular 2 Select Example</h2>
<select [(ngModel)]="selectedObject">
<option *ngFor="let show of SimpleExample" [ngValue]="show">
{{show.name}}
</option>
</select>
{{selectedObject | json}}
The example
object has now been added to the selectedObject
. And we used JSON pipe to display it.
The JSON Pipe can transform an input object into the desired result, which can then be assigned to a variable or passed as an argument. It uses the built-in JavaScript function JSON.stringify()
to transform the data into a JSON string.
To learn more about Angular JSON Pipe, click here.
We are getting the following output of the example mentioned above.
Click here to check the complete working code mentioned above.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook