How to Pass Data From One State to the next Using Angular state.go Function
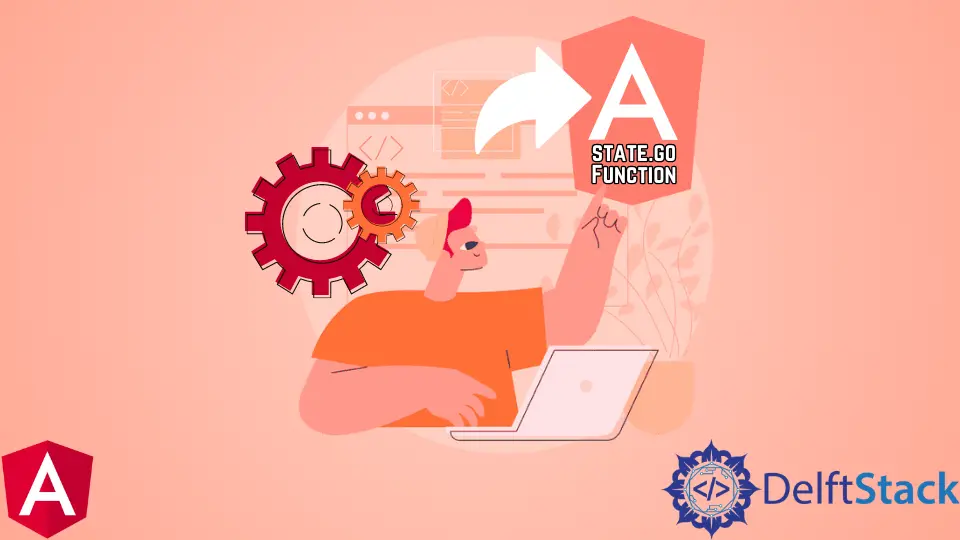
The state is a very important concept for building web applications, and it’s one of the core features of AngularJS. The AngularJS framework helps developers manage the state through two-way data binding and event handling.
This article will discuss the Angular state.go
function.
What Is UI-Router
in Angular
UI-Router
provides routing and navigation for single-page applications.
It is an open-source project created to address the limitations of AngularJS’s $route
service, which was used for URL handling and navigation before introducing Angular’s new router module.
We can use UI-Router
to develop features not available in the inbuilt one like routing, navigation, deep linking, etc.
Nesting and view named properties are not available in ngRoute
. It allows you to structure the UI rendering interface using state-based URLs.
It also enables the usage of many views on the same page, enhancing a view’s usefulness. UI-Router
is a fantastic tool for managing dynamic web applications.
What Is UI-Router’s $state.go
Function in Angular
Angular has many ways to pass data from one state to the next. For example, we can use a custom HTML attribute called ng-model
, and we can also use a custom event called ng-click
.
But $state.go
is probably the best way to pass an object from one state to the next because it’s less verbose and easier for other people who want to work on different Angular projects in the future.
Syntax of $state.go
is given below.
$state.go('destination.state');
Difference Between $state.go
and $location.path
in Angular
The $state.go
is an AngularJS directive that tells the view to update its URL to reflect the current state of the application. This directive is used to change the URL without navigating away from the current page.
The $location.path
is an AngularJS directive that tells the view to update its URL to reflect the application’s current location. This directive is used to navigate away from a particular page by changing its URL.
For new Angular developers, it might be hard to understand why we utilize both in different situations when the goal is the same.
You may manage location objects with the $location
service, and the UI-router
module includes the $state
service, which helps to manage routes in a complex manner. Only use the $state
service to manage states and routes using ui-router
.
Let’s discuss the example of $state.go
to understand it better.
Typescript code:
app = angular.module('myApp',['ui.router',])
app.config(['$stateProvider',function($stateProvider){
$stateProvider
.state('main',{
url: '/',
templateUrl:"main.html",
controller: 'Demo',
})
.state('Roll',{
url: '/Roll',
template:"<p> Saved</p>"
})
}]);
app.config(['$urlRouterProvider',function($urlRouterProvider){
$urlRouterProvider.otherwise('/');
}]);
app.controller('Demo', ['$scope', '$http', '$state'
,function($scope, $http, $state){
$scope.user = { RollNumber : "1234" }
$scope.save = function(){
$http.get("dummy.json", { params : $scope.user } )
.success(function(data){
$state.go("Roll");
})
}
}]);
HTML Code:
<!DOCTYPE html>
<html ng-app="myApp">
<head>
<script data-require="angular.js@1.3.0-beta.5" data-semver="1.3.0-beta.5" src="https://code.angularjs.org/1.3.0-beta.5/angular.js"></script>
<script data-require="ui-router@*" data-semver="0.2.11" src="https://rawgit.com/angular-ui/ui-router/0.2.10/release/angular-ui-router.js"></script>
<script data-require="lodash.js@*" data-semver="2.4.1" src="http://cdnjs.cloudflare.com/ajax/libs/lodash.js/2.4.1/lodash.js"></script>
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.4.1/css/bootstrap.min.css" integrity="sha384-Vkoo8x4CGsO3+Hhxv8T/Q5PaXtkKtu6ug5TOeNV6gBiFeWPGFN9MuhOf23Q9Ifjh" crossorigin="anonymous">
<script src="app.js"></script>
</head>
<body>
<div ui-view=""></div>
</body>
</html>
Click here to check the live demonstration of the code.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook