How to Send Data With HTTP Post in Angularjs
-
Post Request to API With Response Type
<any>
in Angular - Post Request With Expected Response in Angular
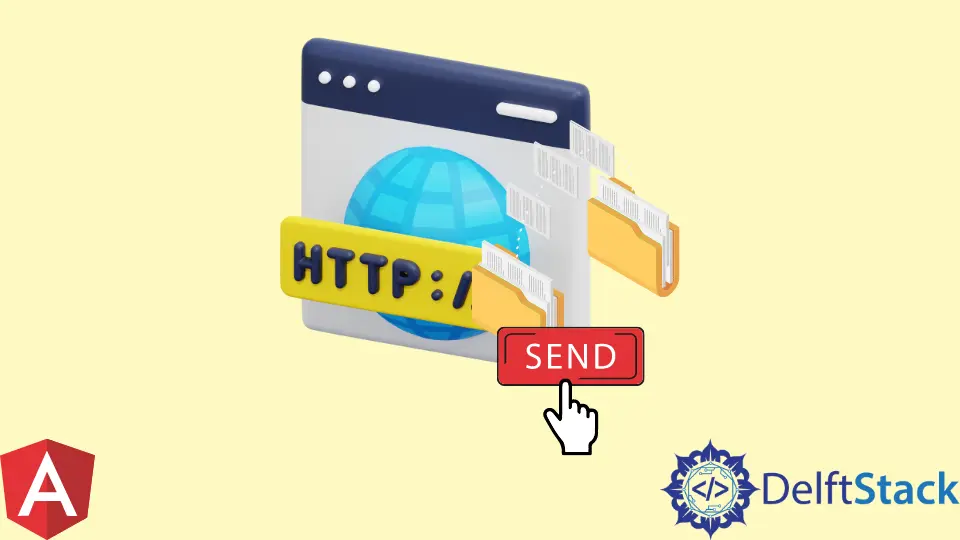
Before we dive into the real point of this topic, let us first learn what http.post()
does in Angular. When you think of Google, the search results that we receive when we search are sent to the server using the post
method, and when a user initiates a search on Google, the get
method is called.
So the http.post()
is used in Angular to store data on the server. The server that will stop this data will have a URL.
When a coder comes across $http.post()
for the first time, especially someone with jQuery knowledge, the first instinct is to replace $jQuery.post()
with $http.post()
, but this won’t work because Angular transmit data differently.
When the $http.post()
function is not applied correctly, the URL data that will be sent is called, but no data is sent. Let’s go ahead and see different ways to apply the HTTP Post function properly.
Post Request to API With Response Type <any>
in Angular
The first example will require us to request a URL, which returns the id assigned to Jason
. The response type is <any>
, so it handles any property sent back as a response.
We have a very simple html
to set up.
<div>
<h1>Angularjs HTTP POST</h1>
<div>Post Id: {{ postId }}</div>
</div>
We then move to the app.component.ts
where we will import HttpClient
, which sends HTTP requests to Angular. We then use the same HttpClient
as a constructor.
import { Component, OnInit } from '@angular/core';
import { HttpClient } from '@angular/common/http';
@Component({ selector: 'app-root', templateUrl: 'app.component.html' })
export class AppComponent implements OnInit {
postId;
constructor(private http: HttpClient) {}
ngOnInit() {
this.http
.post<any>('https://reqres.in/api/users', {
name: 'Jason',
})
.subscribe((data) => {
this.postId = data.id;
});
}
}
Then finally we head on to the app.module.ts
. Here we will import HttpClientModule
into angular:
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { HttpClientModule } from '@angular/common/http';
import { AppComponent } from './app.component';
@NgModule({
imports: [
BrowserModule,
HttpClientModule
],
declarations: [
AppComponent
],
bootstrap: [AppComponent]
})
export class AppModule { }
If all goes as instructed, you should see Post id, with three numbers generated.
Post Request With Expected Response in Angular
This method gives users better control over the response we get from the URL because we can assign the data we want to get. We will call the interface Article
function for this task.
We only make slight changes to the app.component.ts
where we replace the <any>
with <Article>
and create the Article
function right below it.
import { Component, OnInit } from '@angular/core';
import { HttpClient } from '@angular/common/http';
@Component({ selector: 'app-root', templateUrl: 'app.component.html' })
export class AppComponent implements OnInit {
postId;
constructor(private http: HttpClient) {}
ngOnInit() {
this.http
.post<Article>('https://reqres.in/api/users', {
name: 'Jason',
})
.subscribe((data) => {
this.postId = data.name;
});
}
}
interface Article {
id: number;
name: string;
}
Instead of HTTP returning the id as a response, the name assigned to the id is what we get.
Fisayo is a tech expert and enthusiast who loves to solve problems, seek new challenges and aim to spread the knowledge of what she has learned across the globe.
LinkedIn